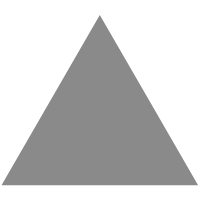
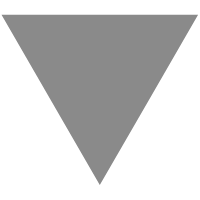
How to Prevent Cross-Site Scripting in Node.js
source link: https://www.makeuseof.com/prevent-cross-site-scripting-in-nodejs/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
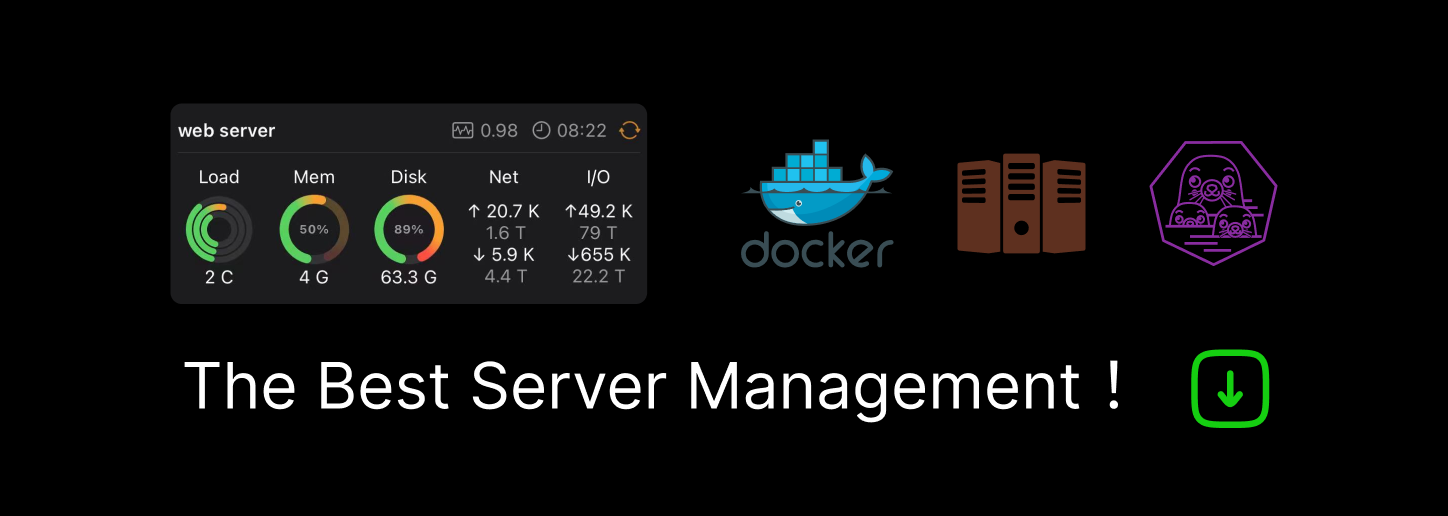
How to Prevent Cross-Site Scripting in Node.js
Attackers might steal sensitive data from your website. Learn how to protect your website from XSS attacks.
Cross-site scripting (XSS) is a type of security exploit that allows attackers to inject malicious scripts on websites using client code. It poses a significant threat as attackers can use it to impersonate users, gain access to sensitive data, or even change the page contents of the website.
It is so dangerous that in 2021, it was number two in the common weakness enumeration list of the top 25 most dangerous weaknesses. This means if you are creating websites, you must know about cross-site scripting and how to prevent it.
How Does Cross-Site Scripting Work?
Before understanding how cross-site scripting works, it is important to know what the same-origin policy (SOP) means. SOP is a security mechanism policy that restricts a website (one origin) from reading or writing to another website (a different origin). It prevents malicious websites from sending malicious code to trusted websites.
Cross-site scripting attacks try to bypass this policy by exploiting the browser’s inability to distinguish between legitimate HTML and malicious code. For example, an attacker can inject JavaScript code into the target website. Suppose the browser executes the code, and the attacker gains access to session tokens, cookies, and other sensitive data.
There are three types of cross-site scripting that hackers use to break websites: reflected, stored, and DOM XSS.
How to Prevent Cross-Site Scripting in Node
The following are some steps you can take to prevent cross-site scripting in Node.
Sanitize Input
Attackers have to be able to send data to your web application and display it to a user in order to perform an XSS attack. Therefore, the first preventative measure you must take is sanitizing all the input your application receives from its users. This is crucial because it detects the bogus data before the server executes it. You could do this manually or use a tool like validator which makes the process faster.
For instance, you can use the validator to escape HTML tags in user input like below.
import validator from "validator";
let userInput = `Jane <script onload="alert('XSS hack');"></script>`;
let sanitizedInput = validator.escape(userInput);
If you were to run the above code, the sanitized output would be this.
Jane <script onload="alert('XSS hack');"></script>
Restrict User Input
Restrict the type of input a user can submit in your form through validation. For instance, if you have an input field for an email, only allow input with the email format. This way, you minimize the chances of attackers submitting bad data. You can also use the validator package for this.
Implement HTTP Only Cookie Policy
Cookies store data in a local cache and send it to back to the server via HTTP. But attackers can also use JavaScript to access them via the browser, so they are easy targets.
The HTTP-only cookie is a policy that prevents client-side scripts from accessing cookie data. This means that even if your application contains a vulnerability and an attacker exploits it, they won’t be able to access the cookie.
Here is an example of how you can implement the HTTP-only cookie policy in Node.js using Express:
app.use(express.session({
secret: "secret",
cookie: {
httpOnly: true,
secure: true
}
}))
If an attacker tried to access the cookie with the httpOnly tag set to true as shown above, they would receive an empty string.
Cross-Site Scripting Is an Easy Target for Hackers
While ensuring the security of your application is crucial, implementing it can get complicated. In this post, you learned about cross-site scripting attacks and how you can prevent them in Node. Since attackers take advantage of vulnerabilities in your application to inject malicious code into your server, always ensure you sanitize user input. By doing this, you remove the malicious code before your application stores it or executes it.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK