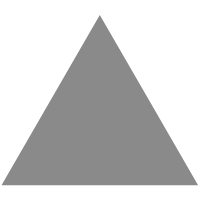
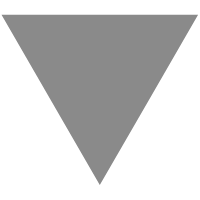
Introduction to HttpClient
source link: https://blog.oio.de/2019/12/23/introduction-to-httpclient/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
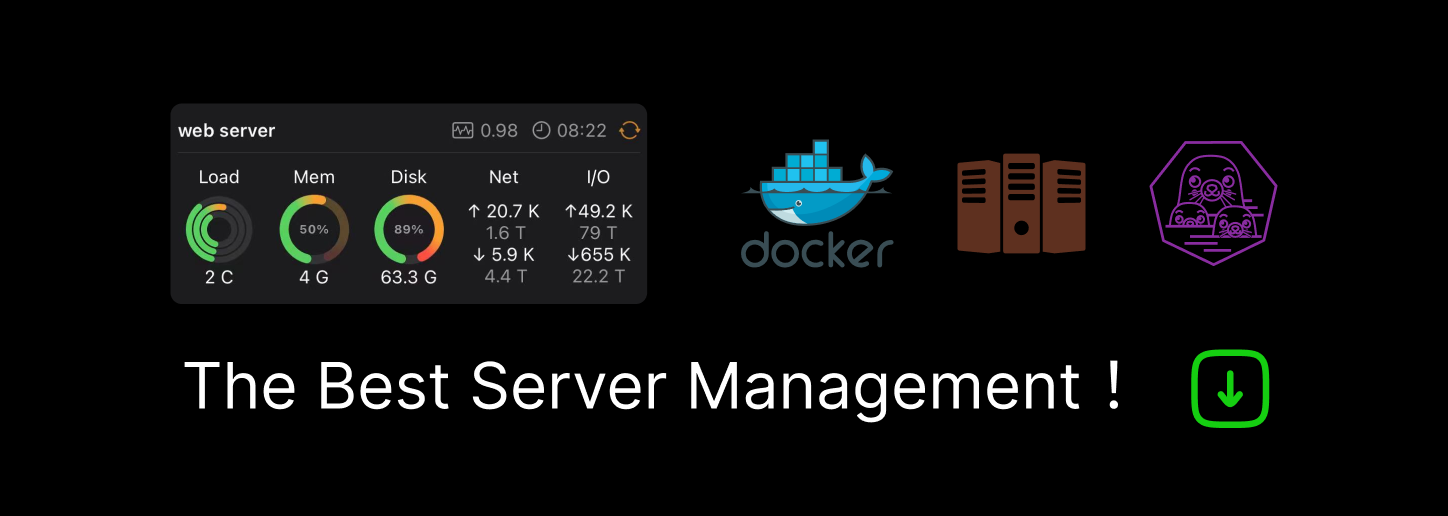
Introduction to HttpClient
And now let’s GET some info booster POSTED in the spirit of the winter holidays …
This article shows you how to use the new Java 11 HtppClient
API to send HTTP GET/POST
requests using two very common examples.
HttpClient
provides synchronous and asynchronous request mechanisms:
send(HttpRequest, BodyHandler)
blocks until the request has been sent and the response has been received.
sendAsync(HttpRequest, BodyHandler)
sends the request and receives the response asynchronously.
In this article, I will focus on the synchronous GET request.
I will explain the following code for GET
step by step:
HttpClient httpClient = HttpClient.newBuilder().build(); HttpRequest mainRequest = HttpRequest.newBuilder() .build(); HttpResponse<String> response; try { response = httpClient.send(mainRequest, BodyHandlers.ofString()); System.out.println(response.body()); } catch (IOException e) { e.printStackTrace(); } |
The HttpClient
is generated by a builder which is used to configure the per-client state. The HttpClient
once built is immutable and can be used to send multiple requests.
The HttpClient.Builder
contains methods such as:
authenticator(Authenticator auth)
– used for HTTP authenticationconnectTimeout(Duration duration)
– used to set the connection timeout for the specific clientcookieHandler(cookieHandler cookieHandler)
– for setting the cookie handlerfollowRedirects(HttpClient.Redirect policy)
– used to define if requests will automatically follow the redirects issued by the serverproxy(ProxySelector proxySelector)
– used to set aProxySelector
. This selects the proxy server to use, if any, when connecting to the network resource referenced by a URL.sslContext(SSLContext sslContext)
– for setting theSSLContext
version(HTTPClient.Version version)
– to request a specific HTTP protocol version if possible
In our example the following response is generated:
HttpResponse<String> response = httpClient.send(mainRequest, BodyHandlers.ofString());
A BodyHandler has to be supplied for every HttpRequest
sent because it determines how to handle the response body if it exists.
In the example above an ofString
BodyHandler
is used in order to convert the response body into a String
. The BodyHandler
is invoked once the response status code and headers are available, but before the response body bytes are received.
There are a few methods using the predefined body handlers to convert a stream of response body data into common high-level Java objects.
We have seen the response body handled as a String
but it can also be received as:
- a file:
HttpResponse<Path> response = httpClient.send(mainRequest, BodyHandlers.ofFile(Paths.get( "index.html" ))); |
- an input stream:
HttpResponse<InputStream> response = httpClient.send(mainRequest, BodyHandlers.ofInputStream()); |
- discarded:
HttpResponse<Void> response = httpClient.send(mainRequest, BodyHandlers.discarding()); |
Now it’s time to take a look at HTTP POST
:
An example of it is the following:
HttpRequest mainRequest = HttpRequest.newBuilder().POST(BodyPublishers.ofString( "Send me!" )) .header( "Content-Type" , "text/plain" ) .build(); try { httpClient.send(mainRequest, BodyHandlers.ofString()); } catch (IOException e) { e.printStackTrace(); } |
HttpRequest
describes an object which represents the request we want to send. New instances can be created using HttpRequest.Builder
.
The Builder
class provides some methods we can use for the configuration of our request. The first step is to specify the HTTP method. In our case this is POST. If you do not know what the others HTTP methods are, here is the answer:
GET
POST(HttpRequest.BodyPublisher body)
PUT(HttpRequest.BodyPublisher body)
DELETE()
A predefined body publisher is used to convert common high-level Java objects into a stream of data suitable for being sent as a request body. In our example a request body derived from a String is used but there are much more methods like BodyPublisher
:
fromPublisher(Flow.Publisher<? extends ByteBuffer> publisher
) – This method is used as an adapter betweenBodyPublisher
andFlow.Publisher
, where the size of the request body that the publisher will publish is unknown.
fromPublisher(Flow.Publisher<? extends ByteBuffer> publisher, long contentLength)
– Unlike with the method above, the size of the request body that the publisher will publish is known.
noBody()
– is a method of a request body publisher which sends no request body
ofByteArray(byte[] buf)
– the method returns a request body publisher whose body is the given byte array.
ofByteArray(byte[] buf, int offset, int length
) – compared to the method above, here the body is the content of the given byte array oflength
bytes starting from the specifiedoffset
.
ofByteArrays(Iterable<byte[]> iter)
– this is an example of a request body publisher from an Iterable of byte arrays.
ofFile(Path path)
– here the data is taken from the contens of a file
ofInputStream(Supplier<? extends InputStream> streamSupplier)
– aSupplier
of anInputStream
is used if the request needs to be repeated, as the content is not buffered. TheSupplier
may returnnull
on subsequent attempts, in which case the request fails.
ofString(String s, Charset charset)
– which returns a request body publisher whose body is the givenString
converted using the given character set.
The next step is setting the header in this example to a single key-value header.
A very important thing to do when creating a request is to provide the URL. Using the uri()
method we can set the URL, in our case http://localhost:8080/postIt
.
This is the principle of creating a synchronous HTTP request. Some more info is on its way in the next blog posts to come.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK