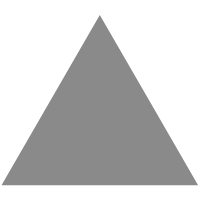
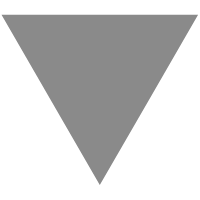
javascripts中的map
source link: https://zhangrr.github.io/posts/20220523-javascripts_map/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
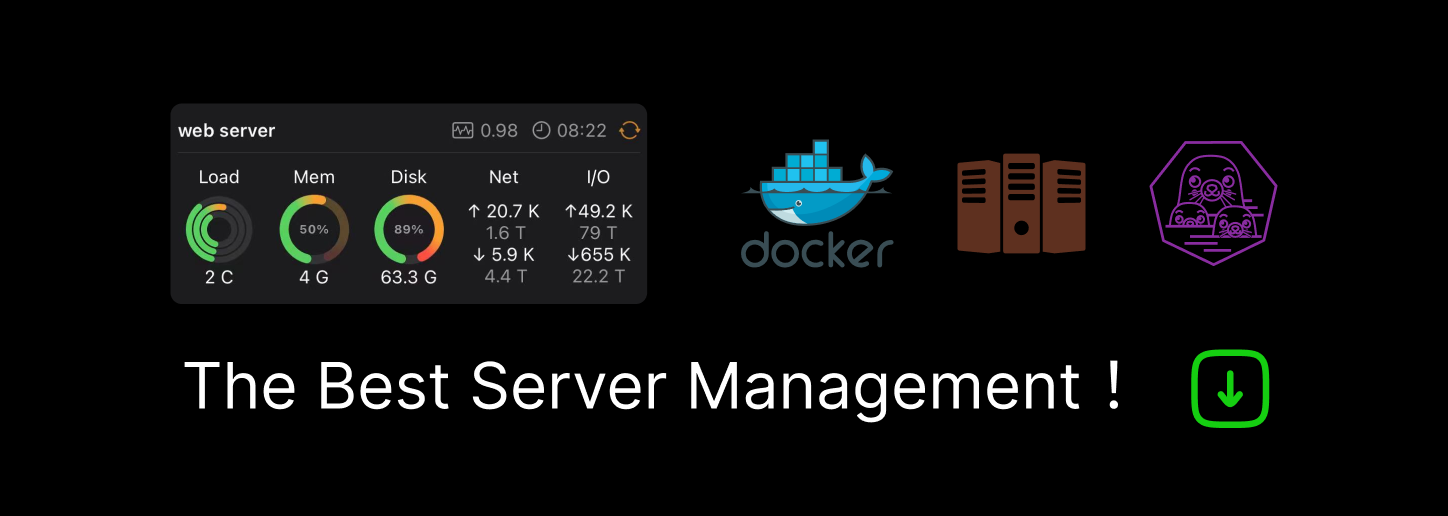
Javascripts中的map
Javascripts 中的 map
说到map,如果我们有循环的话,不停遍历,会把时间搞成 O(n)。如果有map,时间就变成了O(1),所以还是相当有效率的。
map 是 ES2015 中新增加的,是一种新的 Object 类型,允许存放各种 key-value 对。
map 最大的特性是,key 可以是任何类型,包括 object 和 function;可以调用 size 得到 map 的大小;迭代的时候,是严格按照添加的顺序进行迭代的。
map 的方法如下: set, get, size, has, delete, clear:
let things = new Map();
const myFunc = () => '🍕';
things.set('🚗', 'Car');
things.set('🏠', 'House');
things.set('✈️', 'Airplane');
things.set(myFunc, '😄 Key is a function!');
things.size; // 4
things.has('🚗'); // true
things.has(myFunc) // true
things.has(() => '🍕'); // false, not the same reference
things.get(myFunc); // '😄 Key is a function!'
things.delete('✈️');
things.has('✈️'); // false
things.clear();
things.size; // 0
// setting key-value pairs is chainable
things.set('🔧', 'Wrench')
.set('🎸', 'Guitar')
.set('🕹', 'Joystick');
const myMap = new Map();
// Even another map can be a key
things.set(myMap, 'Oh gosh!');
things.size; // 4
things.get(myMap); // 'Oh gosh!'
迭代它的方法,可以用 for … of,顺序是严格按照你插入的顺序来的:
let activities = new Map();
activities.set(1, '🏂');
activities.set(2, '🏎');
activities.set(3, '🚣');
activities.set(4, '🤾');
for (let [nb, activity] of activities) {
console.log(`Activity ${nb} is ${activity}`);
}
// Activity 1 is 🏂
// Activity 2 is 🏎
// Activity 3 is 🚣
// Activity 4 is 🤾
也可以用 forEach 来迭代,注意一点即可,forEach 的 callback 函数,第一个参数是 value,第二个参数是 key,跟 for … of 是相反的:
activities.forEach((value, key) => {
console.log(`Activity ${key} is ${value}`);
});
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK