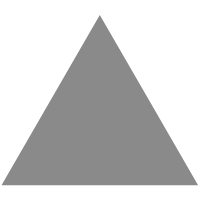
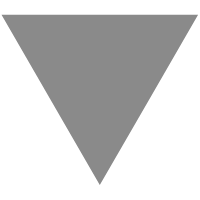
Instancio: Random Test Data Generator for Java - DZone Java
source link: https://dzone.com/articles/instancio-random-test-data-generator-for-java
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
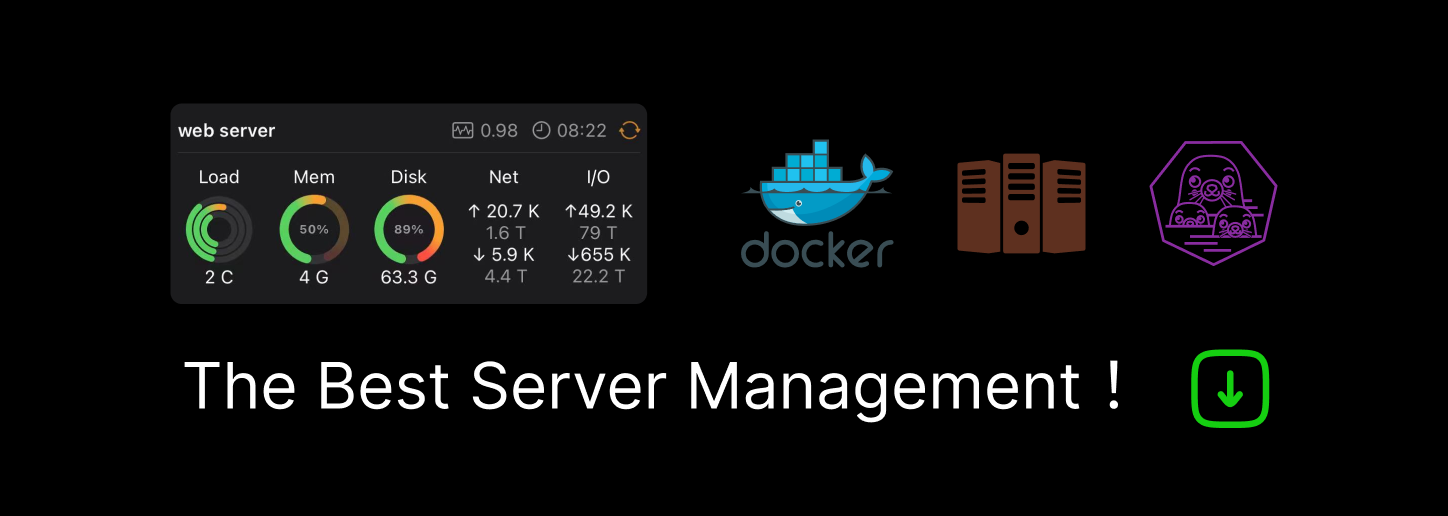
Instancio: Random Test Data Generator for Java (Part 1)
More testing, less plumbing
Join the DZone community and get the full member experience.
Join For FreeBy now hopefully all of us, developers, have embraced unit testing. Good test coverage of our codebase, combined with that green checkmark after running the tests, gives us a warm fuzzy feeling that everything is in order. While we all like that warm fuzzy feeling, writing tests usually requires some plumbing. This is the "boring part", where you create your test objects and manually set them up with dummy data. Oftentimes the values themselves don't even matter. A typical example of this is testing "converter" logic. For example, we might be converting a JPA entity to a DTO, or a JAXB class to something else. Such a test might look something like this:
@Test
void verifyCustomer() {
Customer customer = new Customer();
customer.setFirstName("John");
customer.setLastName("Doe");
customer.setAddress(new Address("123 Main St", "Seattle", "US"));
customer.setPhoneNumbers(List.of(
new Phone(PhoneType.HOME, "+1", "123-44-55"),
new Phone(PhoneType.WORK, "+1", "555-77-88")));
// ... etc
CustomerDTO customerDto = customerMapper.toDto(customer);
assertThat(customerDto.getFirstName()).isEqualTo(customer.getFirstName());
assertThat(customerDto.getLastName()).isEqualTo(customer.getLastName());
// ... etc
}
Writing setup code like above is tedious, especially when you have a class with a lot of properties and relationships. Some projects will have "fixture" classes with static helper methods for creating test objects, but that's still extra code that needs to be maintained. A better alternative is to delegate this task to a library.
Automate the "Boring Part"
Instancio is a library that can help automate the "boring part" by generating test data with a single method call (or a few method calls if you need to customize the data). Using Instancio, the above test will look like this:
@Test
void verifyCustomer() {
Customer customer = Instancio.create(Customer.class);
CustomerDTO customerDto = customerMapper.toDto(customer);
assertThat(customerDto.getFirstName()).isEqualTo(customer.getFirstName());
assertThat(customerDto.getLastName()).isEqualTo(customer.getLastName());
// ... etc
}
Instancio.create(Customer.class)
will generate a fully-populated Customer instance, including nested objects and collections, like address and the list of phone numbers. By default, Instancio will generate non-null values and non-empty collections and arrays. It's pretty easy to customize generated values. For example, the following snippet will create a Customer with 3 phone numbers with the given pattern:
Customer customer = Instancio.of(Customer.class)
.generate(field("phoneNumbers"), gen -> gen.collection().size(3))
.generate(field(Phone.class, "number"), gen -> gen.text().pattern("#d#d#d-#d#d-#d#d"))
.create();
The gen
variable provides access to built-in generators for customizing generated values. Some of the things you can do:
gen.string().allowEmpty().minLength(10) // string of at least 10 chars, allow empty strings
gen.text().pattern("#C#C-#d#d") //string with two uppercase and two digits, e.g. "AB-12"
gen.localDate().past() // past LocalDate
gen.arrays().length(5) // array of length 5
gen.ints().range(1, 10) // integer within given range
In addition to the generate()
method, Instancio has a supply()
method in case you want to set non-random values. For example, if you want a customer with several phone numbers, all with country code "+1":
Customer customer = Instancio.of(Customer.class)
.generate(field("phoneNumbers"), gen -> gen.collection().minSize(3))
.supply(field(Phone.class, "countryCode"), () -> "+1")
.create();
In addition to creating a single object, you can also create a java.util.stream.Stream
of objects. The Instancio.stream()
method creates an infinite stream of distinct, fully populated instances. For example, the following will create a map of 5 customers with id
as the map key:
Map<UUID, Customer> customerMap = Instancio.stream(Customer.class)
.limit(5)
.collect(Collectors.toMap(Customer::getId, Function.identity()));
Objects created using the stream API can be customized exactly the same way as single objects:
Map<UUID, Customer> customerMap = Instancio.of(Customer.class)
.generate(all(LocalDateTime.class), gen -> gen.localDateTime().past())
.supply(field(Phone.class, "countryCode"), () -> "+1")
.stream()
.limit(5)
.collect(Collectors.toMap(Customer::getId, Function.identity()));
There's a lot more you can do with Instancio, including creating instances of generic classes, creating models that act as a cookie cutter template for generating objects, JUnit 5 integration, and a metamodel generator that can automatically generate metamodels so that you don't have to reference fields by names. More on this will be covered in Part 2 (or you can check out the documentation). The best way is to try it out for yourself. Instancio is available from Maven central.
The standalone library:
<dependency>
<groupId>org.instancio</groupId>
<artifactId>instancio-core</artifactId>
<version>1.1.10</version>
<scope>test</scope>
</dependency>
Or if you use JUnit 5, instancio-junit
provides a JUnit 5 integration, including InstancioExtension
, an arguments provider for use with @ParameterizedTest
, and more:
<dependency>
<groupId>org.instancio</groupId>
<artifactId>instancio-junit</artifactId>
<version>1.1.10</version>
<scope>test</scope>
</dependency>
Project home on Github: https://github.com/instancio/instancio
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK