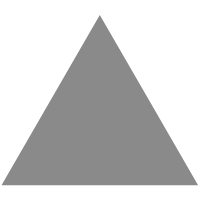
3
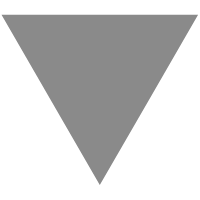
PHP 系列 (三)
source link: https://sevming.github.io/PHP/php-series-3.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
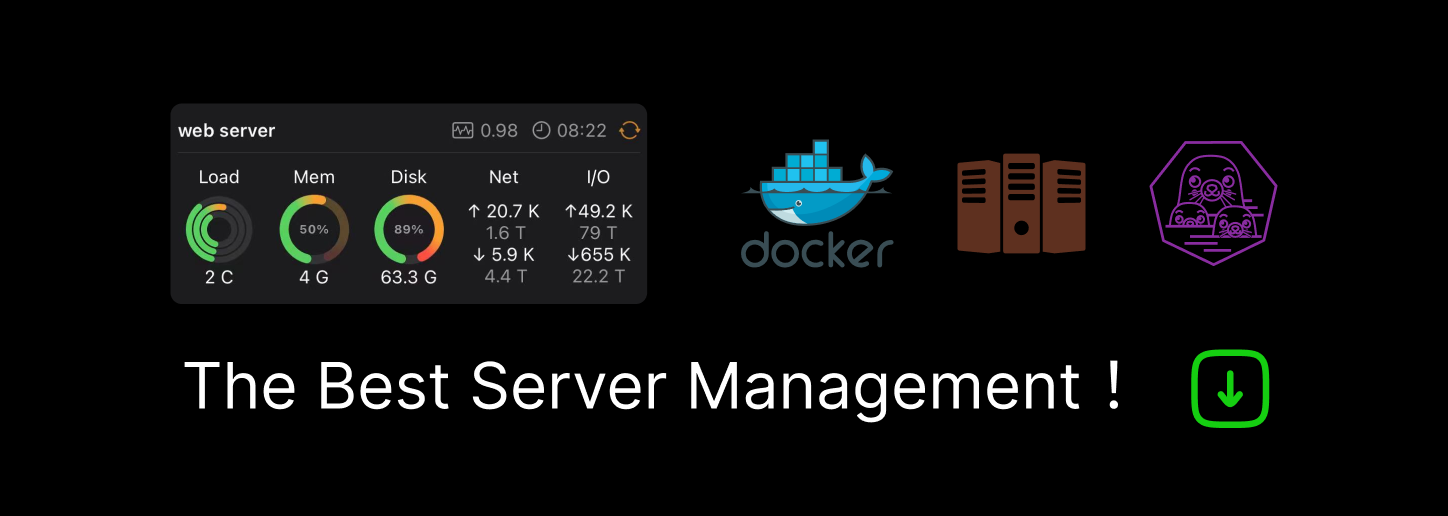
PHP 系列 (三)
cURL 模拟登录
<?php
$data = [
'email' => '[email protected]',
'password' => '123456',
];
// 模拟登录
$cookie = __DIR__ . '/cookie.txt';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// 连接结束后,保存 cookie 信息的文件
curl_setopt($ch, CURLOPT_COOKIEJAR, $cookie);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
$result = curl_exec($ch);
// 采集数据
$url = 'http://xxx.com/login';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// 包含 cookie 数据的文件
curl_setopt($ch, CURLOPT_COOKIEFILE, $cookie);
$result = curl_exec($ch);
$result = json_decode($result, true, JSON_UNESCAPED_UNICODE);
var_dump($result);
通过 SSH 拷贝远程文件至本地
<?php
class SSH2
{
/**
* @var resource
*/
private $ssh;
/**
* SSH2 constructor.
*
* @param string $host
* @param int $port
* @param string $username
* @param string $password
*/
public function __construct(string $host, int $port, string $username, string $password)
{
$this->ssh = ssh2_connect($host, $port);
ssh2_auth_password($this->ssh, $username, $password);
}
/**
* Exec.
*
* @param string $command
*
* @return bool|string
*/
public function exec(string $command)
{
$stream = ssh2_exec($this->ssh, $command);
stream_set_blocking($stream, true);
return stream_get_contents($stream);
}
/**
* Scp send.
*
* @param string $local
* @param string $remote
* @param int|null $mode
*
* @return bool
*/
public function scpSend(string $local, string $remote, ?int $mode = null)
{
return ssh2_scp_send($this->ssh, $local, $remote, $mode);
}
/**
* Scp recv.
*
* @param string $remote
* @param string $local
*
* @return bool
*/
public function scpRecv(string $remote, string $local)
{
return ssh2_scp_recv($this->ssh, $remote, $local);
}
}
try {
$ip = '192.168.0.77';
$username = 'root';
$password = 'root';
$ssh2 = new SSH2($ip, 22, $username, $password);
// 打包远程文件
$ssh2->exec('cd /data/backup && rm -rf backup.zip && zip -r backup.zip ./');
// 拷贝远程文件至本地
$ssh2->scpRecv('/data/backup/backup.zip', 'D:\backup\backup.zip');
} catch (Exception $e) {
exit($e->getMessage());
}
cURL 代理 (GuzzleHttp)
<?php
require_once 'vendor/autoload.php';
try {
// curl --proxy 127.0.0.1:58591 https://www.google.com/
$url = 'https://www.google.com/';
$client = new GuzzleHttp\Client([
'timeout' => 3
]);
$response = $client->get($url, [
'curl' => [
CURLOPT_SSL_VERIFYPEER => 0,
CURLOPT_SSL_VERIFYHOST => 0,
CURLOPT_PROXY => '127.0.0.1',
CURLOPT_PROXYPORT => '58591',
]
]);
var_dump(json_decode($response->getBody()->getContents(), true));
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_TIMEOUT, 3);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($ch, CURLOPT_PROXY, '127.0.0.1');
curl_setopt($ch, CURLOPT_PROXYPORT, '58591');
$result = curl_exec($ch);
curl_close($ch);
var_dump(json_decode($result, true));
} catch (Throwable $t) {
exit($t->getMessage());
}
合并多维数组
<?php
/**
* 需求:处理多维数组,并以一维数组的显示如下结果
* A 品牌 => 一组
* B 品牌 => 一组
* ...
* E 品牌 => 二组
* ...
*/
$brandArray = [];
$brandGroups = [
'一组' => [
['A 品牌'],
[
'B 品牌',
'C 品牌',
],
['D 品牌'],
],
'二组' => [
[
'E 品牌',
'F 品牌',
],
['G 品牌'],
]
];
// 第一种:foreach - 数组有几个层级,就得遍历几次
foreach ($brandGroups as $groupName => $groups) {
foreach ($groups as $brands) {
$brandArray = array_merge($brandArray, array_fill_keys($brands, $groupName));
}
}
// 第二种:RecursiveIteratorIterator
foreach ($brandGroups as $groupName => $groups) {
$it = new \RecursiveIteratorIterator(new \RecursiveArrayIterator($groups));
$brandArray = array_merge($brandArray, array_fill_keys(iterator_to_array($it, false), $groupName));
}
// 第三种:array_walk_recursive
foreach ($brandGroups as $groupName => $groups) {
$brands = [];
array_walk_recursive($groups, function ($arr) use (&$brands) {
$brands[] = $arr;
});
$brandArray = array_merge($brandArray, array_fill_keys($brands, $groupName));
}
dd($brandArray);
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK