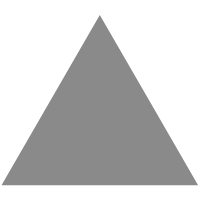
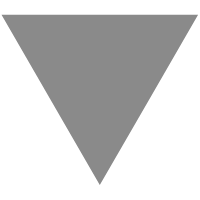
Share Data Between Child and Parent Components in Angular 12 Part 2
source link: https://www.laravelcode.com/post/share-data-between-child-and-parent-components-in-angular-12-part-2
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
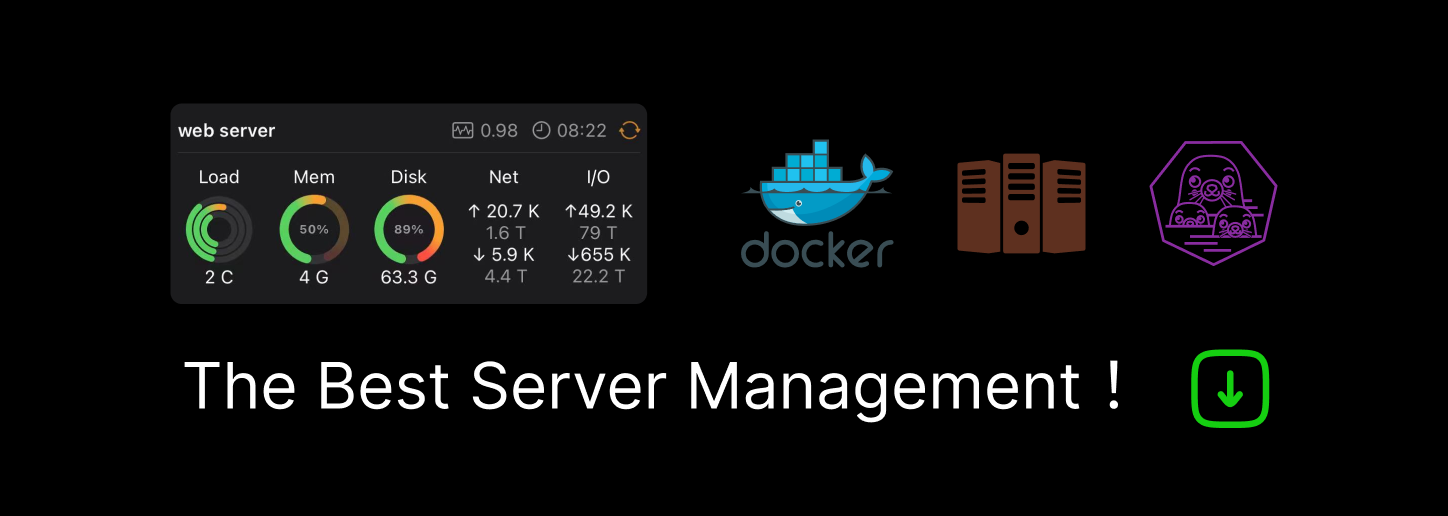
Share Data Between Child and Parent Components in Angular 12 Part 2
In the previous article, we have created parent component and set dynamic data view in modal.
In this article, we will create child component and move modal view to it. So let's continue from creating a child component.
Step 1: Create child component.
You may have already running application in Terminal, so open new Terminal and run the following command in it.
ng generate component child
This will create new component folder. Now remove modal HTML code from parent view and move it to child view. Change property name from selectedUser to user.
Here is child.component.ts file.
<div class="modal fade" id="modal" tabindex="-1" aria-labelledby="modalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="modalLabel">Modal title</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<div *ngIf="user">
<p>{{ user.email }}</p>
<p>{{ user.name }}</p>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
Step 2: Parent view
And in the parent.component.ts file, instead of modal, add the tag for child selector tag. Here is the file after changes. You will also need to pass the selectedUser
property to child component.
<table class="table table-hover">
<thead>
<tr>
<th scope="col">ID</th>
<th scope="col">Name</th>
<th scope="col">Email</th>
<th scope="col">Aciton</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let user of users">
<td>{{ user.id }}</td>
<td>{{ user.name }}</td>
<td>{{ user.email }}</td>
<td>
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#modal" (click)="onClick(user)">Show</button>
</td>
</tr>
</tbody>
</table>
<app-child [user]="selectedUser"></app-child>

Step 3: @Input decorator in child component
Now we are passing data from parent component to child component, so we need to add @Input
decorator in ChildComponent
class. Don't forget to import Input from angular/core module.
import { Component, Input, OnInit } from '@angular/core';
@Component({
selector: 'app-child',
templateUrl: './child.component.html',
styleUrls: ['./child.component.css']
})
export class ChildComponent implements OnInit {
@Input() user? : any;
constructor() { }
ngOnInit(): void {
}
}
Step 4: Input fields in child view
Now in the child view, we will put input field instead of text so we can change data that will push to parent component. In the child.component.html, add input fields instead of text. Here is the final view from child component.
<div class="modal fade" id="modal" tabindex="-1" aria-labelledby="modalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="modalLabel">Modal title</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<div *ngIf="user">
<div class="mb-3 row">
<label for="name" class="col-sm-2 col-form-label">Name</label>
<div class="col-sm-10">
<input [(ngModel)]="user.name" class="form-control" id="name">
</div>
</div>
<div class="mb-3 row">
<label for="email" class="col-sm-2 col-form-label">Email</label>
<div class="col-sm-10">
<input [(ngModel)]="user.email" class="form-control" id="email">
</div>
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
ngModel will bind two way, so when we change name or email to any user, it will also reflect into parent component also.
Step 5: import FormsModule class
We will two-way bind input fields, so add square brackets and round brackets to input fields. We have added form fields into the application so we also required to import FormsModule from angular/forms module in app.module.ts file.
import { FormsModule } from '@angular/forms';
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { ParentComponent } from './parent/parent.component';
import { ChildComponent } from './child/child.component';
@NgModule({
declarations: [
AppComponent,
ParentComponent,
ChildComponent
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Now everything is done. Open any user data and try to change name or email. This will also change data in table also.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK