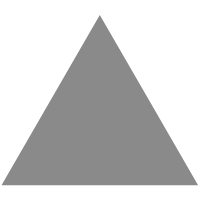
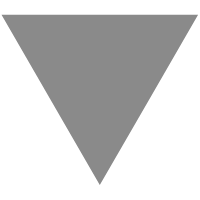
How to Break and Continue in Nested Loops in JavaScript
source link: https://www.dannyguo.com/blog/how-to-break-and-continue-in-nested-loops-in-javascript/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
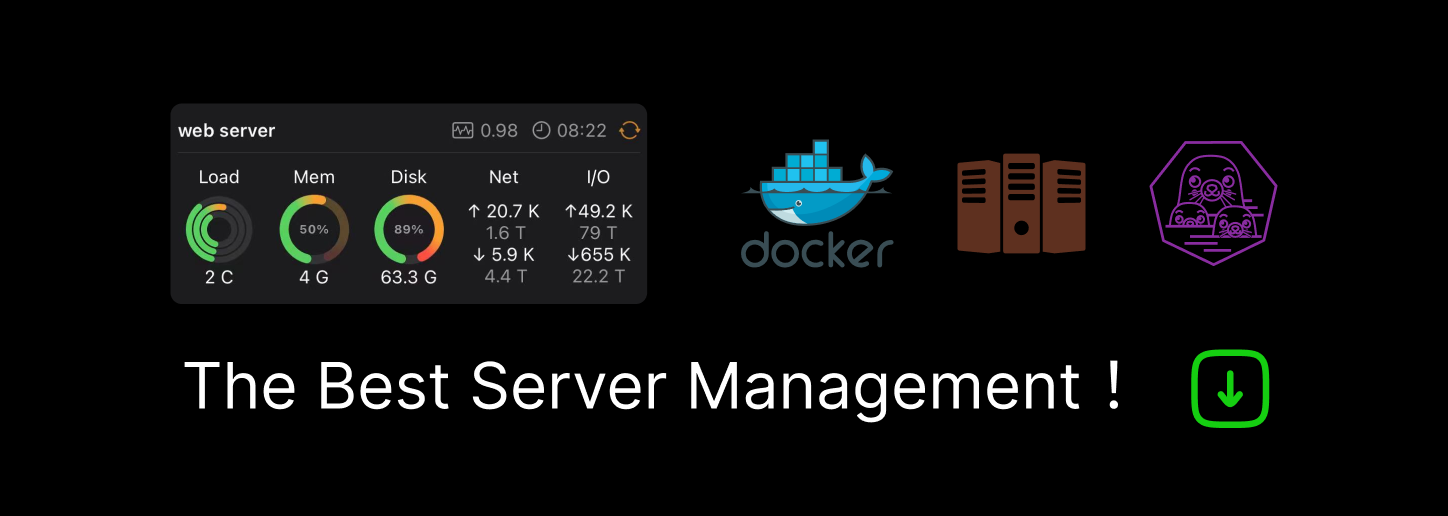
How to Break and Continue in Nested Loops in JavaScript
The easiest way to break
out of nested loops in JavaScript is to use
labels.
By labeling a loop, you can use it in a break
statement to break out of not
only the loop you’re in but also all the way out of the specified loop.
You can try out the examples in this post for yourself with this Replit.
const chunks = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
chunkLoop: for (const chunk of chunks) {
for (const number of chunk) {
if (number === 5) {
break chunkLoop;
}
console.log(number);
}
}
/*
Output:
1
2
3
4
*/
The output stops at 4 because when the code reaches 5, the code breaks all the
way out of the outer loop (chunkLoop
).
Continue
You can also use continue
with a label if you want to skip to the next
iteration of an outer loop.
const chunks = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
chunkLoop: for (const chunk of chunks) {
for (const number of chunk) {
if (number === 5) {
continue chunkLoop;
}
console.log(number);
}
}
/*
Output:
1
2
3
4
7
8
9
*/
5 and 6 aren’t in the output because when the code reaches 5, the code continues
on to the next iteration of the chunk loop, which is the [7, 8, 9]
chunk.
Follow me on Twitter or subscribe to my free newsletter or RSS feed for future posts.
Found an error or typo? Feel free to open a pull request on GitHub.
You can encrypt messsages with my GPG key if you'd like.
© 2022 Danny Guo
This content is licensed under CC BY-NC-SA 4.0 and hosted on GitHub.
As an Amazon Associate I earn from qualifying purchases.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK