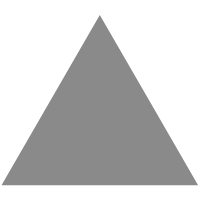
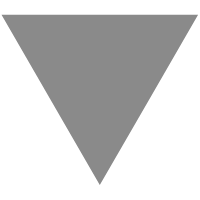
Did You know That users can modify your JavaScript variables from Browser consol...
source link: https://avikalpgupta.medium.com/did-you-know-that-users-can-modify-your-javascript-variables-from-browser-console-e7537ce85d62
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
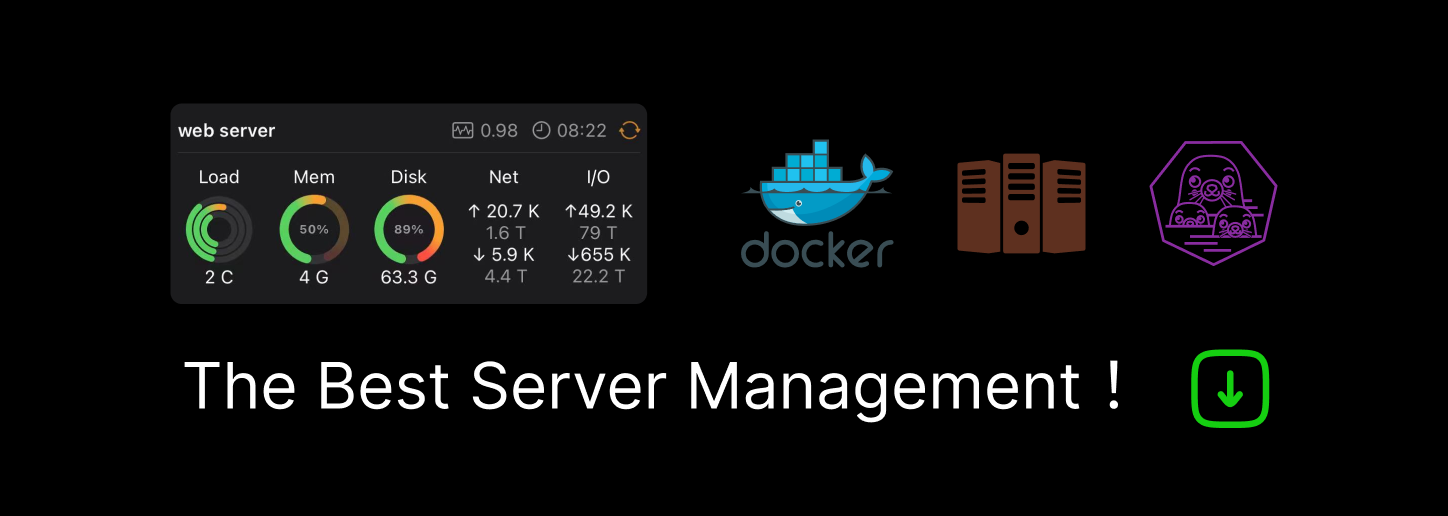
Did You know That users can modify your JavaScript variables from Browser console?
Almost all the websites built nowadays have some JavaScript code running within them. You wouldn’t believe how easy it is to mess with these scripts. If you too use client-side JS to make backend calls, read along!
The JavaScript in most browsers (tested in MS Edge and Chrome) manages its memory partitioning in such a manner that the console
and rest of the scripts in the displayed HTML file would run in the same environment. Which is good if you want to test something, like the final value of a variable, as a developer. But it leads to some possibilities of user behaviours that you might not end up being a fan of.
Especially because all your client-side code is easily accessible in the ‘source’ tab of the developer options. Have a look at GitHub.com’s client-side source code:

Client-side Source Code of GitHub’s website
I will show you some scenarios, which can demonstrate the problem, and then talk about the best practices that help mitigate the risks associated with this.
I will be using setTimeout
to emulate any delay in the execution of any part of your code. If your web-application makes any API call, or does some kind of complex processing which might take as much as 1 second to execute, your situation might match the ones shown here.
Accessing browser variables in HTML <script>
Introducing a variable from the browser console
If you run the above file in the browser, you will see what you expect: a Reference error for using a variable never defined.

Reference Error thrown for trying to use an undefined variable
But see what happens if I define the variable just in time.

Introducing a variable from the browser console
I performed this experiment to see if the console and the scripts share the same memory space. I thought that they wouldn’t, as this might raise security questions. But when I saw the output,
MY MIND WAS BLOWN!!
I started testing the limits of this. Next, I tried to modify a variable that the current script uses.
And right in front of my eyes, I saw myself attacking my own code with fraudulent values:

Modifying a script variable from browser console
So this is clearly a security risk! But is it, really? Most of the work we do is inside functions. Does this work with variables declared within functions as well?
Changing variables inside a function
This is the expected output:

Expected output of the script without any intrusions
And this is what happened when I tried to mess with function f1()
's variables:

Browser console was not able to budge a `let` variable inside a function
Like a mother, the function f1
protected its child variable! Outsiders would never be able to hurt the function’s variables, as long as the function is alive. Once the function execution has started, it will work as expected. Of course, the browser console attacker can re-write the whole function if the function execution does not start immediately on page load (which is often the case with most applications).
Modifying whole functions from the browser console
Many of our functions are called from user interactions. Consider:
See how the mother perishes while saving her children:

Function definition perishes to the browser-console attacker
At least, the mother died heroically… Or did she?
let
variables, as well as var
and const
variables have a scope limited to the function’s definition, as we had learnt in this article:
var, let, const and undeclared variables in JavaScript
Everything you need to know about the different variable declarations in JavaScript and the best practices associated…
What if we used an undeclared variable inside the function? Is that variable at risk of the browser-console attacker? Let’s see
Modifying variables within a function from the browser console
Here, a
is clearly an undeclared variable, while b
is declared with var
. We are sure that b
would be protected, but is a
safe?

Normal Expected output of the code

When happens when we try to modify the variables inside the function
As we feared, a
got modified.
How to write secure JavaScript client-side code
But how can we safeguard our code from such risks? The answer was clear from the experiments we performed!
Safeguarding variables
- Always use scoped variables! Which means, ALWAYS declare your variables (using
var
,let
orconst
). In fact, this article recommends that we uselet
andconst
in every case. - Use
const
for defining unchanging parameters to guarantee immutability. This would work even in the global scope! Here is an example:
Here is what happens when the browser-console attacker tries to modify your constant:

Perfectly safe!
Safeguarding functions
Again, const
is the answer! Instead of defining the functions directly, it’d be much safer to create anonymous functions and assigning them to constants. Reasons:
const
would ensure that the constant value of the function is immutable- Assigning an anonymous function would ensure that you and any of your team members would only use the
const
value for calling the function, and not accidently call the function name rather than theconst
it was assigned to.
Recall that we can define anonymous functions using the arrow syntax as well:
const f1 = () => {
// function code here
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK