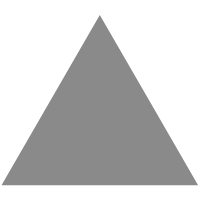
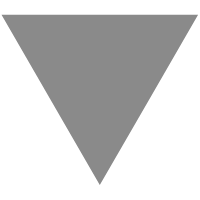
java数据结构之哈希表
source link: https://blog.51cto.com/u_14486541/5369902
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
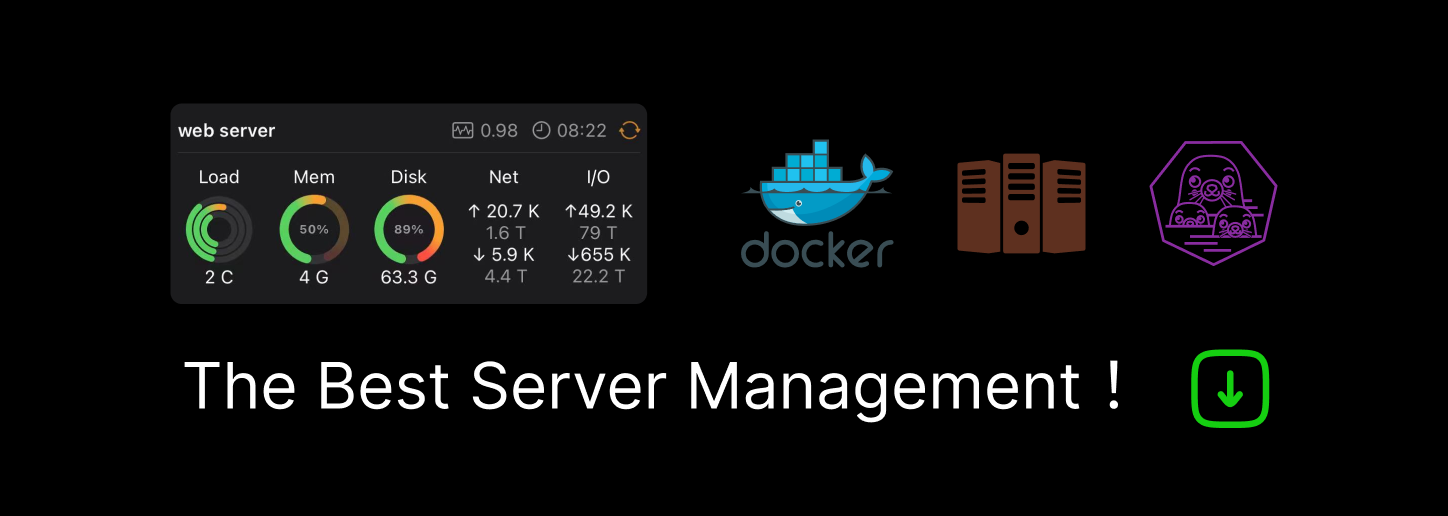
哈希表(散列)-Google 上机题
package cn.ycl.dataStructures.HashTable;
import java.util.Scanner;
//哈希表
public class HashTableDemo{
public static void main(String[] args) {
//创建哈希表
HashTable hashTable = new HashTable(7);
//创建一个简单的菜单
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("add:添加员工");
System.out.println("list:查询员工");
System.out.println("exit: 退出");
String key= scanner.next();
switch (key) {
case "add":
System.out.println("请输入id:");
int id=scanner.nextInt();
System.out.println("请输入name:");
String name= scanner.next();
Emp emp = new Emp(id ,name);
hashTable.add(emp);
break;
case "list":
hashTable.list();
break;
case "exit":
scanner.close();
System.exit(0);
break;
default:
break;
}
}
}
}
//创建HashTabble管理多条链表
class HashTable{
private int size;
private EmpLinkedList [] empLinkdedListArray;
//
public HashTable(int size) {
super();
this.size = size;
empLinkdedListArray=new EmpLinkedList[size];
for (int i = 0; i < size; i++) {
empLinkdedListArray[i]=new EmpLinkedList();
}
}
//添加员工
public void add(Emp emp) {
//根据员工id,判断该员工应该添加到那条链表中去
int num=selectLinkList(emp.id);
//将员工加入到对应的链表中
empLinkdedListArray[num].add(emp);
}
//显示员工
public void list() {
for (int i = 0; i < size; i++) {
empLinkdedListArray[i].list(i);
}
}
// 散列函数,使用简单的取模法
public int selectLinkList(int id) {
// 返回的取余代表将员工插入到哈希表中的哪个链表中
return id % size;
}
}
class Emp {
int id;
String name;
Emp next;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Emp getNext() {
return next;
}
public void setNext(Emp next) {
this.next = next;
}
public Emp() {
super();
}
public Emp(int id, String name) {
super();
this.id = id;
this.name = name;
}
}
class EmpLinkedList {
Emp head;
//添加员工
public void add(Emp emp) {
// 当前链表为空时
if (head == null) {
head= emp;
return;
}
Emp temp=head;
while (true) {
// 持续查找,直到找到链表末尾
if (temp.next==null) {
break;
}
temp=temp.next;
}
temp.next=emp;
}
// 遍历员工
public void list(int no) {
Emp temp = head;
if (head== null) {
System.out.println("第"+(no+1)+"条链表为空");
return;
}
System.out.print("第"+(no+1)+"条链表的信息为:");
while (true) {
System.out.printf("=>id=%d, name=%s\t", temp.id, temp.name);
System.out.println();
if (temp.next == null) {
break;
}
temp = temp.next;// 后移,遍历
}
}
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK