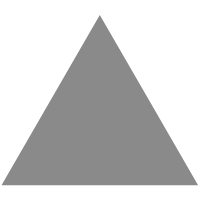
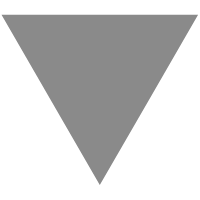
React报错之Cannot assign to 'current' because it is a read-only property - chuck...
source link: https://www.cnblogs.com/chuckQu/p/16578306.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
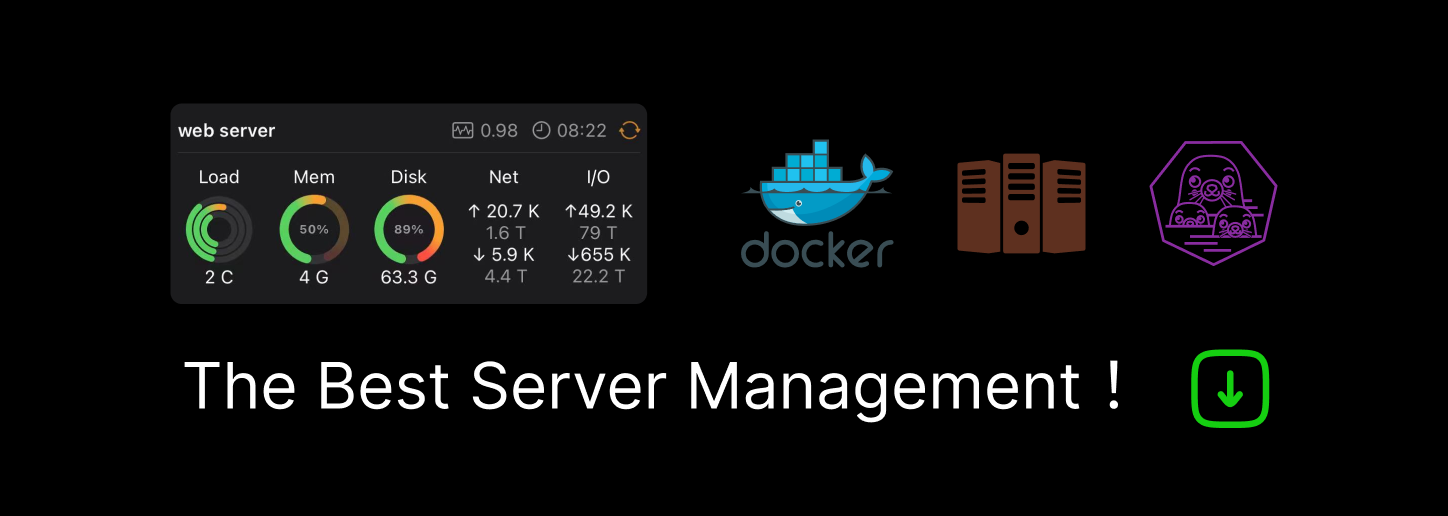
React报错之Cannot assign to 'current' because it is a read-only property
正文从这开始~
当我们用一个null
值初始化一个ref
,但在其类型中不包括null
时,就会发生"Cannot assign to 'current' because it is a read-only property"错误。为了解决该错误,请在ref
的类型中包含null
。比如说,const ref = useRef<string | null>(null)
。
这里有个例子来展示错误是如何发生的。
// App.tsx
import {useEffect, useRef} from 'react';
const App = () => {
const ref = useRef<string>(null);
useEffect(() => {
// ⛔️ Error: Cannot assign to 'current' because it is a read-only property.ts(2540)
ref.current = 'hello';
}, []);
return (
<div>
<h2>hello world</h2>
</div>
);
};
export default App;
上面例子的问题在于,当我们将null
作为初始值传递到useRef
钩子中时,并且我们传递给钩子的泛型中没有包括null
类型,我们创建了一个不可变的ref
对象。
正确的泛型
为了解决该错误,我们必须在传递给钩子的泛型中包括null
类型。
// App.tsx
import {useEffect, useRef} from 'react';
const App = () => {
// 👇️ include null in the ref's type
const ref = useRef<string | null>(null);
useEffect(() => {
ref.current = 'hello';
}, []);
return (
<div>
<h2>hello world</h2>
</div>
);
};
export default App;
在ref
的类型中,我们使用联合类型来包括null
类型,这使它成为可变ref
对象。
现在这个例子中
ref
的类型是字符串或null
,它的current
属性可以赋值为这两种类型中的任意一种的值。
DOM元素
如果你的引用指向一个DOM元素,情况也是一样的。如果你需要改变ref
的current
属性的值,你必须将钩子的类型定为 const ref = useRef<HTMLElement | null>(null)
。
注意,如果你不直接赋值给它的current
属性,你不必在 ref
的类型中包含 null
。
// App.tsx
import {useEffect, useRef} from 'react';
const App = () => {
const ref = useRef<HTMLInputElement>(null);
useEffect(() => {
ref.current?.focus();
}, []);
return (
<div>
<input ref={ref} type="text" defaultValue="" />
</div>
);
};
export default App;
上述例子中的ref
是用来聚焦input
元素的。因为没有对其.current
属性进行赋值,所以没有必要在其类型中包含null
。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK