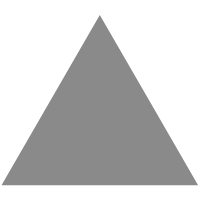
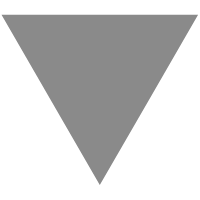
draft!! dot parenthesis
source link: https://ppsteven.github.io/pages/5d6302/#%E6%A1%88%E4%BE%8B
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
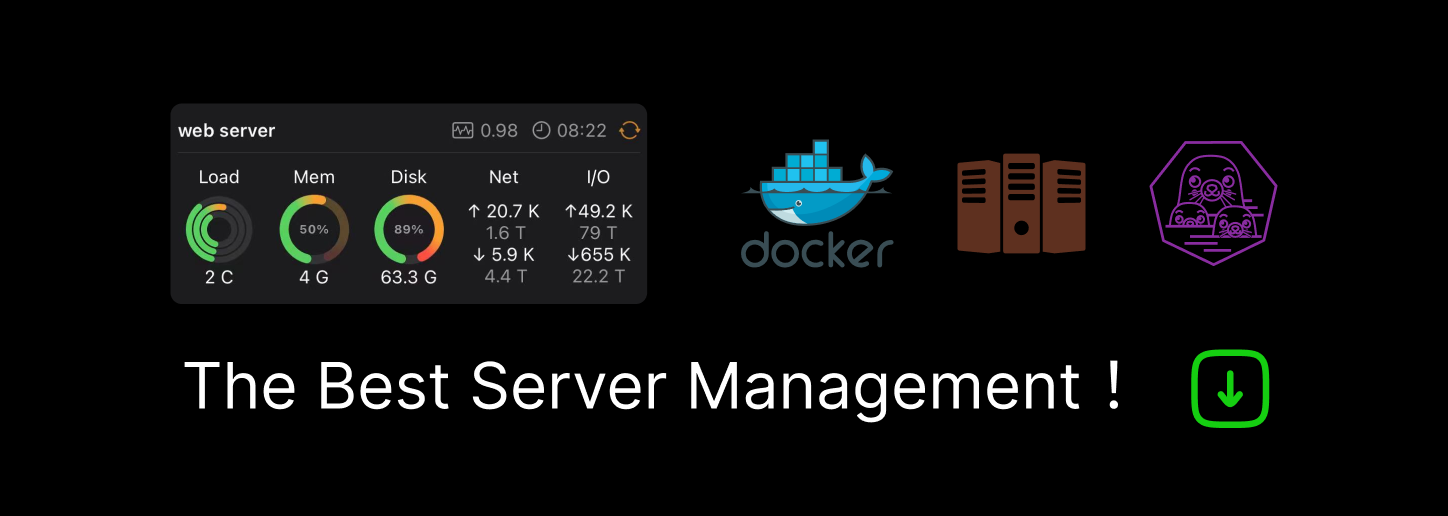
draft!! dot parenthesis
# Type Switch
- https://golang.org/doc/effective_go#type_switch
var t interface{}
t = functionOfSomeType()
switch t := t.(type) {
default:
fmt.Printf("unexpected type %T\n", t) // %T prints whatever type t has
case bool:
fmt.Printf("boolean %t\n", t) // t has type bool
case int:
fmt.Printf("integer %d\n", t) // t has type int
case *bool:
fmt.Printf("pointer to boolean %t\n", *t) // t has type *bool
case *int:
fmt.Printf("pointer to integer %d\n", *t) // t has type *int
}
2
3
4
5
6
7
8
9
10
11
12
13
14
# Type Assertion
Type Switch 使用了 type
标识符,如果我们只关心一种类型的话,可以使用确定 的 type
类型来替代 type
标识符,如 value.(typeName)
如果 interface{}
中不包含类型 typeName
就会报错。
var i interface{}
i = int(42)
a, ok := i.(int)
// a == 42 and ok == true
b, ok := i.(string)
// b == "" (default value) and ok == false
2
3
4
5
6
7
8
type Stringer interface {
String() string
}
if str, ok := value.(string); ok {
return str
} else if str, ok := value.(Stringer); ok {
return str.String()
}
2
3
4
5
6
7
8
9
# 案例
由于历史原因或代码对接过程中没有做到统一数据类型
如出现下面两种类型
type Message struct {
Name string
Time string
}
type Message struct {
Name string
Time float64
}
2
3
4
5
6
7
8
处理的方式就是结合使用 interface{}
和 type switch
type Message struct {
Name string
Time interface{}
}
b := []byte(`{"Name":"Wednesday","Time":6}`)
var f Message
json.Unmarshal(b, &f)
switch v := f.Time.(type) {
case float64:
fmt.Printf("Name: %v; Time: %v\n", f.Name, v)
fmt.Printf("Time type %T!\n", v)
case string:
fmt.Printf("Name: %v; Time: %v\n", f.Name, v)
fmt.Printf("Time type %T!\n", v)
default:
fmt.Printf("I don't know about type %T!\n", v)
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
Recommend
-
126
"dotfiles" and system configuration These dotfiles are affectionately dedicated to the vi editor originally created by Bill Joy, with whom I have spent many pleasant evenings
-
102
-
137
dot-osk/monitor_ctrl: DDC/CI master
-
38
vim-parinfer - vim plugin to balance your parenthesis
-
43
Let’s dig in a not-well-publicized ES6 feature: calling functions without using parenthesys. If you are familiar with Ruby, you know that in Ruby you can omit parenthesis when they’re not ambiguous: pu...
-
31
Dot Dot Considered Harmful Child processes on Fuchsia are only capable of accessing the resources provided to them -- this is an essential idea encompassing microkernels, and other “capability-based” systems. I...
-
7
An unmatched left parenthesis... ...creates an unresolved tension that will stay with you all day. 25 August 2016 (relevant XKCD) Consider the followi...
-
4
What is awesome-pair.el ? awesome-pair.el is a plugin that provides grammatical parenthesis completion. Installation Clone or download this repository (path of the folder is the <path-to-awesome-pair>
-
2
Conversation Contributor
-
2
Parenthesis CheckerJanuary 06, 2024 |80 ViewsSDE Sheet - Parenthesis CheckerSDE Sheet, GFG SDE Sheet, stack Save Share LikeDescriptionDiscussion
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK