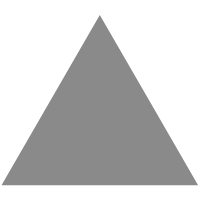
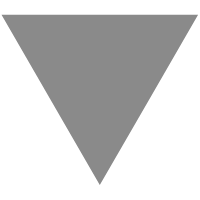
Bad or Good? Behavior Driven Development within Scrum.
source link: https://dzone.com/articles/bad-or-good-behavior-driven
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
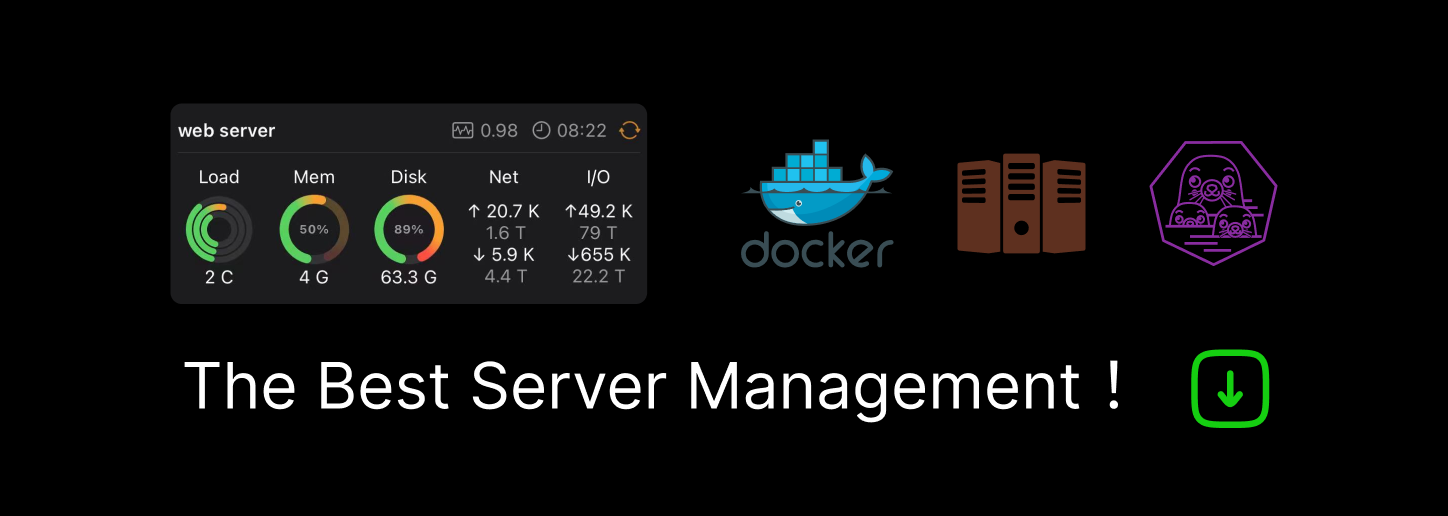
Bad or Good? Behavior Driven Development within Scrum.
I wanted to explore the possibility of using JBehave to formalize scrum's definition of done. The idea being to encapsulate a definition of done as a JBehave scenario. So in true scrum style I decided to timebox 4 hours of work dedicated to JBehave.
From a scrum point of view BDD can be used to turn the definition of done into a test artifact. The team produces scenarios for each task. With JBehave a scenario file describes the required behavior and test steps it will need to pass to be considered done. I.e Given some prerequisites, perform some action and expect some results. See the JBehave project for more detail as this is only a simple example.
Why Examine This Topic?
It is useful to examine anything that a programmer may use in the course of doing their work. Not only should we challenge the assumptions that are floating around out there about different programs, but it may also be useful to look at the resources that are available to us and ask ourselves if we are truly using them in the most effective ways possible.
There is always the chance that just taking a hard look at some of the elements of the work that we do may help us understand where it can be improved upon when the time comes. Indeed, many people find the concept of examining such things quite appealing, and that leads to major research into these topics.
There are a lot of people making incredible strides towards getting things done that the world never believed was possible, but those changes are happening rapidly, and we should all be thankful that this is the case.
BDD in 4 hours?
So scenarios are just text files describing the required functionality in terms of expected behavior. Even before development though, it's possible to run them and see the pending expectations. Let's wizz through a simple example showing who in a scrum team is responsible for what. I already have a project that I use to explore test patterns so I thought I would just introduce it to that. If you want the working version just take a fork from GitHub.
Nb. I have a data fixture used in testing a complex page component. So whilst not a pure scenario I can make its existence part of the Givens. In this project, it's likely that no real given is required, but a developer would add it during the build.
First the product owner and tester produce a simple scenario file describing behavior and expectations.
Given a data fixture When a page is created with 20 items on a page Then expected lines on a page is 4 with data items in a line 5
Next developers write a harness and flesh out the steps required to meet the behavior.
We need to bootstrap the scenario and add steps to it. The general idea is to have a scenario test, that then includes the steps. This is one way of bootstrapping in the steps, other ways are available including a spring system that uses SpringStepsFactory. I ran into problems with the spring system as my project uses spring 3 and JBehave is still locked into spring 2.5.6. In addition, I am using junit 4.8 and spring 2.5.6 requires 4.4. This is ultimately an issue as on many projects. I would not want to tie in those older versions. In fact, other ideas in my project prevent me from doing this too. So in my timebox, I avoid the issue and bootstrap manually.
The Bootstrap test class
public class SimplePageScenario extends JUnitScenario { public SimplePageScenario() { super(new MostUsefulConfiguration() { @Override public ScenarioDefiner forDefiningScenarios() { return new ClasspathScenarioDefiner( new UnderscoredCamelCaseResolver(".scn"), new PatternScenarioParser()); } }); addSteps(new SimplePageSteps()); } }
Before adding the call to addSteps()) running the scenario shows the steps that are pending.
(org/testpatterns/scenarios/example_scenario.scn) Scenario: Given a spring data fixture (PENDING) When a page is created with 20 items on a page (PENDING) Then expected lines on a page is 4 with data items in a line 5 (PENDING)
Finally the developers fill in the Steps class adding sections that match against the scenario keywords, Given, When and Then. Development is done until the behavior passes the test.
public class SimplePageSteps extends Steps { private SimplePage page; private final PageDataFixture dataFixture = new PageDataFixture(); @Given("a data fixture") public void givenASpringDataFixture() { notNull(dataFixture); } @When("a page is created with $itemsOnAPage items on a page") public void createPage(int itemsOnAPage) { page = SimplePage.newInstance(dataFixture.getDataItem(), itemsOnAPage); } @Then("expected lines on a page is $linesOnAPage with data items in a line $itemsInALine") public void validatePage(int linesOnAPage, int itemsInALine) { Map expectedLines = dataFixture.createPageExpectation(linesOnAPage, itemsInALine); Map actualMap = page.getMap(); ensureThat(actualMap, is(expectedLines)); } }
Good Points
I like the potential to get key scrum members interacting. The collaboration between the testers and the product owner is an important one as it should flesh out questions about behavior at an early stage. Similar benefits occur when the development team starts to get to grip on the behavior.
Having a simple scenario artifact means the product owner can get involved with something that actually becomes part of the continuous integration.
Bad Points
The bootstrap is clumsy. Having to add steps means that after producing the new scenarios development has to get involved before the pending steps appear. There are other ways to bootstrap. The spring system is a step in the right direction, in fact I would like to use injection throughout the application. This still does not solve the problem of having to edit something before the steps appear. I would prefer that once the scenario is in place, steps are automatically found as they are written, and appear as not started until they are cut.
Extending the Junit Testcase is also a problem. It's highly likely that in more complex projects we would need mocking techniques or spring-based tests. These have their own runners brought in with the @RunWith annotation. It would be better if there were some way to bootstrap the behavior into any other test framework.
In my 4-hour timebox, I did not get the reporting working. Unfortunately, the reporting requires more coding, and setup and is far from being just a bit of maven configuration.
When running from maven, things didn't work until I configured the maven-resource-plugin to copy over the scenario files. This should have just worked out of the box.
Most of my 4 hours were taken up with configuration which was compounded by the slim documentation.
Conclusion
Attempting a behavior-driven approach using scenarios is worth doing. I could see the benefits. Not sure if JBehave is the solution for me though. Difficult to configure, and incompatibilities with other frameworks would put me off at the moment. I will find some time to have a look at easyb another Java BDD system next.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK