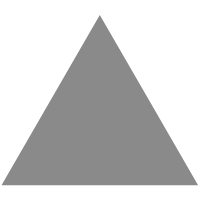
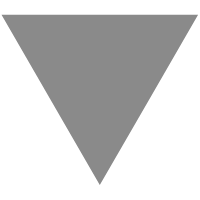
JavaScript shorthand tips and tricks
source link: https://dev.to/amitavmishra99/javascript-shorthand-tips-and-tricks-4i7h
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
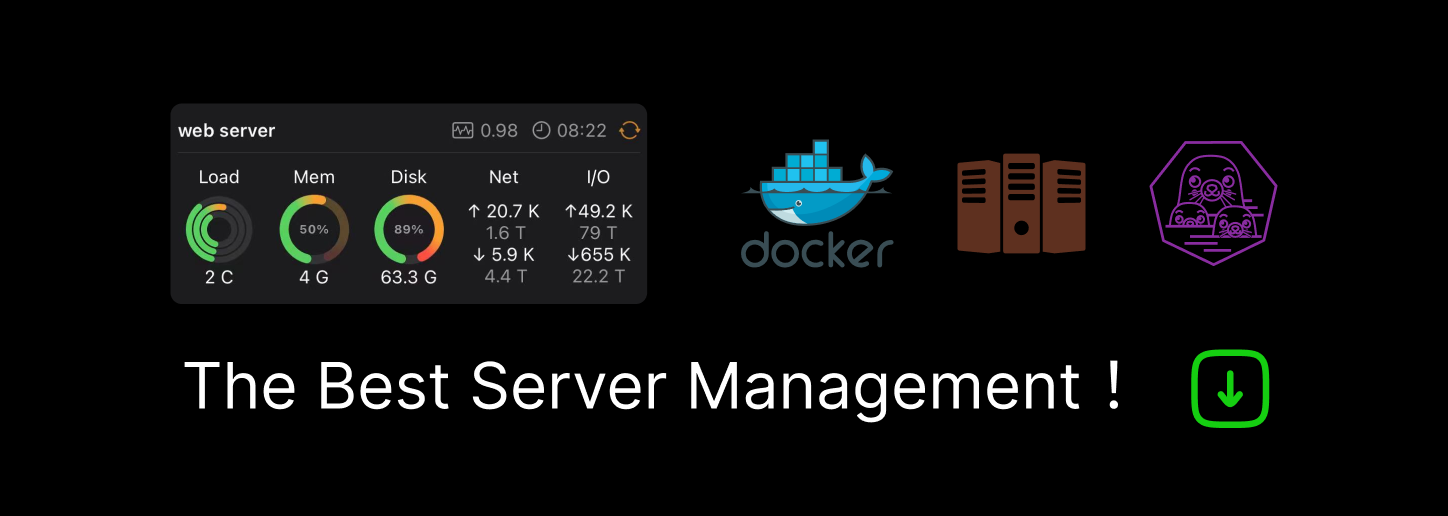
Let's checkout some shorthand tips and other tricks.
Remember, some of these are not readable and might not seems relevant to use in a project, but my intensions here is to make you aware that these tricks exist.
Assigning values to multiple variables
We can assign values to multiple variables in one line with array destructuring.
//Longhand
let a, b, c;
a = 5;
b = 8;
c = 12;
//Shorthand
let [a, b, c] = [5, 8, 12];
Assigning a default value
We can use OR(||)
short circuit evaluation or nullish coalescing operator (??)
to assign a default value to a variable in case the expected value is found falsy.
//Longhand
let imagePath;
let path = getImagePath();
if (path !== null && path !== undefined && path !== '') {
imagePath = path;
} else {
imagePath = 'default.jpg';
}
//Shorthand
let imagePath = getImagePath() || 'default.jpg';
// OR
let imagePath = getImagePath() ?? 'default.jpg';
AND(&&) Short circuit evaluation
If you are calling a function only if a variable is true
, then you can use the AND(&&)
short circuit as an alternative for this.
//Longhand
if (isLoggedin) {
goToHomepage();
}
//Shorthand
isLoggedin && goToHomepage();
Swap two variables
To swap two variables, we often use a third variable. We can swap two variables easily with array destructuring assignment.
let x = 'Hello', y = 55;
//Longhand
const temp = x;
x = y;
y = temp;
//Shorthand
[x, y] = [y, x];
Template Literals
We normally use the +
operator to concatenate string values with variables. With ES6 template literals, we can do it in a more simple way.
//Longhand
console.log('You got a missed call from ' + number + ' at ' + time);
//Shorthand
console.log(`You got a missed call from ${number} at ${time}`);
Multiple condition checking
For multiple value matching, we can put all values in one array and use indexOf()
or includes()
method.
//Longhand
if (value === 1 || value === 'one' || value === 2 || value === 'two') {
// Execute some code
}
// Shorthand
if ([1, 'one', 2, 'two'].indexOf(value) >= 0) {
// Execute some code
}
// OR
if ([1, 'one', 2, 'two'].includes(value)) {
// Execute some code
}
Object Property Assignment
If the variable name and object key name are the same then we can just mention the variable name in object literals instead of both key and value. JavaScript will automatically set the key same as the variable name and assign the value as the variable value.
let firstname = 'Amitav';
let lastname = 'Mishra';
//Longhand
let obj = {firstname: firstname, lastname: lastname};
//Shorthand
let obj = {firstname, lastname};
String into a Number
We can convert string to number by simply providing an unary operator (+)
in front of the string value.
//Longhand
let total = parseInt('453', 10);
let average = parseFloat('42.6', 10);
//Shorthand
let total = +'453';
let average = +'42.6';
Exponent Power
We can use Math.pow()
method to find the power of a number. There is a shorter syntax to do it with a double asterisk (**)
.
//Longhand
const power = Math.pow(4, 3); // 64
// Shorthand
const power = 4**3; // 64
Double NOT bitwise operator (~~)
The double NOT bitwise operator is a substitute for Math.floor()
method.
//Longhand
const floor = Math.floor(6.8); // 6
// Shorthand
const floor = ~~6.8; // 6
The double NOT bitwise operator approach only works for 32 bit integers i.e (2**31)-1 = 2147483647. So for any number higher than 2147483647 and less than 0, bitwise operator
(~~)
will give wrong results, so recommended to useMath.floor()
in such case.
Find max and min number in an array
const arr = [2, 8, 15, 4];
Math.max(...arr); // 15
Math.min(...arr); // 2
Merging of arrays
let arr1 = [20, 30];
//Longhand
let arr2 = arr1.concat([60, 80]); // [20, 30, 60, 80]
//Shorthand
let arr2 = [...arr1, 60, 80]; // [20, 30, 60, 80]
Remove falsey values from Array
let arr = [12, null, 0, 'xyz', null, -25, NaN, '', undefined, 0.5, false];
let filterArray = arr.filter(Boolean);
// filterArray = [12, "xyz", -25, 0.5]
Note: Zero (0) is considered to be a falsey value so it will be removed in both the cases. You can add an extra check for zero to keep it.
Remove duplicates from Array
const arr = [10, 5, 20, 10, 6, 5, 8, 5, 20];
const unique = [...new Set(arr)];
console.log(unique); // [10, 5, 20, 6, 8]
Create random string token
const token = Math.random().toString(36).slice(2);
// it will create a 11 character string. Ex: '3efstaz9g8a'
Find number of arguments expected by a function
function func(a, b, c) {
// code
}
console.log(func.length); // 3
// function with Rest parameter
function func(a, b, ...c) {
// code
}
console.log(func.length); // 2
Check if a key exists in an object
const ob = {x: 5, y: 10};
// using "in" operator
console.log('x' in ob); // true
console.log('abc' in ob); // false
// using Reflect object
console.log(Reflect.has(ob, 'x')); // true
console.log(Reflect.has(ob, 'abc')); // false
You may also like
Thanks for your time ❤️
Find more of my writings on web development blogs at jscurious.com
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK