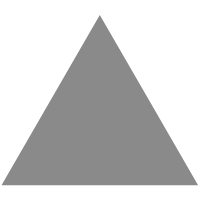
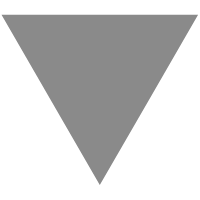
Angular 14 – Introducing Standalone Components – Dhananjay Kumar
source link: https://debugmode.net/2022/06/07/angular-14-introducing-standalone-components%ef%bf%bc/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
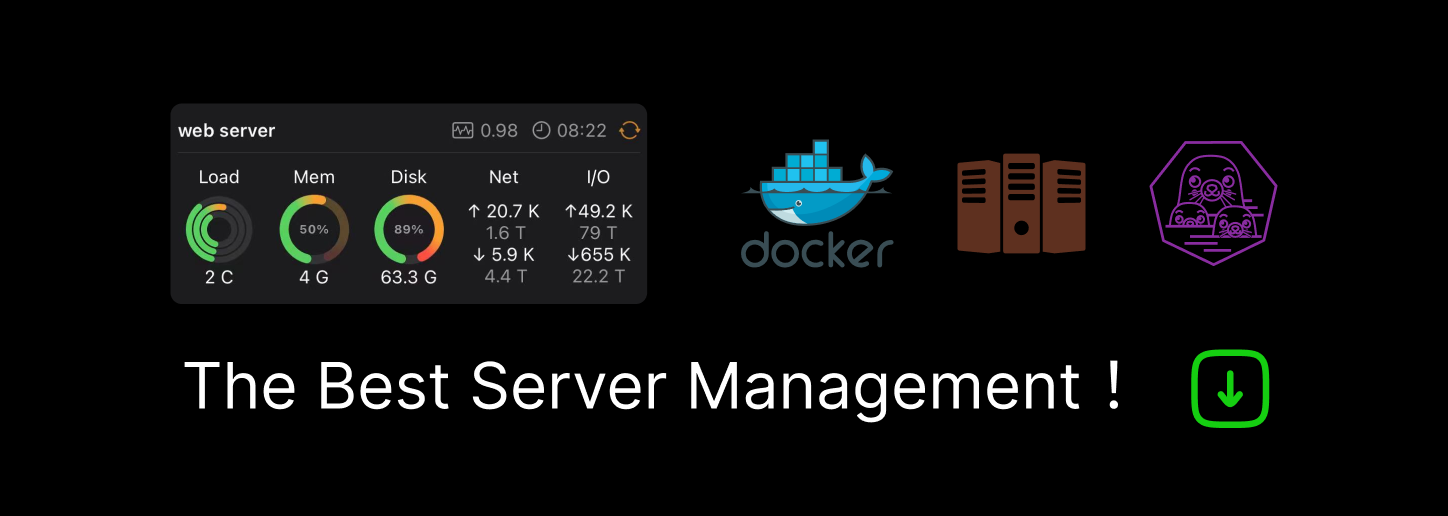
Angular 14 – Introducing Standalone Components
Angular 14 introduces Standalone Components. A component that is not part of ngModule.

From Angular 14 and higher versions, Standalone Components provide simplified and easier ways to create an angular application. A standalone component does not need a module or, more precisely, the ngModule. You can make the whole application without having to make any custom angular modules. Angular 14 allows you to create,
- Standalone Components
- Standalone Directives
- Standalone Pipes
You can use standalone components with
- Module-based components
- With other standalone components
- With routes
Besides the above options, you can also lazy load a standalone component.
Creating standalone component
You can create a standalone component by using the –-standalone flag in the ng generate component command:
ng g c login –standalone
After successful running of this command, you can find a Login Component added to the application as below. Here you notice that the standalone property of the component decorator is set to true.
import { Component, OnInit } from '@angular/core' ; import { CommonModule } from '@angular/common' ; @Component({ selector: 'app-login' , standalone: true , imports: [CommonModule], templateUrl: './login.component.html' , styleUrls: [ './login.component.css' ] }) export class LoginComponent implements OnInit { constructor() { } ngOnInit(): void { } } |
Any component, directive, or pipe with the standalone property set to true does not require to be part of any ngModule. If you try to add a standalone component to a module, Angular will complain about that by throwing an error as shown below,

You can make an existing component a standalone component by setting its standalone property to true.
Dependencies in Standalone component
A standalone component may depend on other members, pipes, and directives.
These dependencies can be divided into two parts,
- Standalone
- Part of a module
Both types of dependencies can be added to a standalone component using the imports array of the @Component decorator. For example, ReactiveFormsModule can be added to the LoginComponent by passing it to the imports array as shown in the below code listing:
@Component({ selector: 'app-login' , standalone: true , imports: [CommonModule, ReactiveFormsModule], templateUrl: './login.component.html' , styleUrls: [ './login.component.css' ] }) export class LoginComponent implements OnInit { |
If you want to use a component that is part of a module in a standalone component, pass that module in the imports array of the standalone component.
LoginComponent with a reactive form will look like below,
import { Component, OnInit } from '@angular/core' ; import { CommonModule } from '@angular/common' ; import { FormControl, FormGroup, ReactiveFormsModule } from '@angular/forms' ; const template = ` <form (ngSubmit)= 'loginUser()' [formGroup]= 'loginForm' novalidate class = "form" > <input formControlName= 'email' type= "text" class = "form-control" placeholder= "Enter Email" /> <input formControlName= 'password' type= "password" class = "form-control" placeholder= "Enter Password" /> <button [disabled]= 'loginForm.invalid' class = "btn btn-default" >Login</button> </form> ` @Component({ selector: 'app-login' , standalone: true , imports: [CommonModule, ReactiveFormsModule], template: template }) export class LoginComponent implements OnInit { loginForm: FormGroup; constructor() { this .loginForm = new FormGroup({ email: new FormControl( ' ' ), password: new FormControl( ' ' ) }) } ngOnInit(): void { } loginUser() { console.log( this .loginForm.status); console.log( this .loginForm.value); } } |
Using a Standalone component
You can use a standalone component, directive, or pipe in either of two ways:
- Inside another standalone component
- Inside a module
You need to pass It inside the imports array for both the options. Keep in mind that you don’t pass standalone components in the declaration array of the modules.
So to use it inside AppComponent, which is part of AppModule, you can pass it to the imports array as shown below :
@NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, AppRoutingModule, LoginComponent ], providers: [], bootstrap: [AppComponent] }) export class AppModule { } |
Now you can use it on the AppComponent as below,
< h1 >App</ h1 > < app-login ></ app-login > |
You can use standalone component in other standalone component by passing it to the imports property of the standalone component as shown below,
@Component({ selector: 'app-product' , standalone: true , imports: [CommonModule, LoginComponent], templateUrl: './product.component.html' , styleUrls: [ './product.component.css' ] }) export class ProductComponent implements OnInit { |
Bootstrapping Standalone Component
Angular 14 allows you to bootstrap the whole application using a standalone component. To bootstrap an application using a standalone component, follow the steps discussed below,
In the main.ts import the standalone component to be bootstrapped and bootstrapapplication function as shown below,
import {bootstrapApplication} from '@angular/platform-browser' ; import { ProductComponent } from './app/product/product.component' ; |
After that, call bootstrapapplication and pass the component in it as shown below,
bootstrapApplication(ProductComponent,{ providers:[] }); |
Next, on the index.html, replace app-root with your component.
< body > <!-- <app-root></app-root> --> < app-product ></ app-product > </ body > |
Now when you run the application, the application should bootstrap from ProductComponent. In the next post, we will talk in detail about
- dependency injection in a standalone component.
- Routing with standalone component
- Lazy loading standalone component
I hope you find this post valuable and are ready to explore the standalone component starting with Angular 14. Thanks for reading.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK