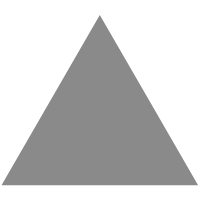
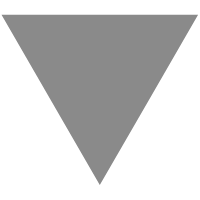
Laravel DynamoDB Eloquent Models and Query Builder
source link: https://laravel-news.com/laravel-dynamodb
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
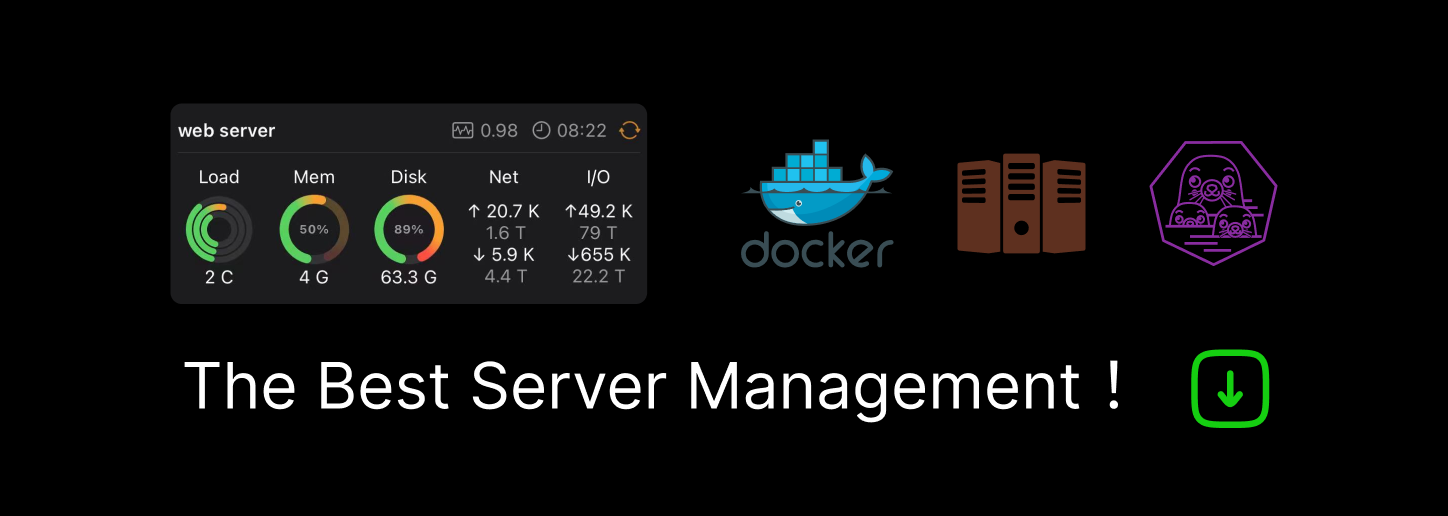
Laravel DynamoDB Eloquent Models and Query Builder
Laravel DynamoDB is a DynamoDB-based Eloquent model and Query builder for Laravel. Using the provided Dynamo driver, models extend the Eloquent base model:
1use Kitar\Dynamodb\Model\Model;
3class ProductCatalog extends Model
5 // Required
6 protected $table = 'ProductCatalog';
8 // Name of the partition key (required)
9 protected $primaryKey = 'Id';
11 // Name of the sort key (optional)
12 protected $sortKey = 'Subject';
14 // Default sort key value when we
15 // call find without a sort key.
16 protected $sortKeyDefault = 'profile';
18 protected $fillable = ['Id', 'Price', 'Title'];
Here are a few examples from the readme of queries and operations you can expect using this package:
1// Get all models
2$products = ProductCatalog::scan();
3// or
4$products = ProductCatalog::all();
6// Paginated
7$products = ProductCatalog::limit(5)->scan();
9// Creating a user
10$user = User::create([
11 'email' => '[email protected]',
12 // Sort key. If we don't specify this,
13 // sortKeyDefault will be used.
14 'type' => 'profile',
15]);
17// Instantiate a model and then save.
18$user = new User([
19 'email' => '[email protected]',
20 'type' => 'profile'
21]);
23$user->save();
25// Update an existing model
26$user->update([
27 'name' => 'foobar'
28]);
This package includes more advanced usage of Dynamo, including a query builder that you can use without models (and outside of Laravel too). Also, the query builder supports things such as Condition Expressions. For example:
1DB::table('ProductCatalog')
2 ->condition('Id', 'attribute_not_exists')
3 ->orCondition('Price', 'attribute_not_exists')
4 ->putItem([/*...*/]);
Here's an example of filter expressions, which can filter results with expressions before results are returned from the database:
1$response = DB::table('Thread')
2 ->filter('LastPostedBy', '=', 'User A')
3 ->scan();
5// orFilter()
6$response = DB::table('Thread')
7 ->filter('LastPostedBy', '=', 'User A')
8 ->orFilter('LastPostedBy', '=', 'User B')
9 ->scan();
11// filterIn
12$response = DB::table('Thread')
13 ->filterIn('LastPostedBy', ['User A', 'User B'])
14 ->scan();
16// filterBetween
17$response = DB::table('ProductCatalog')
18 ->filterBetween('Price', [0, 100])
19 ->scan();
The Laravel DynamoDB package also has detailed instructions on using a DynamoDB model for user authentication. You can learn more about this package, get full installation instructions, and view the source code on GitHub.
This package was submitted to our Laravel News Links section. Links is a place the community can post packages and tutorials around the Laravel ecosystem. Follow along on Twitter @LaravelLinks
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK