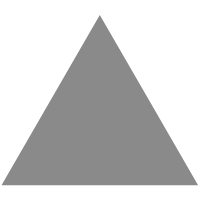
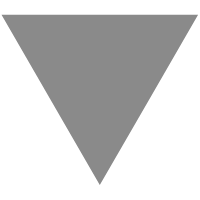
CompletableFuture和Future介绍
source link: https://perkins4j2.github.io/posts/13999/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
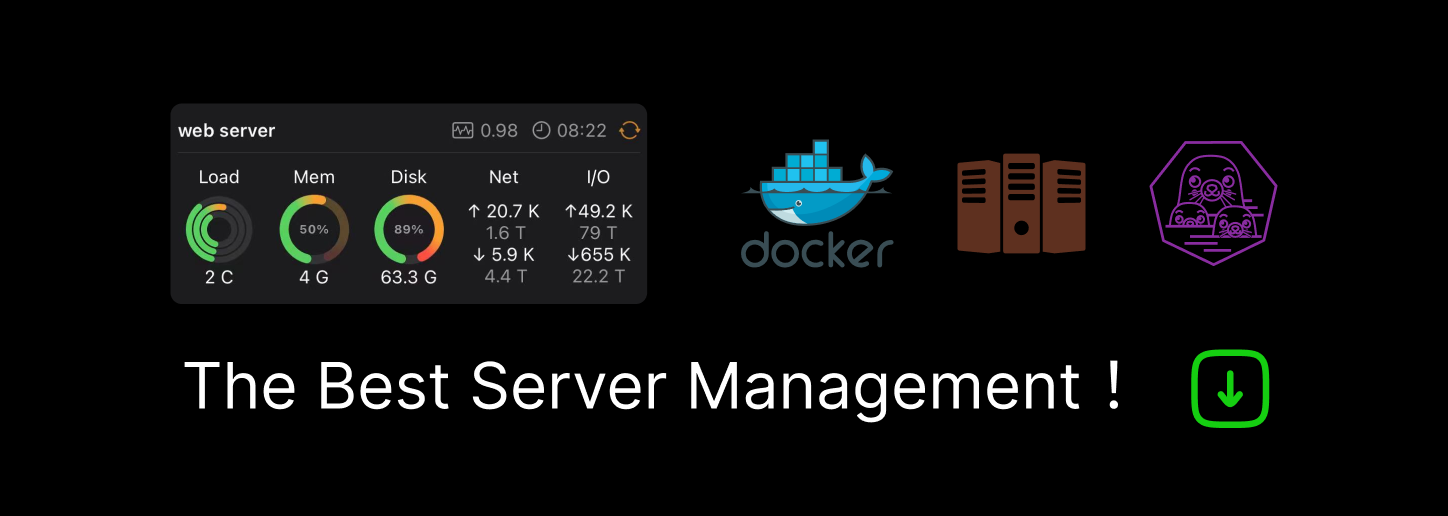
CompletableFuture和Future介绍
Future是JDK1.5后提供的异步调用接口。
CompletableFuture是JDK8提出的一个支持非阻塞的多功能的异步类,实现了Future。
与Future区别
- CompletableFuture支持回调,可指定线程池
- CompletableFuture支持链式、顺序和聚合等执行
- CompletableFuture支持不阻塞主线程且无需轮询等待状态
- 都是提交后直接执行,并在get时阻塞等待
CompletableFuture主要方法
- thenRun,前一个执行后再同步执行
- thenRunAsync,前一个执行后再异步执行
- runAsync,无返回值的异步执行
- supplyAsync,有返回值的异步执行
- allOf,聚合多个调用
- get,阻塞等待执行完成
CompletableFuture异步执行Demo
public class CompletableFutureTest { |
Future 阻塞Demo
while (true) { |
CompletableFuture-allOf问题
allOf等待所有任务执行完成,直到timeout超时。
CompletableFuture |
若timeout超时后,所有任务均返回失败;类似事务操作,导致已获取结果的任务后续处理异常,无法容错。
正确使用方法
allOf不限制等待时间,子任务内部限定。具体操作如下:
@Slf4j |
调用方法:
CompletableFutureUtil completableFutureUtil = CompletableFutureUtil.newInstance(); |
- 异步任务内部进行限定超时时间,runAsync(Runnable runnable, int timeout)
- 内部由定时任务进行扫描和容错处理(返回null),es.schedule(() -> f.complete(null), timeout, TimeUnit.MILLISECONDS);
- allOf不限定执行时候,直接get等待
本文标题:CompletableFuture和Future介绍
文章作者:Perkins
发布时间:2020年05月19日
原始链接:https://perkins4j2.github.io/posts/13999/
许可协议: 署名-非商业性使用-禁止演绎 4.0 国际 转载请保留原文链接及作者。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK