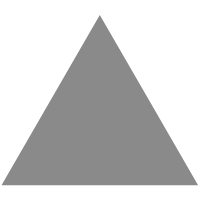
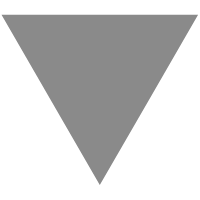
[Golang] Insert Line or String to File
source link: https://siongui.github.io/2017/01/30/go-insert-line-or-string-to-file/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
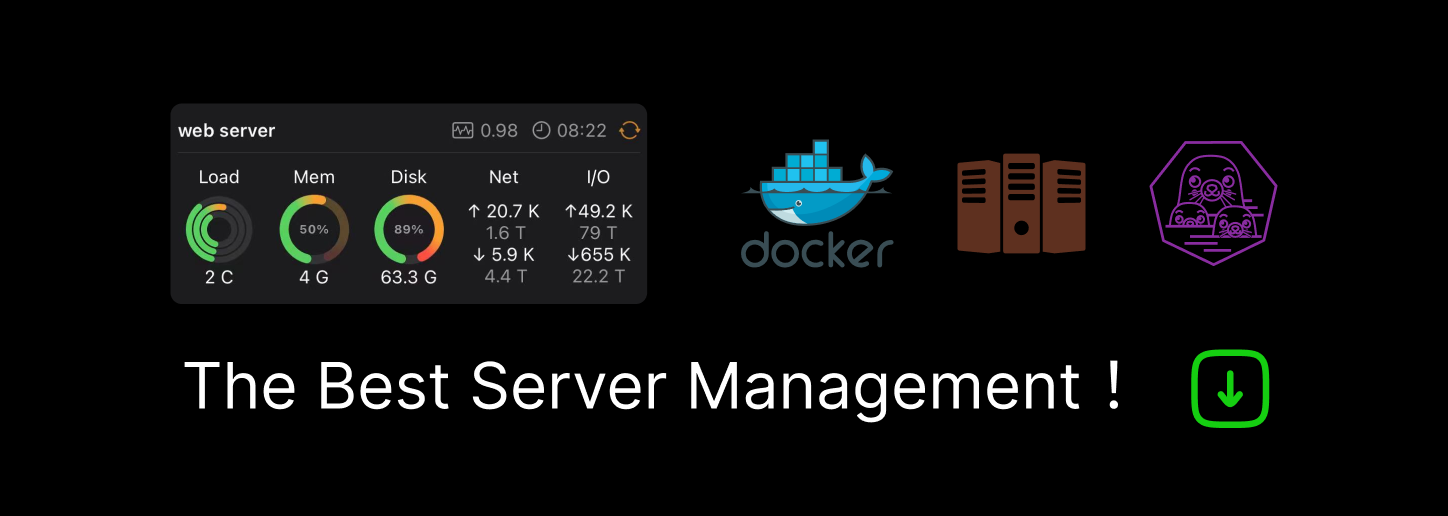
[Golang] Insert Line or String to File
January 30, 2017Insert a string or line to the position of n-th (0-indexed) line of the file via Golang.
The logic of the code:
- Read the file line by line into a slice of type string [1].
- for range the string slice to insert the string to n-th line of the file. Note that line is actually a string with trailing newline '\n'. If you want to insert a line, just append '\n' to the end of the string.
The following is full source code:
utils.go | repository | view rawpackage insert import ( "bufio" "io" "io/ioutil" "os" ) func File2lines(filePath string) ([]string, error) { f, err := os.Open(filePath) if err != nil { return nil, err } defer f.Close() return LinesFromReader(f) } func LinesFromReader(r io.Reader) ([]string, error) { var lines []string scanner := bufio.NewScanner(r) for scanner.Scan() { lines = append(lines, scanner.Text()) } if err := scanner.Err(); err != nil { return nil, err } return lines, nil } /** * Insert sting to n-th line of file. * If you want to insert a line, append newline '\n' to the end of the string. */ func InsertStringToFile(path, str string, index int) error { lines, err := File2lines(path) if err != nil { return err } fileContent := "" for i, line := range lines { if i == index { fileContent += str } fileContent += line fileContent += "\n" } return ioutil.WriteFile(path, []byte(fileContent), 0644) }
Usage:
Assume we have a text file named test.txt as follows:
We want to insert a line with text hello world! to the third line (index 2) of the file. Call InsertStringToFile("test.txt", "hello world!\n", 2)
utils_test.go | repository | view rawpackage insert import "testing" func TestCountLeadingSpace(t *testing.T) { err := InsertStringToFile("test.txt", "hello world!\n", 2) if err != nil { t.Error(err) } }
The result is as follows
1 2 hello world! 3 4 5
Tested on:
- Ubuntu Linux 16.10
- Go 1.7.5
References:
[2][Golang] Append Line/String to File[5]insert sting to n-th line of file · siongui/go-rst@3bdfb1a · GitHub
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK