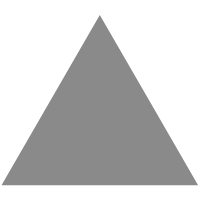
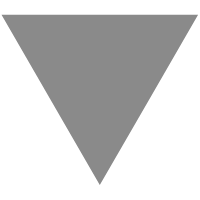
Golang html/template versus Python Jinja2 (4) - Arrays and Slices Index
source link: https://siongui.github.io/2015/03/06/python-jinja2-vs-go-html-template-array-slice-index/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
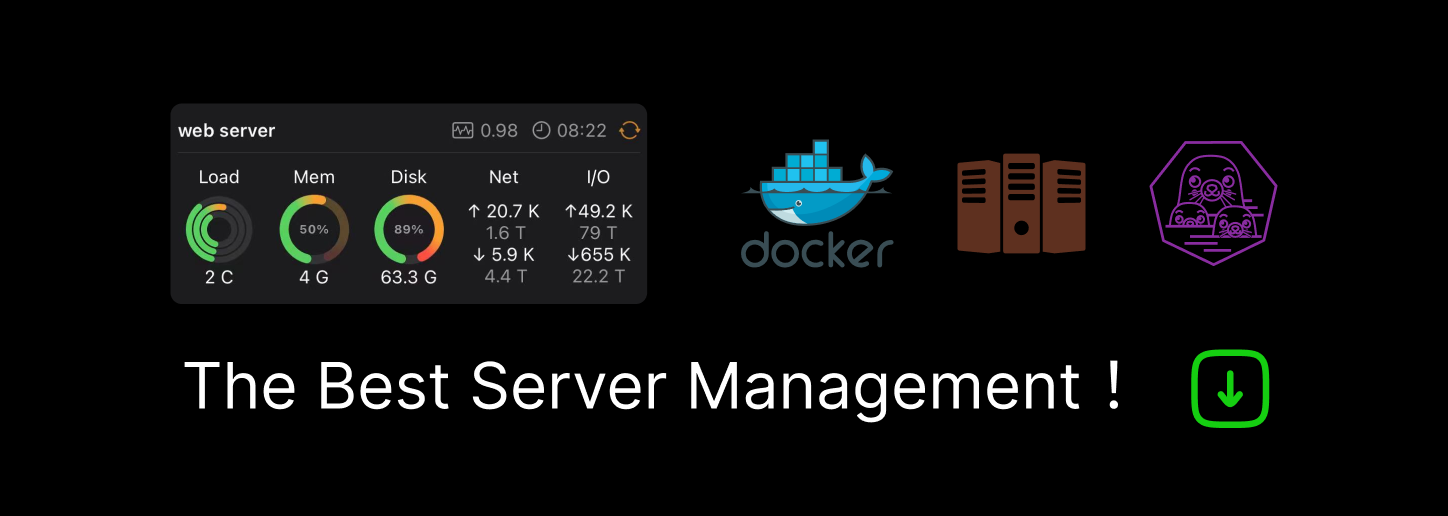
Golang html/template versus Python Jinja2 (4) - Arrays and Slices Index
March 06, 2015When loop through the arrays/slices (Go) or lists (Python) in templates, sometimes the loop index is needed. This post shows how to access loop index in Python Jinja2 and Go html/template.
In Jinja2, loop.index or loop.index0 is used to access the loop index, starting from 1 or 0. (see [a])
In Go html/template, one more variable is declared in range action to access loop index, starting from 0. (see [b])
Go html/template versue Python Jinja2 - Arrays and Slices Index Go html/template Python Jinja2
template:
{{range $index, $link := .}} {{$index}}: <a href="{{$link.Href}}">{{$link.Name}}</a> {{end}}
template:
{# index start from 0 #} {% for link in links %} {{loop.index0}}: <a href="{{link.href}}">{{link.name}}</a> {% endfor %}
{# index start from 1 #} {% for link in links %} {{loop.index}}: <a href="{{link.href}}">{{link.name}}</a> {% endfor %}
template values:
type Link struct { Name string Href string } // arrays var la [2]Link la[0] = Link{"Google", "https://www.google.com/"} la[1] = Link{"Facebook", "https://www.facebook.com/"} // slices var ls []Link ls = append(ls, Link{"Google", "https://www.google.com/"}) ls = append(ls, Link{"Facebook", "https://www.facebook.com/"})
template values:
links = [ {'name': 'Google', 'href': 'https://www.google.com'}, {'name': 'Facebook', 'href': 'https://www.facebook.com'} ]
Complete Go html/template source code:
html-template-example-3.go | repository | view rawpackage main import ( "html/template" "os" ) const tmpl = ` {{range $index, $link := .}} {{$index}}: <a href="{{$link.Href}}">{{$link.Name}}</a> {{end}} ` type Link struct { Name string Href string } func main() { // arrays var la [2]Link la[0] = Link{"Google", "https://www.google.com/"} la[1] = Link{"Facebook", "https://www.facebook.com/"} t, _ := template.New("foo").Parse(tmpl) t.Execute(os.Stdout, la) // slices var ls []Link ls = append(ls, Link{"Google", "https://www.google.com/"}) ls = append(ls, Link{"Facebook", "https://www.facebook.com/"}) t.Execute(os.Stdout, ls) }
Complete Python Jinja2 source code:
jinja2-example-3.py | repository | view raw#!/usr/bin/env python # -*- coding:utf-8 -*- from jinja2 import Template import sys tmpl = """ {% for link in links %} {{ loop.index0 }}: <a href="{{link.href}}">{{link.name}}</a> {{ loop.index }}: <a href="{{link.href}}">{{link.name}}</a> {% endfor %} """ if __name__ == '__main__': links = [ {'name': 'Google', 'href': 'https://www.google.com'}, {'name': 'Facebook', 'href': 'https://www.facebook.com'} ] t = Template(tmpl) sys.stdout.write(t.render(links=links))
Tested on: Ubuntu Linux 14.10, Go 1.4, Python 2.7.8, Jinja2 2.7.3
Golang html/template versus Python Jinja2 series:
[1]Golang html/template versus Python Jinja2 (1)[2]Golang html/template versus Python Jinja2 (2)[3]Golang html/template versus Python Jinja2 (3) - Arrays and Slices[4]Golang html/template versus Python Jinja2 (4) - Arrays and Slices Index[5]Golang html/template versus Python Jinja2 (5) - Maps and Dictionaries[6]Golang html/template versus Python Jinja2 (6) - Template Inheritance (Extends)[7]Golang html/template versus Python Jinja2 (7) - Custom Functions and FiltersReferences:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK