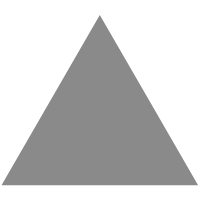
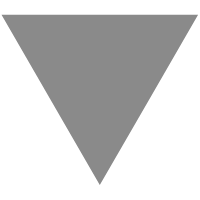
Draggable Toggleable Simple Web Keyboard
source link: http://siongui.github.io/2015/02/16/draggable-toggleable-simple-web-keyboard/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Draggable Toggleable Simple Web Keyboard
February 16, 2015
First we create a simple 0-9 kaypad:
keyboard.html | repository | view raw
<!doctype html> <html> <head> <meta charset="utf-8"> <title>Simple Keypad Example</title> </head> <body> <input id="PaliInput" type="text" name="word" value=""> <div id="keyboard" style="position: absolute; border: 1px solid #ccc; padding: 1em;"> <input name="7" type="button" value="7" onclick="numClicked(this)"/> <input name="8" type="button" value="8" onclick="numClicked(this)"/> <input name="9" type="button" value="9" onclick="numClicked(this)"/> <br> <input name="4" type="button" value="4" onclick="numClicked(this)"/> <input name="5" type="button" value="5" onclick="numClicked(this)"/> <input name="6" type="button" value="6" onclick="numClicked(this)"/> <br> <input name="1" type="button" value="1" onclick="numClicked(this)"/> <input name="2" type="button" value="2" onclick="numClicked(this)"/> <input name="3" type="button" value="3" onclick="numClicked(this)"/> </div> <script type="text/javascript"> function numClicked(elm) { document.getElementById("PaliInput").value += elm.value; document.getElementById("PaliInput").focus(); } </script> </body> </html>
Next, we make the kaypad draggable via jQuery and toggleable via vanilla JavaScript:
draggable-toggleable-keyboard.html | repository | view raw
<!doctype html> <html> <head> <meta charset="utf-8"> <title>Simple Draggable Toggleable Keypad Example</title> </head> <body> <input id="PaliInput" type="text" name="word" value=""> <span style="cursor: pointer; color: blue;" onclick="toggle(this);">Hide Keypad</span> <div id="keyboard" style="position: absolute; border: 1px solid #ccc; padding: 1em; cursor: move;"> <input name="7" type="button" value="7" onclick="numClicked(this)"/> <input name="8" type="button" value="8" onclick="numClicked(this)"/> <input name="9" type="button" value="9" onclick="numClicked(this)"/> <br> <input name="4" type="button" value="4" onclick="numClicked(this)"/> <input name="5" type="button" value="5" onclick="numClicked(this)"/> <input name="6" type="button" value="6" onclick="numClicked(this)"/> <br> <input name="1" type="button" value="1" onclick="numClicked(this)"/> <input name="2" type="button" value="2" onclick="numClicked(this)"/> <input name="3" type="button" value="3" onclick="numClicked(this)"/> </div> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script> <script src="https://ajax.googleapis.com/ajax/libs/jqueryui/1.11.2/jquery-ui.min.js"></script> <script type="text/javascript"> $(function() { $( "#keyboard" ).draggable(); }); function numClicked(elm) { document.getElementById("PaliInput").value += elm.value; document.getElementById("PaliInput").focus(); } function toggle(elm) { var kb = document.getElementById("keyboard"); if (kb.style.display == "none") { kb.style.display = "block"; elm.innerHTML = "Hide Keypad"; } else { kb.style.display = "none"; elm.innerHTML = "Show Keypad"; } } </script> </body> </html>
For a full-featured keyboard, please check the references.
References:
[3]How Can I Implement A Simple Virtual Keyboard ?
[4]Creating a Virtual jQuery Keyboard
Other links I found:
[5]On-screen HTML/Javascript keyboard
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK