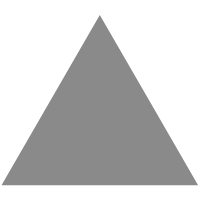
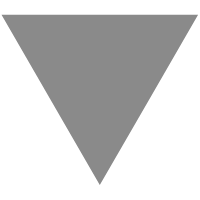
LeetCode刷题之Golang模版
source link: https://shaoyuanhangyes.github.io/2021/05/13/LeetCode%E5%88%B7%E9%A2%98%E4%B9%8BGolang%E6%A8%A1%E7%89%88/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
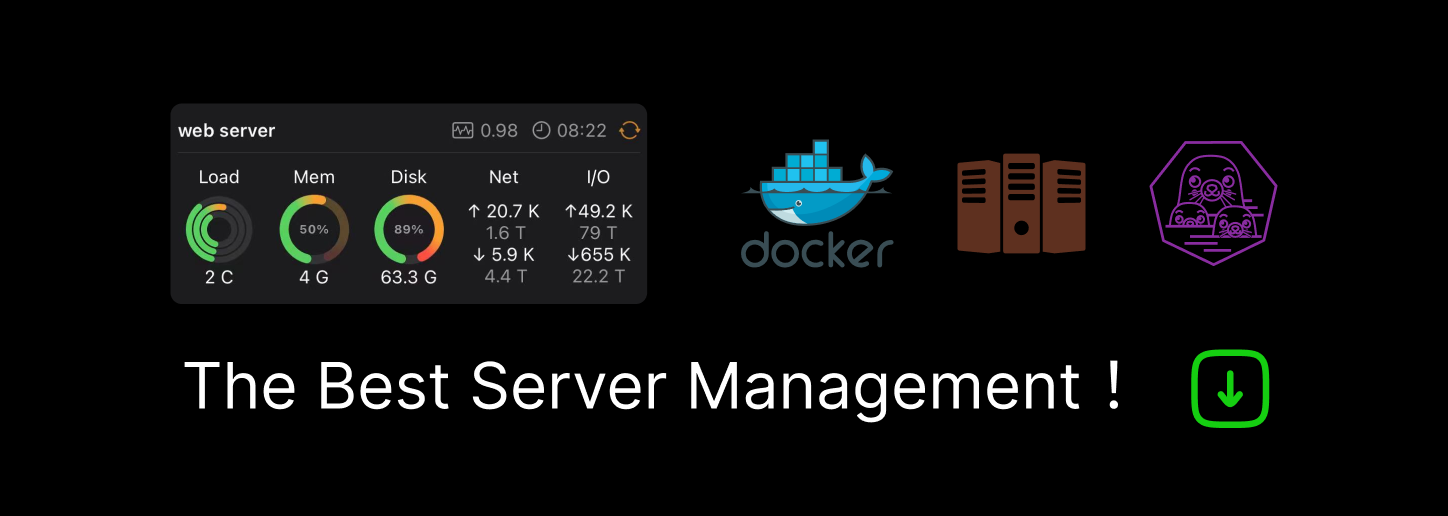
LeetCode刷题之Golang模版
发表于
2021-05-13 更新于 2021-08-25 分类于 Go
在LeetCode上刷题时 有的时候自己无法理解和追踪某个变量的变化 因此需要debug代码 但LeetCode的debug功能很鸡肋 不如自己在本地编译器中进行断点调试 但LeetCode的解题只需要写出功能的函数即可 所以我们需要自己补写出其他函数来保证debug的成功
package main
import (
"fmt"
)
//链表结构体
type ListNode struct {
Val int
Next *ListNode
}
//使用数组创建对应的链表 后插法
func createList(nums []int) *ListNode {
prev := new(ListNode)
prev.Val = nums[0]
prevHead := new(ListNode)
prevHead = prev
for i:=1;i<len(nums);i++ {
temp := new(ListNode)
temp.Val = nums[i]
prev.Next = temp
prev = temp
}
return prevHead
}
//显示链表中的所有元素
func ShowList(L *ListNode) {
temp := L
for temp != nil {
fmt.Print(temp.Val," ")
temp = temp.Next
}
fmt.Println()
}
//二叉树结构体
type TreeNode struct {
Val int
Left *TreeNode
Right *TreeNode
}
//创建二叉树
func createTree(nums []int, len int, index int) *TreeNode {
root := new(TreeNode)
if index < len && nums[index] != -1 {
root.Val = nums[index]
if 2*index+1 < len {
root.Left = createTree(nums, len, 2*index+1)
}
if 2*index+2 < len {
root.Right = createTree(nums, len, 2*index+2)
}
}
return root
}
//二叉树的层序遍历 输出到一个二维数组中
func levelOrder(root *TreeNode) [][]int {
res := [][]int{}
if root == nil {
return res
}
queue := []*TreeNode{root}
for i := 0; len(queue) > 0; i++ {
res = append(res, []int{})
temp := []*TreeNode{}
for j := 0; j < len(queue); j++ {
node := queue[j]
res[i] = append(res[i], node.Val)
if node.Left != nil {
temp = append(temp, node.Left)
}
if node.Right != nil {
temp = append(temp, node.Right)
}
}
queue = temp
}
return res
}
//打印二维数组
func PrintMartrix(res [][]int) {
for i := 0; i < len(res); i++ {
fmt.Print("[")
for j := 0; j < len(res[i]); j++ {
fmt.Print(" ", res[i][j], " ")
}
fmt.Println("]")
}
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK