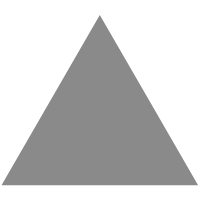
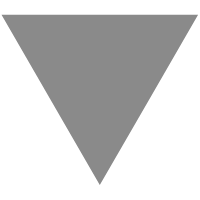
推荐20个JavaScript代码优化的技巧
source link: https://www.fly63.com/article/detial/11408
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
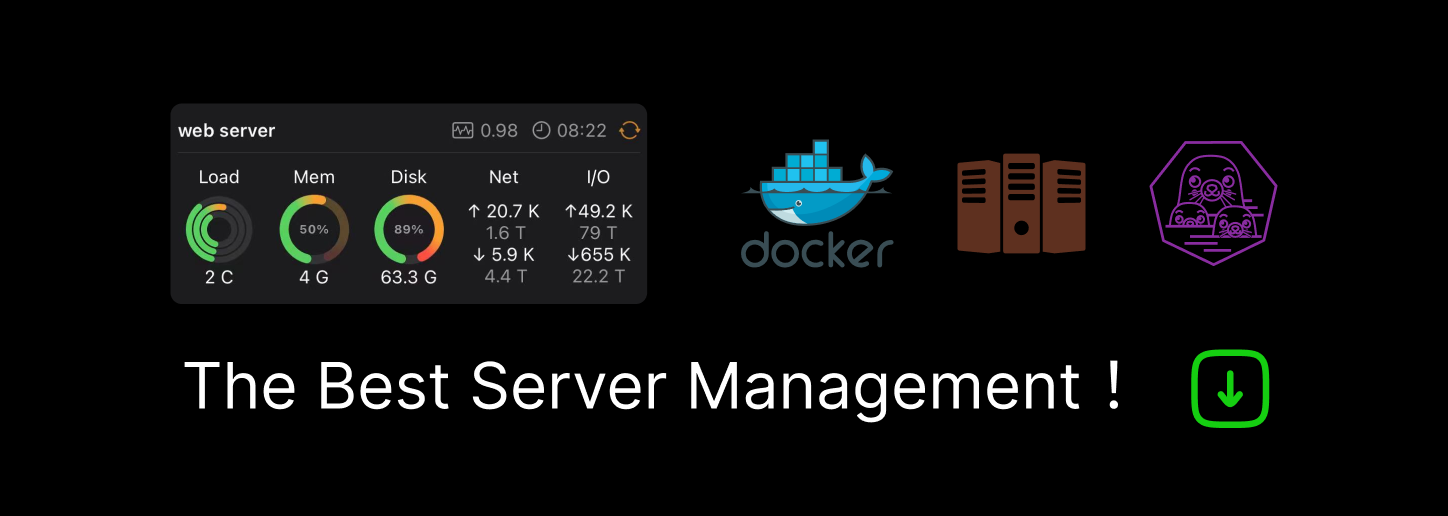
扫一扫分享
1. 多个条件的 if 语句, includes方法
我们可以在数组中存储多个值,并且可以使用数组的 includes 方法。
if (x === 'abc' || x === 'def' || x === 'ghi' || x ==='jkl') {
//logic
}
//shorthand
if (['abc', 'def', 'ghi', 'jkl'].includes(x)) {
//logic
}
2.If … else 的缩写法
当我们的 if-else 条件中的逻辑比较简单时,可以使用这种简洁的方式——三元条件运算符。
let test: boolean;if (x > 100) {
test = true;
} else {
test = false;
}
// Shorthand
let test = (x > 10) ? true : false;
//or we can use directly
let test = x > 10;console.log(test);
3.对 Null、Undefined、Empty 这些值的检查
我们创建一个新变量,有时候需要检查是否为 Null 或 Undefined。JavaScript 本身就有一种缩写法能实现这种功能。
if (test1 !== null || test1 !== undefined || test1 !== '') {
let test2 = test1;
}
// Shorthand
let test2 = test1 || '';
4.给多个变量赋值
我们可以使用数组解构来在一行中给多个变量赋值。
let a, b, c;
a = 5;
b = 8;
c = 12;
//Shorthand
let [a, b, c] = [5, 8, 12];
5.赋默认值
我们可以使用 OR(||) 短路运算来给一个变量赋默认值,如果预期值不正确的情况下。
//Longhand
let imagePath;
let path = getImagePath();
if(path !== null && path !== undefined && path !== '') {
imagePath = path;
} else {
imagePath = 'default.jpg';
}
//Shorthand
let imagePath = getImagePath() || 'default.jpg';
6.交换两个变量
为了交换两个变量,我们通常使用第三个变量。我们可以使用数组解构赋值来交换两个变量。
let x = 'Hello', y = 55;
//Longhand
const temp = x;
x = y;
y = temp;
//Shorthand
[x, y] = [y, x];
7.多行字符串
对于多行字符串,我们一般使用 + 运算符以及一个新行转义字符(\n)。我们可以使用 (`) 以一种更简单的方式实现。
//Longhand
console.log('JavaScript, often abbreviated as JS, is a\n' + 'programming language that conforms to the \n' +
'ECMAScript specification. JavaScript is high-level,\n' +
'often just-in-time compiled, and multi-paradigm.' );
//Shorthand
console.log(`JavaScript, often abbreviated as JS, is a programming language that conforms to the ECMA
8.For 循环
为了遍历一个数组,我们一般使用传统的for循环。我们可以使用for...of来遍历数组。为了获取每个值的索引,我们可以使用for...in循环。
let arr = [10, 20, 30, 40];
//Longhand
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
//Shorthand
//for of loop
for (const val of arr) {
console.log(val);
}
//for in loop
for (const index in arr) {
console.log(arr[index]);
}
我们还可以使用for...in循环来遍历对象属性。
let obj = {x: 20, y: 50};
for (const key in obj) {
console.log(obj[key]);
}
参考:JavaScript 中遍历对象和数组的不同方法 https://jscurious.com/different-ways-to-iterate-through-objects-and-arrays-in-javascript/
9.合并数组
let arr1 = [20,30];
//Longhand
let arr2 = arr1.concat([60,80]);
// [20, 30, 60, 80]
//Shorthand
let arr2 = [...arr1,60,80];
// [20, 30, 60, 80]
10.深拷贝多级对象
为了深拷贝一个多级对象,我们要遍历每一个属性并检查当前属性是否包含一个对象。如果当前属性包含一个对象,然后要将当前属性值作为参数递归调用相同的方法(例如,嵌套的对象)。
我们可以使用JSON.stringify()和JSON.parse(),如果我们的对象不包含函数、undefined、NaN 或日期值的话。
如果有一个单级对象,例如没有嵌套的对象,那么我们也可以使用扩展符来实现深拷贝。
let obj = {x: 20, y: {z: 30}};
//Longhand
const makeDeepClone = (obj) => {
let newObject = {};
Object.keys(obj).map(key => {
if(typeof obj[key] === 'object'){
newObject[key] = makeDeepClone(obj[key]);
} else {
newObject[key] = obj[key];
}
});
return newObject;
}
const cloneObj = makeDeepClone(obj);
//Shorthand
const cloneObj = JSON.parse(JSON.stringify(obj));
//Shorthand for single level object
let obj = {x: 20, y: 'hello'};
const cloneObj = {...obj};
11.重复一个字符串多次
为了重复一个字符串 N 次,你可以使用for循环。但是使用repeat()方法,我们可以一行代码就搞定。
//Longhand
let str = '';
for(let i = 0; i < 5; i ++) {
str += 'Hello ';
}
console.log(str); // Hello Hello Hello Hello Hello
// Shorthand
'Hello '.repeat(5);
提示: 想要给某人发 100 遍“sorry”来道歉吗?用 repeat() 方法试试吧。如果你想要每次在新的一行重复字符串,可以在字符串后面加一个 。
'sorry\n'.repeat(100);
12.双非位运算符 (~~)
双非位运算符是Math.floor()方法的缩写。
//Longhand
const floor = Math.floor(6.8); // 6
// Shorthand
const floor = ~~6.8; // 6
改进: 双非位运算符只对 32 位整数有效,例如 (2**31)-1 = 2147483647。所以对于任何大于 2147483647 的数字,双非位运算符 (~~) 都会给出错误的结果,这种情况下推荐使用 Math.floor() 方法。
13.找出数组中的最大和最小数字
我们可以使用 for 循环来遍历数组中的每一个值,然后找出最大或最小值。我们还可以使用 Array.reduce() 方法来找出数组中的最大和最小数字。
但是使用扩展符号,我们一行就可以实现。
// Shorthand
const arr = [2, 8, 15, 4];
Math.max(...arr); // 15
Math.min(...arr); // 2
14.指数幂
我们可以使用Math.pow()方法来得到一个数字的幂。有一种更短的语法来实现,即双星号 (**)。
//Longhand
const power = Math.pow(4, 3); // 64
// Shorthand
const power = 4**3; // 64
15.字符串转成数字
有一些内置的方法,例如parseInt和parseFloat可以用来将字符串转为数字。我们还可以简单地在字符串前提供一个一元运算符 (+) 来实现这一点。
//Longhand
let total = parseInt('453');
let average = parseFloat('42.6');
//Shorthand
let total = +'453';
let average = +'42.6';
16.数字分隔符
这是比较新的语法,ES2021 提出来的,数字字面量可以用下划线分割,提高了大数字的可读性:
// 旧语法
let number = 98234567
// 新语法
let number = 98_234_567
17.善用箭头函数简化 return
ES6 的箭头函数真是个好东西,当函数简单到只需要返回一个表达式时,用箭头函数最合适不过了,return都不用写:
Longhand:
//longhand
function getArea(diameter) {
return Math.PI * diameter
}
//shorthand
getArea = diameter => (
Math.PI * diameter;
)
18.简化 switch
这个技巧也很常用,把switch 转换成对象的key-value形式,代码简洁多了:
// Longhand
switch (data) {
case 1:
test1();
break;
case 2:
test2();
break;
case 3:
test();
break;
// And so on...
}
// Shorthand
var data = {
1: test1,
2: test2,
3: test
};
data[anything] && data[anything]();
19.转换Boolean型
常规的boolean型值只有 true 和 false,但是在JavaScript中我们可以将其他的值认为是 ‘truthy’ 或者 ‘falsy’的。 除了0, “”, null, undefined, NaN 和 false,其他的我们都可以认为是‘truthy’的。 我们可以通过负运算符!将一系列的变量转换成“boolean”型。
const isTrue = !0;
const isFalse = !1;
const alsoFalse = !!0;
console.log(isTrue); // Result: true
console.log(typeof true); // Result: "boolean"
20.Array.find 缩写法
当我们需要在一个对象数组中按属性值查找特定对象时,find 方法很有用。
const data = [{
type: 'test1',
name: 'abc'
},
{
type: 'test2',
name: 'cde'
},
{
type: 'test1',
name: 'fgh'
},
]function findtest1(name) {
for (let i = 0; i < data.length; ++i) {
if (data[i].type === 'test1' && data[i].name === name) {
return data[i];
}
}
}
//Shorthand
filteredData = data.find(data => data.type === 'test1' && data.name === 'fgh');
console.log(filteredData); // { type: 'test1', name: 'fgh' }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK