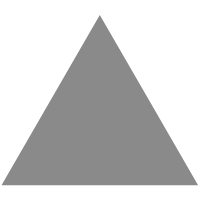
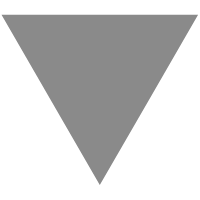
[Golang] Compile SASS/SCSS files to CSS via libsass
source link: http://siongui.github.io/2016/01/28/go-compile-sass-scss/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
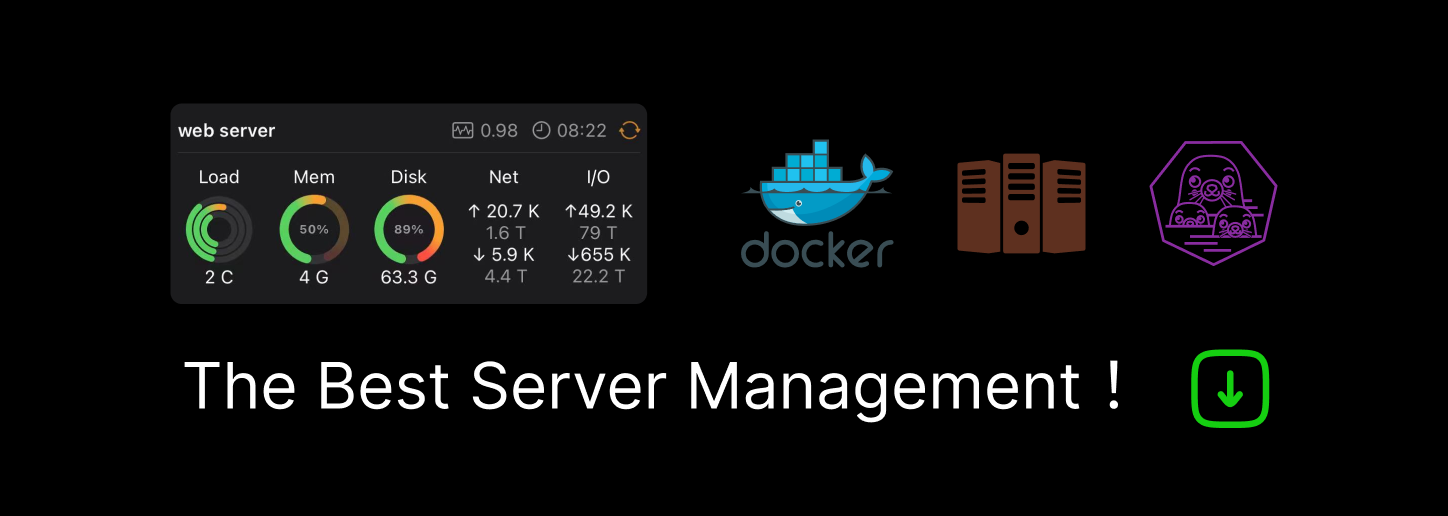
[Golang] Compile SASS/SCSS files to CSS via libsass
January 28, 2016
Write a Golang program to compile your SASS/SCSS (CSS extension language) files to CSS via libsass and Go wrapper for libsass.
Download Makefile for demo (remember to modify GOROOT and GOPATH in Makefile):
Makefile | repository | view raw
# cannot use relative path in GOROOT, otherwise 6g not found. For example, # export GOROOT=../go (=> 6g not found) # it is also not allowed to use relative path in GOPATH export GOROOT=$(realpath ../go) export GOPATH=$(realpath .) export PATH := $(GOROOT)/bin:$(GOPATH)/bin:$(PATH) FILE=testsass SCSSDIR=src/style default: @go run $(FILE).go fmt: @go fmt $(FILE).go download: @[ -d $(SCSSDIR) ] || mkdir -p $(SCSSDIR) @[ -e $(SCSSDIR)/_normalize302.scss ] || wget -O $(SCSSDIR)/_normalize302.scss http://necolas.github.com/normalize.css/3.0.2/normalize.css @[ -e $(SCSSDIR)/_css4rst.scss ] || wget -O $(SCSSDIR)/_css4rst.scss https://github.com/siongui/userpages/raw/master/theme/styling/_css4rst.scss @[ -e $(SCSSDIR)/_pygments.scss ] || wget -O $(SCSSDIR)/_pygments.scss https://github.com/siongui/userpages/raw/master/theme/styling/_pygments.scss @[ -e $(SCSSDIR)/style.scss ] || wget -O $(SCSSDIR)/style.scss https://github.com/siongui/userpages/raw/master/theme/styling/style.scss install: go get -u github.com/wellington/go-libsass
Install go-libsass (You do not need to install libsass):
# cd to the directory where you put Makefile $ make install
Download demo SCSS files:
# cd to the directory where you put Makefile $ make download
Now we write a Go program to compile SCSS files to CSS (Put this Go file in the same directory as Makefile):
testsass.go | repository | view raw
package main import ( libsass "github.com/wellington/go-libsass" "os" ) func main() { const sassdir = "src/style/" // open input sass/scss file to be compiled fi, err := os.Open(sassdir + "style.scss") if err != nil { panic(err) } defer fi.Close() // create output css file fo, err := os.Create("style.css") if err != nil { panic(err) } defer fo.Close() // options for compilation p := libsass.IncludePaths([]string{sassdir}) s := libsass.OutputStyle(libsass.COMPRESSED_STYLE) // create a new compiler with options comp, err := libsass.New(fo, fi, p, s) if err != nil { panic(err) } // start compile if err := comp.Run(); err != nil { panic(err) } }
Run the Go program:
# cd to the directory where you put Makefile $ make
The output file will be style.css in the same directory.
Tested on: Ubuntu Linux 15.10, Go 1.5.3.
Reference:
[1]wellington/go-libsass: Go wrapper for libsass, the only Sass 3.3 compiler for Go
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK