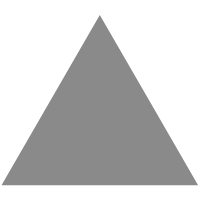
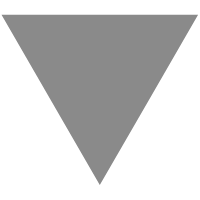
为 Vue3 添加一个简单的 Store
source link: https://blog.ixk.me/post/add-simple-store-for-vue3
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
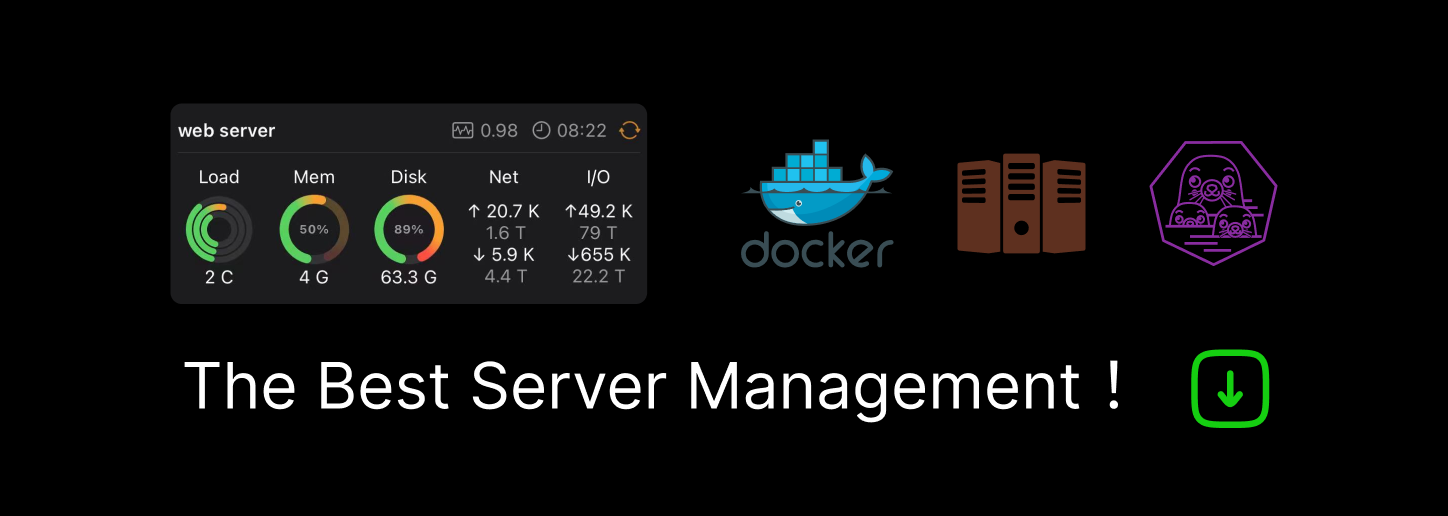
为 Vue3 添加一个简单的 Store
Vue 3.0 挺早就已经发布了 Beta 版本,一直没有机会尝试下。主要是最近在折腾后端,前端几乎没再碰过。现在 XK-Java 已经大致写完了,最近应该也不会添加太多的功能了,XK-PHP 也打算等到 Swoole 支持 PHP8 的时候重构一波,所以比较闲,便打算翻出以前的项目(XK-Note,XK-Editor)重构(重写)一下。
由于 Vue 2.x 对 TypeScript 的支持性不好,所以便打算直接使用 Vue 3.0,顺便体验一把函数化组件。因为 XK-Editor 需要使用 Store 来管理状态,而之前的并不太适合 Vue 3.0,于是便有了这篇文章。
首先放下效果:
1<template> 2 <div id="app"> 3 <img alt="Vue logo" src="./assets/logo.png" /> 4 <div> 5 {{ count }} 6 <button @click="addCount">+</button> 7 </div> 8 <div> 9 {{ subCount }} 10 <button @click="addSubCount">+</button> 11 </div> 12 <div>{{ arr1 }}</div> 13 </div> 14</template> 15 16<script lang="ts"> 17 import { defineComponent } from "vue"; 18 import { useAction, useState } from "@/store"; 19 20 export default defineComponent({ 21 name: "App", 22 setup() { 23 const count = useState<number>("count"); 24 const addCount = useAction<Function>("addCount"); 25 const subCount = useState<number>("sub.count"); 26 const addSubCount = useAction("sub.addSubCount"); 27 const arr1 = useState<string>("arr.0"); 28 return { count, addCount, subCount, addSubCount, arr1 }; 29 } 30 }); 31</script>
可以看到风格非常像 React Hook,而且使用起来也很方便,可以通过像 Laravel 的 data_get
一样的点语法来获取到嵌套的 state
,同时由于数据是响应式的,同时也可以直接设置值,而不需要另外增加一个 set
方法来设置。当然也不一定需要使用点语法,你也可以像之前的 Vuex 2 的 mapState
一样使用函数的方式获取 state
。支持泛型,可以很好的保持 TypeScript 的特性和优点。
Store 相关的分析这里就不说明了,本文只介绍 Store 用到的 Vue 3.0 新增的特性。
首先就是最重要的 reactive
函数,这是在 Vue 3 新增的函数,用来将普通的数据转换成响应式数据,替代了 Vue 2.x 中的 Vue.observable
。
然后就是 computed
函数,computed
函数对应着 Vue 2.x 的计算属性,由于 Vue 3 不再是 Vue 2.x 的 Options API(虽然依旧可以用)而是采用了和 React Hook 类似的 Composition API,这意味着,我们可以在任何的地方使用 Composition API,而不再局限于组件内。之所以要用到这个函数的原因是外部的响应式数据无法在组件中直接使用,会导致不更新的情况发生,所以需要一层计算属性来解决这种问题,在 Vue 2.x 也存在这个问题。
首先我们先创建 State 的部分:
1import { reactive } from "vue"; 2 3// 使用 reactive 函数完成响应式转换 4const state = reactive({ 5 count: 1, 6 sub: { 7 count: 2 8 }, 9 arr: ["arr1", "arr2"] 10}); 11 12// 由于使用了 TypeScript,光有数据还不行,还需要有类型,利用 typeof 可以很方便的获取对象的类型 13export type State = typeof state; 14export default state;
然后是 Actions:
1import state from "@/store/state"; 2 3const actions = { 4 addCount() { 5 state.count++; 6 }, 7 sub: { 8 addSubCount() { 9 state.sub.count++; 10 } 11 } 12}; 13 14// 同样需要导出类型 15export type Actions = typeof actions; 16export default actions;
接着就是最主要的部分了:
1import state, { State } from "@/store/state"; 2import actions, { Actions } from "@/store/actions"; 3import { WritableComputedRef } from "@vue/reactivity"; 4import { computed } from "vue"; 5 6// 函数的方式获取值的参数类型 7type useStateGetterFn = <R>(state: State) => R; 8// 函数的方式获取值和设置值的参数类型 9type useStateWritableFn<R> = { 10 get: (state: State, ctx?: any) => R; 11 set: (state: State, value: R) => void; 12}; 13// 函数的方式获取 Action 的参数类型 14type useActionFn = (actions: Actions) => any; 15 16// 获取 Store 17export const useStore = (): { state: State; actions: Actions } => { 18 return { state, actions }; 19}; 20 21// 获取 State 22export function useState<R>( 23 key: string | useStateGetterFn | useStateWritableFn<R> | null = null 24): WritableComputedRef<R> { 25 // 如果未传参代表获取全部的 state 26 if (key === null) { 27 // 将 state 包装成计算属性 28 return computed(() => (state as any) as R); 29 } 30 // 函数方式获取 const count = useState(() => state.count) 31 if (typeof key === "function") { 32 // 将 state 传入函数,然后将函数返回值包装成计算属性 33 return computed(() => key(state)); 34 } 35 // 字符串或点语法方式获取和设置 36 if (typeof key === "string") { 37 let result = state as any; 38 const keys = key.split("."); 39 const lastKey = keys.pop() as string; 40 for (const k of keys) { 41 result = result[k]; 42 // 如果 result 为 null 了说明 key 设置错误,抛出异常 43 if (result === null || result === undefined) { 44 throw Error(`key is error [${key}]`); 45 } 46 } 47 // 包装 set 和 get 48 return computed({ 49 get: (ctx) => result[lastKey], 50 set: (v) => (result[lastKey] = v) 51 }); 52 } 53 // 函数的方式,传入了 set 和 get,则将其包装成带 set 的计算属性 54 return computed({ 55 get: (ctx) => key.get(state, ctx), 56 set: (v) => key.set(state, v) 57 }); 58} 59 60// 获取 Action,类似于 useState 61export function useAction<A>(key: string | useActionFn | null = null): A { 62 if (key === null) { 63 return (actions as any) as A; 64 } 65 if (typeof key === "string") { 66 let result = actions as any; 67 const keys = key.split("."); 68 for (const k of keys) { 69 result = result[k]; 70 if (result === null || result === undefined) { 71 throw Error(`key is error [${key}]`); 72 } 73 } 74 return result; 75 } 76 return key(actions); 77}
由于只简单弄了一下,并没有添加 debug
的部分,由于 Vue 不像 React 一样使用不可变数据,所以无法简单的获取旧值,必须要深克隆一份才能保证旧值不随新值变化,这里就不弄了(逃。
使用的方式上面已经展示过了,这里就不展示了。
Vue 3.0 添加了 Composition API 后编码就不会再像 Vue 2.x 那样为了一个方法满屏幕找的情况了,各种逻辑都可以集中在一个区域里,同时也和 React Hook 一样,可以很方便的利用各种 use
函数扩展功能。响应式也改成用 Proxy
,应该不会再遇到各种丢更新的情况了。
Vue 3 香,不过我还是更喜欢 React(逃
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK