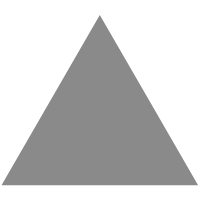
3
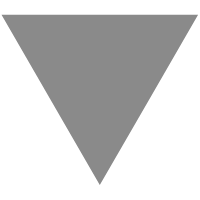
golang中的工厂模式
source link: https://cloudsjhan.github.io/2018/08/27/golang%E4%B8%AD%E7%9A%84%E5%B7%A5%E5%8E%82%E6%A8%A1%E5%BC%8F-md/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
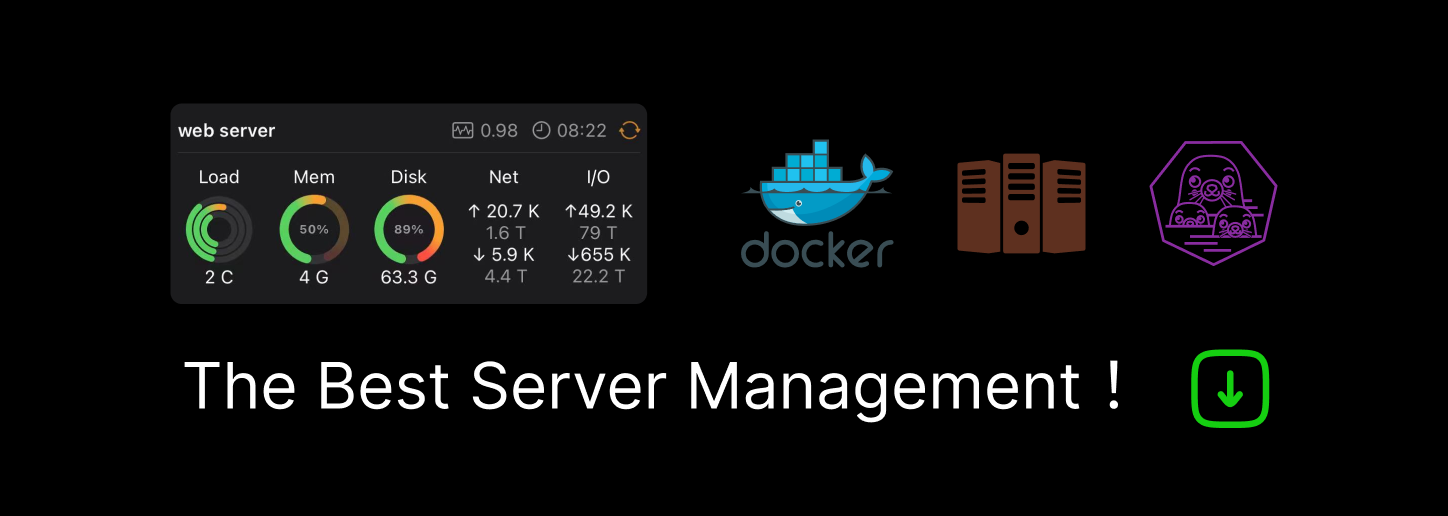
golang中的工厂模式
- 研究go的设计模式,必须了解go的struct和interface,若不熟悉,先阅读以下内容
- go语言的struct
- go语言的interface
* 简单工厂模式 |
* 工厂方法 |
* 抽象工厂方法 |
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK