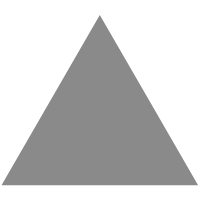
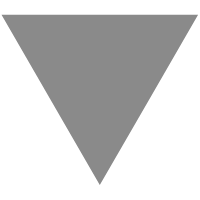
Python 简明教程 --- 16,Python 高阶函数
source link: https://codeshellme.github.io/2020/06/python-learn16/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
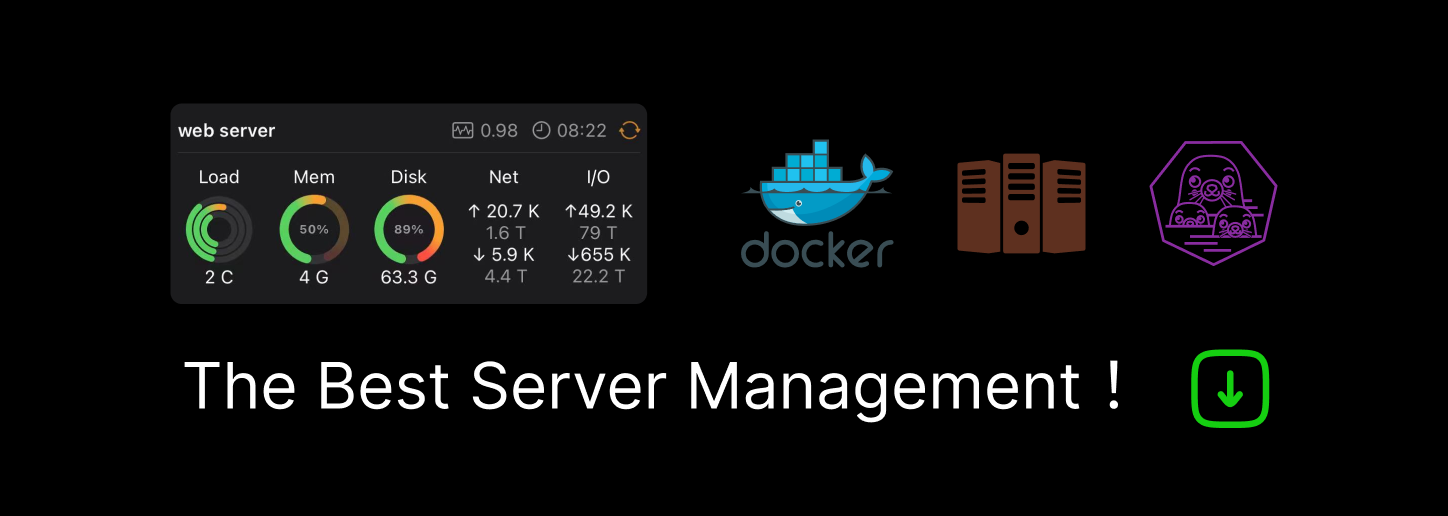
微信公众号:码农充电站pro
对于那些快速算法,我们总是可以拿一些速度差不多但是更容易理解的算法来替代它们。
—— Douglas Jones
目录
高阶函数
一般以函数为参数。
本节我们介绍Python 中三个方便的高阶函数,分别是:
- reduce
- filter
如果你了解过分布式系统框架—Hadoop
,你应该知道map/reduce
的概念。
Python 中的map/reduce
函数与Hadoop
中的map/reduce
基本类似。
1,map 函数
在Python2
中,map
是一个函数。在Python3
中,map
是一个类:
>>> map <class 'map'> |
作用:将函数
func
作用于可迭代对象iterable
中的每一个元素原型:map(func, iterable) -> map object
参数 func:一个函数类型的参数,该函数接收一个参数,并返回一个值
参数 iterable:一个可迭代的对象
返回值:一个
map
对象,同时一个迭代器
>>> m = map(lambda x: x * x, [1, 3, 5]) >>> m <map object at 0x7fe60bb1bf98> >>> l = [i for i in m] >>> l [1, 9, 25] |
参数func
可以是普通函数,也可以是匿名函数
,我们这里使用了匿名函数lambda x: x * x
,该函数接收一个参数,并返回该参数的平方。
可迭代对象是一个列表[1, 3, 5]
,列表中的每个元素,都将传递到匿名函数中,并对每一个元素计算平方后,将结果存储到一个map
对象m
中。
为了查看m
中的值,我们使用列表推导式
生成了一个列表l
,可看到列表l
就是[1, 9, 25]
,就是[1, 3, 5]
中每个元素的平方。
这就是python3 中map
的基本使用方法。
2,reduce 函数
在Python3 中,reduce
函数被放在functools
模块,使用时,要先从functools
模块引入:
>>> from functools import reduce |
作用:将函数
func
作用于序列seq
中的元素,进行一系列的计算原型:reduce(func, seq[, initial]) -> value
参数 func:这是一个函数类型的参数,该函数接收两个参数,并返回一个值
参数 seq:一个序列
参数 initial:
当
initial
存在时:reduce(func, [x1, x2, x3], initial) = func(func(func(initial, x1), x2), x3)
当
initial
不存在时:reduce(func, [x1, x2, x3]) = func(func(x1, x2), x3)
返回值:返回计算结果
示例,当initial
存在时:
>>> reduce(lambda x, y : x * y, [1, 3, 5], 6) # reduce(func, [1, 3, 5], 6) # = func(func(func(6, 1), 3), 5) # = ((6 * 1) * 3) * 5 |
示例,当initial
不存在时:
>>> reduce(lambda x, y : x * y, [1, 3, 5]) # reduce(func, [1, 3, 5]) # = func(func(1, 3), 5) # = (1 * 3) * 5 |
3,filter 函数
在Python3 中,filter
是一个类:
>>> filter <class 'filter'> |
作用:filter 用于对
可迭代
对象iterable
进行过滤,iterable
中的每一个元素会作为一个参数,传递到func
中原型:filter(func, iterable) –> filter object
参数 func:这是一个函数类型的参数,该函数接受一个参数,返回一个
bool
值当
func
返回True
时:对应的iterable
中的元素,会放在结果集中当
func
返回False
时:对应的iterable
中的元素,不会放在结果集中返回值:一个
filter
对象,同时也是一个迭代器
>>> f = filter(lambda x: len(x) == 2, ['dsf', 'df', 'ad', 'dfas', 'as']) >>> f # 一个 filter 对象 <filter object at 0x7f4dff484748> >>> list(f) # 转化为列表,方便查看 ['df', 'ad', 'as'] |
推荐阅读:
Python 简明教程 — 14,Python 数据结构进阶
欢迎关注作者公众号,获取更多技术干货。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK