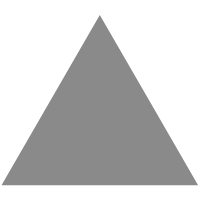
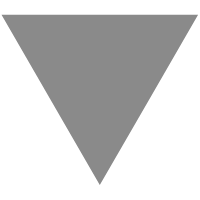
设计模式之单例和原型_程序员田同学的技术博客_51CTO博客
source link: https://blog.51cto.com/u_15476035/5526641
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
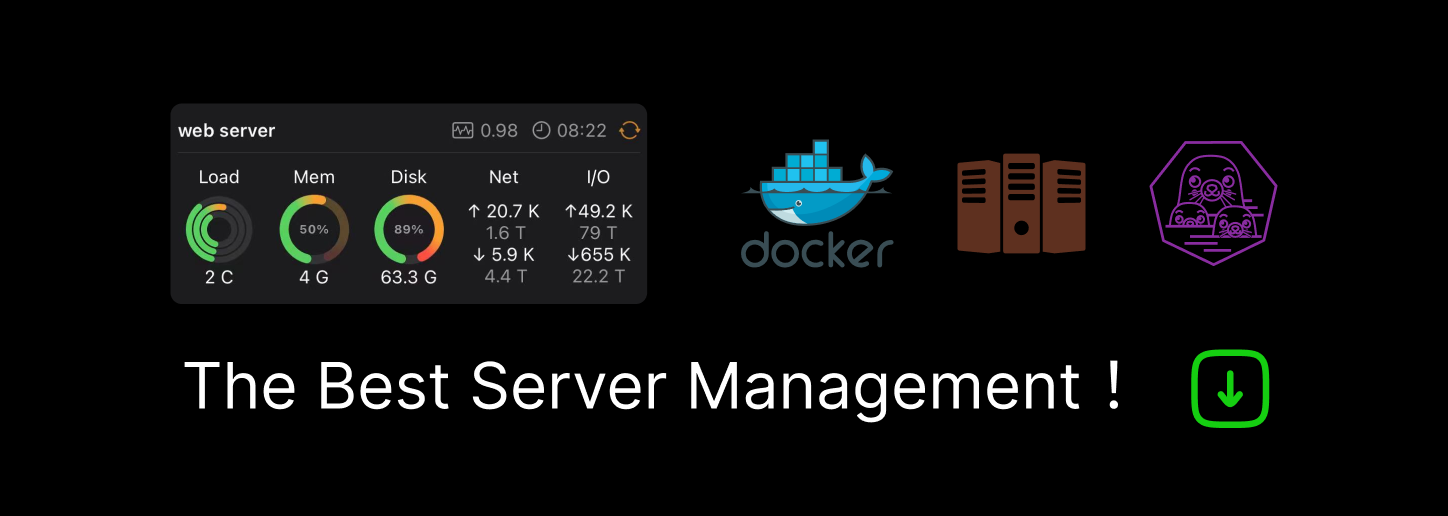
今天这篇文章我们来学习创建型设计模式的另外两个孪生兄弟,单例和原型,其中原型设计模式中我们深入到JVM的内存模型,最后顺便谈谈Java中的值传递和引用传递。
上篇文章 老王买产品 我们从最原始的基本实现方法,到简单(静态)工厂,然后使用工厂方法设计模式进行改造,最后考虑产品会产生变体,我们又扩展到了抽象工厂。
设计模式所有的相关代码均已上传到 码云 读者可以自行下载学习测试。
一、引出问题
今天老王又来了,还是想买我们的产品,今天老王上老就提出来一个要求,当他购买产品的时候,每次都要从货架上给他拿相同的一个。
如果用传统实现方式,当老王拿到产品以后,直接和上一个比对一下就行了,如果不一致老王就还回来。
但通过我们查阅软件的 七大设计原则 ,这很明显违反了依赖倒置原则,为了避免耦合和让代码更易于维护,老王是不能依赖具体产品的。
我们就需要将产品比对在创建产品的时候进行判断,老王就只管拿。
老王来之前应该还有两种情况,一种就是老王还没来,产品就准备好了,也即饿汉式。第二种就是老王什么时候来,什么时候给他准备产品,也即懒汉式。
我们看具体的实现代码:
/**
* 懒汉式
* @author tcy
* @Date 29-07-2022
*/
public class LazySingletonProduct {
private static volatile LazySingletonProduct instance=null;
private LazySingletonProduct(){}
public static synchronized LazySingletonProduct getInstance(){
if (instance==null){
instance=new LazySingletonProduct();
}
return instance;
}
/**
* 饿汉式
* @author tcy
* @Date 29-07-2022
*/
public class HungrySingletonProduct {
private static volatile HungrySingletonProduct instance=new HungrySingletonProduct();
private HungrySingletonProduct(){};
public static synchronized HungrySingletonProduct getInstance(){
if (instance==null){
instance=new HungrySingletonProduct();
}
return instance;
}
}
/**
* @author tcy
* @Date 29-07-2022
*/
public class Client {
public static void main(String[] args) {
HungrySingletonProduct instance1 = HungrySingletonProduct.getInstance();
HungrySingletonProduct instance2 = HungrySingletonProduct.getInstance();
if (instance1==instance2){
System.out.println("我俩一样...");
}else {
System.out.println("我俩不一样...");
}
}
}
以上就是单例设计模式中的懒汉式和饿汉式,应该是设计模式中最简单的一个,理解起来难度也不大。
为了克服老王和他儿子小王一起来拿错的尴尬,我们在方法上加synchronized锁,对象引用上加volatile共享变量,但这样会带来效率问题,如果不考虑多线程需求,读者可自行去掉。
老王今天很明显是找茬,他继续说,如果我不想要一个了,我要每次买都要不同的,你看着办。
每次创建产品都要不同的,传统的方式肯定就是重新new一个对象,但每创建一个对象都是一个复杂的过程,而且这样还会带来一定的代码冗余。
这就需要用到创建型设计模式中的原型模式中的拷贝,其中又分为浅拷贝和深拷贝。
我们先看基本概念。
- 浅克隆:创建一个新对象,对象种属性和原来对象的属性完全相同,对于非基本类型属性仍指向原有属性所指向的内存地址
- 深克隆:创建一个新对象,属性中引用类型也会被克隆,不再指向原来属性所指向的内存地址
这段意思也就是,老王购买产品的时候,如果产品都是基本数据类型(byte(位)、short(短整数)、int(整数)、long(长整数)、float(单精度)、double(双精度)、char(字符)和boolean(布尔值))和String,那么我们就使用浅拷贝。
如果产品包括别的产品(对象)的引用类型就要使用深拷贝。
如果想搞明白,为什么造成深拷贝和浅拷贝这个问题,我们就要重点说说JVM的内存模型。
我们声明一个基本数据类型的变量a=2,实际上是在栈中直接存储了一个a=2,当拷贝的时候直接把值拷贝过去,也就是直接有了一份a的副本。
当我们创建一个对象时Student stu=new Student(),实际上对象的值存储在堆中,在栈中只存放了stu=“对象地址”,stu指向了堆中的地址,jvm拷贝的时候只复制了栈中的地址,实际上他们堆中的对象还是一个。
我们再来看String类型。String 存在于堆内存、常量池;这种比较特殊, 传递是引用地址;由本身的final性, 每次赋值都是一个新的引用地址,原对象的引用和副本的引用互不影响。因此String就和基本数据类型一样,表现出了"深拷贝"特性。
我们具体看实现代码:
浅拷贝类:
/**
* @author tcy
* @Date 29-07-2022
*/
public class ShallowProduct implements Cloneable{
private String name;
private int num;
public void show(){
System.out.println("这是浅产品..."+name+"数量:"+num);
}
public String getName() {
return name;
}
public ShallowProduct setName(String name) {
this.name = name;
return this;
}
public int getNum() {
return num;
}
public ShallowProduct setNum(int num) {
this.num = num;
return this;
}
@Override
public ShallowProduct clone() throws CloneNotSupportedException {
return (ShallowProduct) super.clone();
}
}
如果需要哪个对象浅拷贝,需要该对象实现Cloneable接口,并重写clone()方法。
public void shallowTest()throws CloneNotSupportedException{
ShallowProduct product1=new ShallowProduct();
ShallowProduct product2 = product1.clone();
product1.setName("老王");
product2.setName("老李");
product1.setNum(1);
product2.setNum(2);
product1.show();
product2.show();
}
调用时输出的对象中的值直接就是两个不同的对象,实现了对象的浅拷贝。
如果该对象中包括引用类型呢?那怎么实现呢。
其实原理上也是很简单的,只需要将非基本数据类型也像浅拷贝那样操做就行了,然后在当前clone()方法中,调用非基本数据类型的clone()方法
深拷贝引用类:
/**
* @author tcy
* @Date 29-07-2022
*/
public class Child implements Cloneable{
private String childName;
public String getChildName() {
return childName;
}
public Child setChildName(String childName) {
this.childName = childName;
return this;
}
@Override
protected Child clone() throws CloneNotSupportedException {
return (Child) super.clone();
}
}
深拷贝类:
/**
* @author tcy
* @Date 29-07-2022
*/
public class DeepProduct implements Cloneable{
private String name;
private Integer num;
private Child child;
public String getName() {
return name;
}
public DeepProduct setName(String name) {
this.name = name;
return this;
}
public Integer getNum() {
return num;
}
public DeepProduct setNum(Integer num) {
this.num = num;
return this;
}
public void show(){
System.out.println("这是深产品..."+name+"数量:"+num+"包括child:"+child.getChildName());
}
@Override
public DeepProduct clone() throws CloneNotSupportedException {
DeepProduct clone = (DeepProduct) super.clone();
clone.child=child.clone();
return clone;
}
public Child getChild() {
return child;
}
public DeepProduct setChild(Child child) {
this.child = child;
return this;
}
}
我们测试一下对象中的值是否发生了改变。
public void deepTest() throws CloneNotSupportedException {
DeepProduct product1=new DeepProduct();
Child child=new Child();
child.setChildName("老王child");
product1.setName("老王");
product1.setNum(1);
product1.setChild(child);
//--------------
DeepProduct product2=product1.clone();
product2.setName("老李");
product2.setNum(2);
product2.getChild().setChildName("老李child");
product1.show();
product2.show();
}
老李、老王都正确的输出了,说明实现没有问题。
这样就符合了老王的要求。
既然说到了jvm的内存模型,就有必要说一下java中的值传递和引用传递。
实际上java应该就是值传递,在调用方法的时候,如果参数是基本数据类型,那么传递的就是副本,我们在方法中无论怎么给他赋值,他原本的值都不会有变化。
在调用方法的时候,如果参数是引用数据类型,那么传递的就是这个对象的地址,我们在方法中修改这个对象都会影响他原本的对象。
造成这个现象的原因其实是和浅拷贝、深拷贝的原理是一样的,都是栈、堆内存的结构导致的。
老王看他的要求都满足了,最后心满意足的拿着产品走了。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK