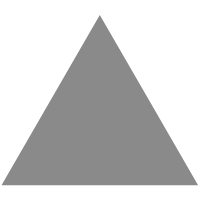
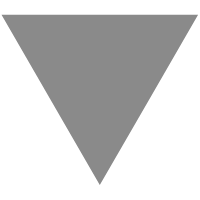
5 Best Python Tricks You Should Know in 2022
source link: https://www.journaldev.com/61283/beginner-python-tricks
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
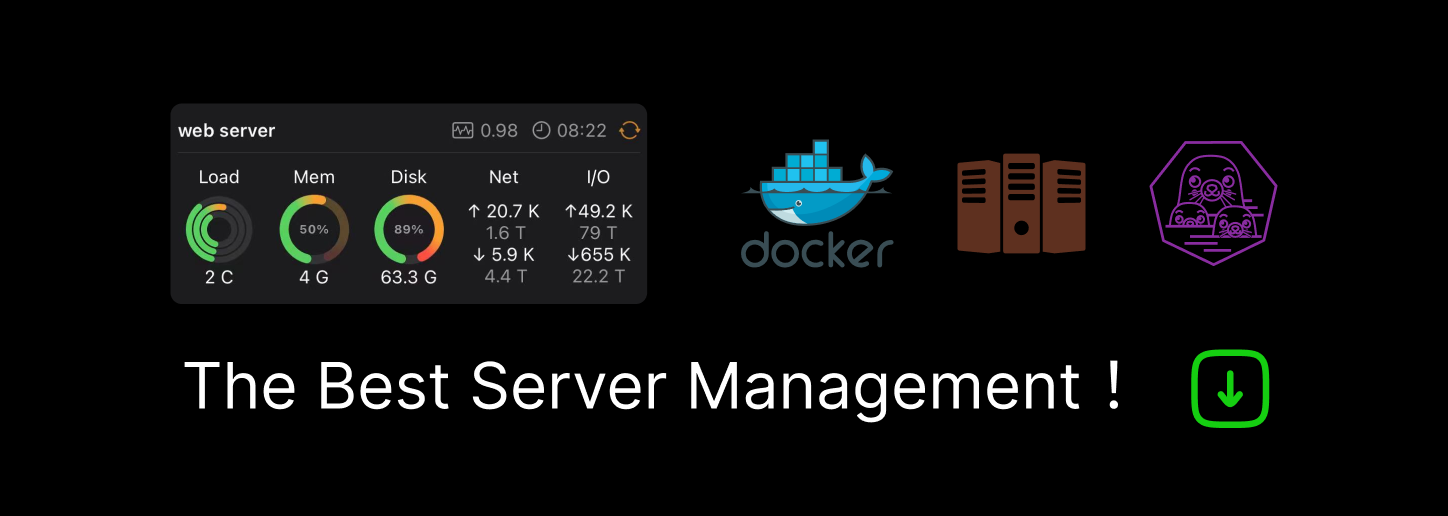
Python is Awesome! Most of us will use Python for data-centric tasks such as analysis, manipulation, and much more. But, Python always offered more than what we have expected. Today, in this article, let’s shed some light on stunning Python tricks which will save your time and energy.
1. Frequency in Python
We often use loops to print the count of each element in the list or so. But, we can also use the counter from the collections module to print out the count of each element in the input data.
Let’s see how it works.
For this you have to import collections
module offered by python.
#frequency
#Import collections module
import
collections
#Derive a list
my_list
=
[
1
,
1
,
2
,
2
,
3
,
3
,
3
,
3
,
4
,
5
,
5
,
6
,
6
,
6
,
7
,
8
,
9
,
9
,
10
,
10
,
10
]
#Call the Counter
freq
=
collections.Counter(my_list)
#print the frequency
print
(
'Count of each element:'
,freq)
Output –
Count of each element: Counter({3: 4, 6: 3, 10: 3, 1: 2, 2: 2, 5: 2, 9: 2, 4: 1, 7: 1, 8: 1})
I hope you will find this helpful.
2. String Formatting
You might have used format()
function in python to format a string. But, do you know without using those functions and crazy methods, you can easily format a string?
If you don’t know, keep reading…
#string formatting - An conversational example
#Assign the values to variables
first
=
'Sheron'
second
=
'Party'
#Call the variables
new
=
f
"Good day {first}. Thanks for your invite to the {second}"
#output
print
(
'Conversation:'
, new)
Output –
Conversation: Good day Sheron. Thanks for your invite to the Party
3. List Iteration
Commonly, we use loops to iterate over the list and print out the elements in it. But, we will see how we can quickly iterate over multiple lists and print out the elements in them.
#iterate over multiple lists
#define the lists
list_1
=
[
1
,
2
,
3
,
4
,
5
]
list_2
=
[
'A'
,
'B'
,
'C'
,
'D'
,
'E'
]
#use the for loop with Zip
for
a,b
in
zip
(list_1,list_2):
print
(a,b)
Output –
1 A
2 B
3 C
4 D
5 E
I have already mentioned that we can iterate over multiple lists. But, can we add one more list to this?
The answer is a big NO. This method can take 2 lists at a time. Not more than that :P. I have attached an error message I got with three lists for your reference.
#iterate over multiple lists
#define the lists
list_1
=
[
1
,
2
,
3
,
4
,
5
]
list_2
=
[
'A'
,
'B'
,
'C'
,
'D'
,
'E'
]
list_3
=
[
'Emily'
,
'Joe'
,
'Raman'
,
'Rav'
,
'Leonard'
]
for
a,b
in
zip
(list_1,list_2,list_3):
print
(a,b)
Output –

Note: Make sure you pass only a couple of lists for this method to work without any errors.
4. Read File with Base Python
I know that using pandas is the easiest way to read and write a CSV file. But, you should also be aware of this method, where we use base python to read and write CSV files.
#Read csv files without pandas
#import csv module
import
csv
#call the csv module with reader function
with
open
(
'Housing.csv'
,
'r'
)as
file
:
r
=
csv.reader(
file
)
for
row
in
r:
print
(row)
Output –

This will only help you when you are angry on Pandas :P.
5. Regular Expression (Regex)
Regular expressions are the sequence of patterns that are used to extract patterns in data. You can also use this for matching and searching patterns. Awesome right!
Let’s see Regex in action.
#regular expression
#import regex module
import
re
#input data
demo
=
"jhk1pi2yt3wx4x5iss6's7unaj8;ss9jsh0sjsss,susiw.su"
'''Use the regex to extarct numbers out
Input data.
To extract the numbers you can use [0-9] pattern'''
r
=
re.findall(
"[0-9]"
, demo)
#print the output
print
("".join(r))
Output –
1234567890
This is the magic of Regex.
Python Tricks – Conclusion
Python is vast and offers infinite possibilities in multiple domains. There are many python tricks of which we are not aware. In this article, I tried to share some of the key python tricks which you will find useful in your daily work using python. That’s all for now. Happy Python!!!
More read: Regex in python
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK