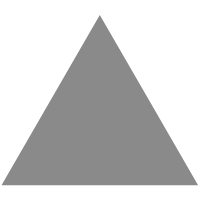
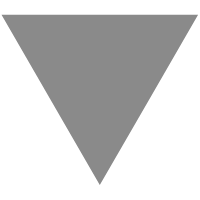
Learning .NET MAUI Part 2
source link: https://jesseliberty.com/2022/06/11/learning-net-maui-part-2/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
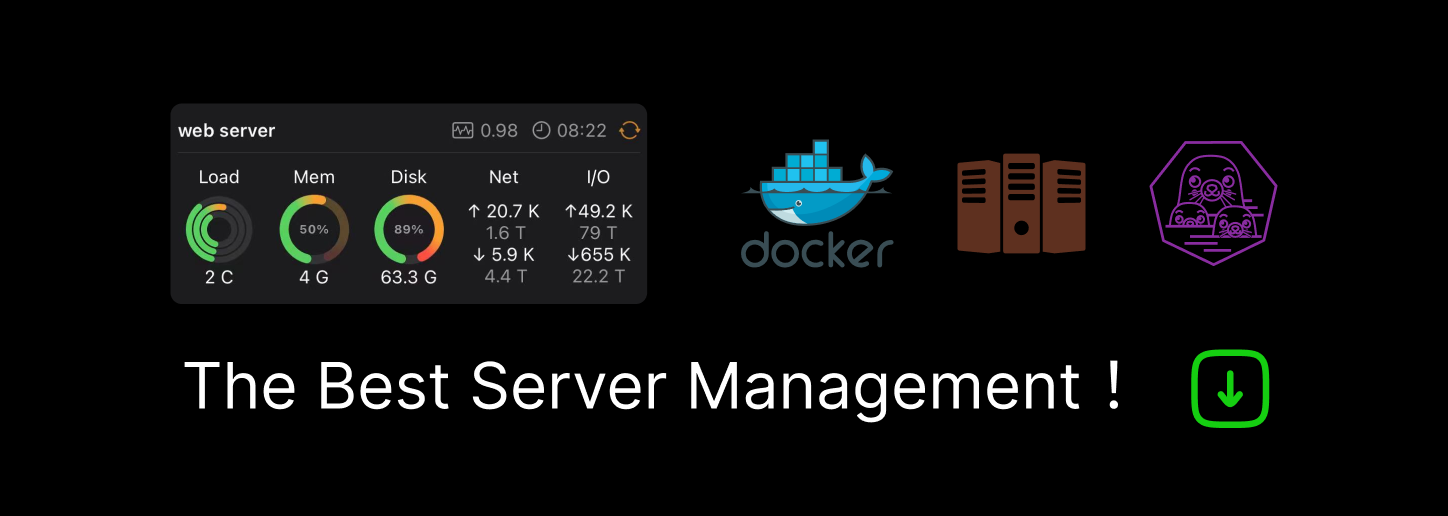
Learning .NET MAUI Part 2
As noted in part 0, I assume you are a Xamarin.Forms/C# programmer, familiar with Visual Studio. This series is not about converting your existing Xamarin.Forms apps; rather it is about converting your brain to MAUI. Since I’m learning as I’m going, your mileage may vary.
Community Toolkit
One of the tools you’ll absolutely want is the .NET Community Toolkit. It is available as an open-source project on GitHub. There are all sorts of goodies in there and you may want to take the time, at least, to read the Read.me file.
Global Usings
C# now offers Global Usings — that is, you mark the using statement once, and it is implicitly available on all your files. To do so create a new file (I called mine GlobalUsings.cs). If you used a class, delete everything in it except the using statements. Next, put the word global before each using (intellicode will help).
global using System;
global using System.Collections.Generic;
global using System.Linq;
global using System.Text;
global using System.Threading.Tasks;
You will not have to enter these using statements in any other file.
First look at ZipWise
We want to talk to a web service and there are a number of free ones to choose from. For this set of blog posts I’ll use ZipWise.
To get started, get yourself an account and an API key. There are a number of endpoints to choose from, and we will eventually build out our app to offer each choice. For now, let’s look at using the zipInfo api.
We’ll be working in json so we’ll use this call:
https://www.zipwise.com/webservices/zipinfo.php?key=YOUR_API_KEY&zip=-1720&format=json
Let’s get some JSON from ZipWise for a city. To do so, go to the ZipWise site, click on [Docs] for Zipinfo, which will bring you to an interactive page where you can put in your zip code and your key. Copy the sample and adjust accordingly.
https://www.zipwise.com/webservices/zipinfo.php?key=YOURKEY&zip=01720&format=json
When I put in my zip code, I get back:
{"results":{"zip":"01720","cities":[{"city":"Acton","preferred":"P"},{"city":"W Acton","preferred":"N"},{"city":"West Acton","preferred":"N"}],"county":"Middlesex","state":"MA","country":"U","area_code":"978","fips":"25017","time_zone":"EST","daylight_savings":"Y","latitude":"42.4836","longitude":"-71.4418","type":"S","population":"22791"}}
Using Json Pretty Print I can get a more readable version:
{
"results": {
"zip": "01720",
"cities": [
{
"city": "Acton",
"preferred": "P"
},
{
"city": "W Acton",
"preferred": "N"
},
{
"city": "West Acton",
"preferred": "N"
}
],
"county": "Middlesex",
"state": "MA",
"country": "U",
"area_code": "978",
"fips": "25017",
"time_zone": "EST",
"daylight_savings": "Y",
"latitude": "42.4836",
"longitude": "-71.4418",
"type": "S",
"population": "22791"
}
}
Now we turn to json2csharp to emit classes for our JSON
public class City
{
public string city { get; set; }
public string preferred { get; set; }
}
public class Results
{
public string zip { get; set; }
public List<City> cities { get; set; }
public string county { get; set; }
public string state { get; set; }
public string country { get; set; }
public string area_code { get; set; }
public string fips { get; set; }
public string time_zone { get; set; }
public string daylight_savings { get; set; }
public string latitude { get; set; }
public string longitude { get; set; }
public string type { get; set; }
public string population { get; set; }
}
public class Root
{
public Results results { get; set; }
}
We'll work with these classes in the next post
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK