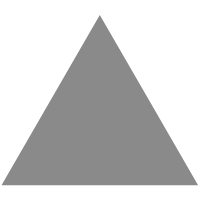
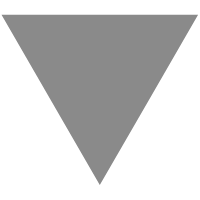
Laravel Livewire CRUD Tutorial
source link: https://www.laravelcode.com/post/laravel-livewire-crud-tutorial
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
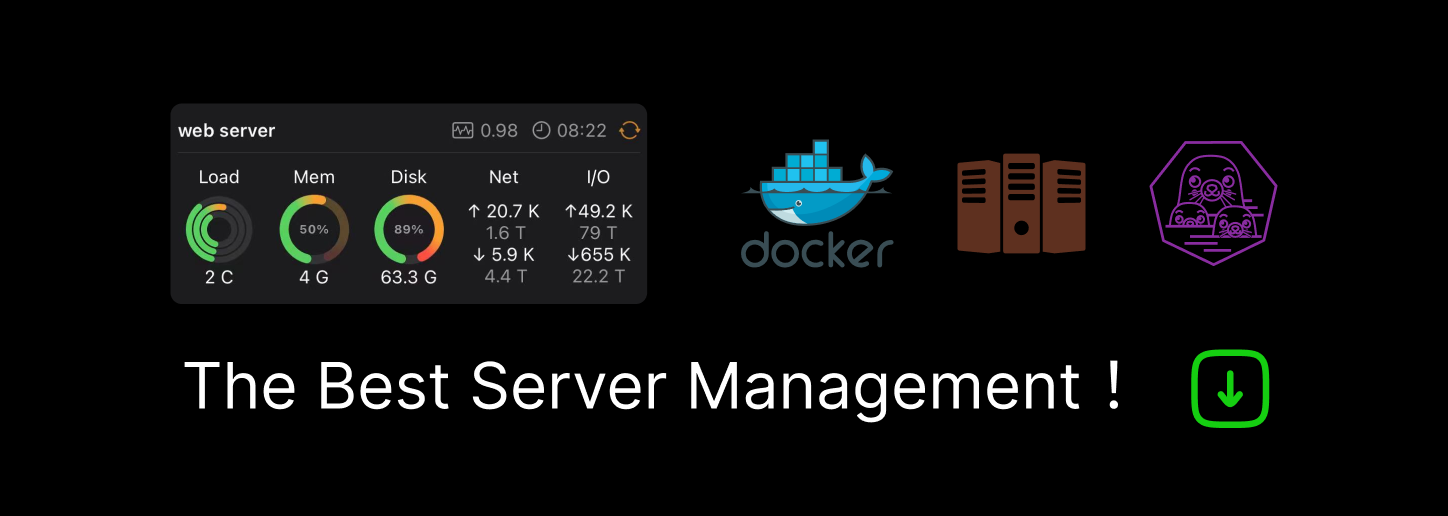
Laravel Livewire CRUD Tutorial
Hello Artisan
Today i am coming with a pristinely incipient tutorial on the topic of laravel livewire. A couple of days ago i engendered a tutorial on the topic of how laravel livewire works. But in this i will show you a depth concept of laravel livewire.
In this pristinely incipient tutorial i will discuss about laravel livewire crud example from scratch. In this laravel livewire examples tutorial you will learn how to engender crud operation in laravel utilizing livewire. In this laravel livewire tutorial you will learn it from step by step.
Livewire is a full-stack framework for Laravel that makes building dynamic interfaces simple, without leaving the comfort of Laravel. It is a frontend framework. After learning it you will just verbalize "Verbally express hello to Livewire".
I will do this livewire crud tutorial utilizing the version of laravel 7. After culminating this project you will visually perceive that it will work like javascript framework vue js or javascript libraary react js. Utilizing laravel livewire you can insert, update or efface data without page refresh. How astounding it is. Isn't it? So let's commence our laravel livewire crud example tutorial.
You can also check the git repository of this project. if you face any error, please have look on it and check you code.
Table of Contents
- Download Laravel App
- Create Routes
- Create Model and Migration
- Install Livewire
- Create Livewire Component and View
- Create Blade File
- Run Project
You can check the project preview also from this below image. Just have a look.
Preview | User List

Preview | User Edit Form

Step 1 : Download Laravel 7
In this laravel livewire crud tutorial i will explain it from step by step. So download the latest laravel app by the following command.
composer create-project --prefer-dist laravel/laravel livewire
Step 2 : Create Routes
Now we need to create our routes. So paste this below code in web.php file.
routes/web.php
Route::view('contacts', 'users.contacts');
Here we used view route and users.contacts indicates blade view directories. So make it like below image.
Now paste this following code to contacts.blade.php.
resources/views/users/contacts.blade.php
@extends('layouts.app')
@section('content')
<div class="flex justify-center">
@livewire('contact')
</div>
@endsection
Step 3 : Create Model and Migration
Now we have to create our model and migration to submit form data in database so that we can check laravel livewire example crud tutorial. So make it by below command.
php artisan make:model Contact -m
now open Contact model and paste below code.
app/Contact.php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Contact extends Model
{
protected $guarded = [];
}
now add this two field to your contacts table and run migrate command to save it database.
database/migrations/create_contacts_table.php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateContactsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('contacts', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('contacts');
}
}
and then run
php artisan migrate
Step 4 : Install Livewire
To setup livewire in your project, run below command
composer require calebporzio/livewire
After running this command open your app.blade.php and paste this below code.
resources/views/layouts/app.blade.php
<!doctype html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="csrf-token" content="{{ csrf_token() }}">
<title>{{ config('app.name', 'Laravel') }}</title>
<script src="{{ asset('js/app.js') }}" defer></script>
<link href="{{ asset('css/app.css') }}" rel="stylesheet">
@livewireStyles
</head>
<body>
<div id="app">
<nav class="navbar navbar-expand-md navbar-light bg-white shadow-sm">
<div class="container">
<a class="navbar-brand" href="{{ url('/') }}">
{{ config('app.name', 'Laravel') }}
</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="{{ __('Toggle navigation') }}">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav mr-auto">
</ul>
<ul class="navbar-nav ml-auto">
@guest
<li class="nav-item">
<a class="nav-link" href="{{ route('login') }}">{{ __('Login') }}</a>
</li>
@if (Route::has('register'))
<li class="nav-item">
<a class="nav-link" href="{{ route('register') }}">{{ __('Register') }}</a>
</li>
@endif
@else
<li class="nav-item dropdown">
<a id="navbarDropdown" class="nav-link dropdown-toggle" href="#" role="button" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false" v-pre>
{{ Auth::user()->name }} <span class="caret"></span>
</a>
<div class="dropdown-menu dropdown-menu-right" aria-labelledby="navbarDropdown">
<a class="dropdown-item" href="{{ route('logout') }}"
onclick="event.preventDefault();
document.getElementById('logout-form').submit();">
{{ __('Logout') }}
</a>
<form id="logout-form" action="{{ route('logout') }}" method="POST" style="display: none;">
@csrf
</form>
</div>
</li>
@endguest
</ul>
</div>
</div>
</nav>
<main class="py-4">
@yield('content')
</main>
</div>
@livewireScripts
</body>
</html>
Step 5 : Create Livewire Component and View
Run the below command to create livewire view and component.
php artisan make:livewire contact
After running this command you will find two file in the following path app/Http/Livewire/Contact.php and resources/views/livewire/contact.blade.php
app/Http/Livewire/Contact.php
namespace App\Http\Livewire;
use App\Contact;
use Livewire\Component;
class Contact extends Component
{
public $data, $name, $email, $selected_id;
public $updateMode = false;
public function render()
{
$this->data = Contact::all();
return view('livewire.contact');
}
private function resetInput()
{
$this->name = null;
$this->email = null;
}
public function store()
{
$this->validate([
'name' => 'required|min:5',
'email' => 'required|email:rfc,dns'
]);
Contact::create([
'name' => $this->name,
'email' => $this->email
]);
$this->resetInput();
}
public function edit($id)
{
$record = Contact::findOrFail($id);
$this->selected_id = $id;
$this->name = $record->name;
$this->email = $record->email;
$this->updateMode = true;
}
public function update()
{
$this->validate([
'selected_id' => 'required|numeric',
'name' => 'required|min:5',
'email' => 'required|email:rfc,dns'
]);
if ($this->selected_id) {
$record = Contact::find($this->selected_id);
$record->update([
'name' => $this->name,
'email' => $this->email
]);
$this->resetInput();
$this->updateMode = false;
}
}
public function destroy($id)
{
if ($id) {
$record = Contact::where('id', $id);
$record->delete();
}
}
}
Step 6 : Create Blade File
Now create a folder structure like below image. We are in the last past of our livewire tutorial.
Now paste this below code to you blade file. Here we will create contact, create and update file to create our laravel livewire crud example.
resources/views/livewire/contact.blade.php
<div>
<div class="col-md-6">
@if (count($errors) > 0)
<div class="alert alert-danger">
<a href="#" class="close" data-dismiss="alert">×</a>
<strong>Sorry!</strong> invalid input.<br><br>
<ul style="list-style-type:none;">
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
@if($updateMode)
@include('livewire.update')
@else
@include('livewire.create')
@endif
<table class="table table-striped" style="margin-top:20px;">
<tr>
<td>NO</td>
<td>NAME</td>
<td>EMAIL</td>
<td>ACTION</td>
</tr>
@foreach($data as $row)
<tr>
<td>{{$loop->index + 1}}</td>
<td>{{$row->name}}</td>
<td>{{$row->email}}</td>
<td>
<button wire:click="edit({{$row->id}})" class="btn btn-sm btn-outline-danger py-0">Edit</button> |
<button wire:click="destroy({{$row->id}})" class="btn btn-sm btn-outline-danger py-0">Delete</button>
</td>
</tr>
@endforeach
</table>
</div>
</div>
resources/views/livewire/create.blade.php
<div>
<div class="form-group">
<label for="exampleInputPassword1">Enter Name</label>
<input type="text" wire:model="name" class="form-control input-sm" placeholder="Name">
</div>
<div class="form-group">
<label>Enter Email</label>
<input type="email" class="form-control input-sm" placeholder="Enter email" wire:model="email">
</div>
<button wire:click="store()" class="btn btn-primary">Submit</button>
</div>
resources/views/livewire/update.blade.php
<div>
<input type="hidden" wire:model="selected_id">
<div class="form-group">
<label for="exampleInputPassword1">Enter Name</label>
<input type="text" wire:model="name" class="form-control input-sm" placeholder="Name">
</div>
<div class="form-group">
<label>Enter Email</label>
<input type="email" class="form-control input-sm" placeholder="Enter email" wire:model="email">
</div>
<button wire:click="update()" class="btn btn-primary">Update</button>
</div>
Now all are set to go. Just visit this below url to test our laravel livewire crud example project.
http://127.0.0.1:8000/contacts
Now if you checked it then i think you must say that if vue and blade had a baby it would be a jellyfish. Isn't it? Hope it can help you.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK