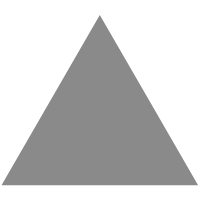
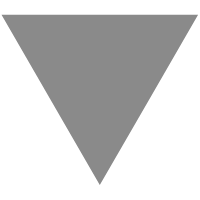
How To Manage C and C++ Packages using Conan
source link: https://computingforgeeks.com/how-to-manage-c-packages-using-conan/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Managing C and C++ packages can be a tough task at times. Many times, developers consider downloading sources, building, installing, and linking them to the libraries. This guide introduces the Conan package manager.
Conan is a free and open-source dependency and package manager for C and C++ language. This tool is similar to NuGet, npm for .NET, and JavaScript. Conan is a cross-platform tool that can run on any platform supporting Python i.e Windows, Mac, and Linux systems.
Conan has the ConanCenter, a central repository containing innumerable open-source libraries as well as precompiled binaries for mainstream compilers. These packages are maintained by a large, active community in Github repositories and the Slack (Conan channel). Futhermore, Conan has integration with the JFrog artifactory that includes a free Artifactory Community Edition for Conan. This allows developers to build their own packages on their servers.
This guide demonstrates how to install Conan and use it to manage C and C++ Packages.
Step 1 – Install Conan on Your System
There are several methods one can use to install Conan on Linux. The methods covered here are:
- Using PIP(Recommended)
- Using Package Managers
- Building from source
Option 1: Install Conan with pip (recommended)
Cona can be installed easily using Python PIP. All you need is Python 3.5 and above installed on your system.
Install Python 3 and PIP on your system using the command:
##On Debian/Ubuntu
sudo apt install python3 python3-pip
##On Rhel/CentOS/Rocky Linux/Alma Linux
sudo yum install python3 python3-pip
Once installed, verify the Python version.
$ python3 --version
Python 3.9.2
Now using Python PIP, install the Conan package manager:
sudo pip3 install conan
Sample output:
......
Successfully installed Jinja2-3.0.3 MarkupSafe-2.0.1 PyJWT-1.7.1 bottle-0.12.19 certifi-2021.10.8 charset-normalizer-2.0.12 colorama-0.4.4 conan-1.46.0 distro-1.6.0 fasteners-0.17.3 importlib-resources-5.4.0 node-semver-0.6.1 patch-ng-1.17.4 pluginbase-1.0.1 pygments-2.11.2 python-dateutil-2.8.2 requests-2.27.1 tqdm-4.63.0 urllib3-1.26.8 zipp-3.6.0
Option 2: Install Conan Using Package Managers
Conan can as well be installed from package managers such as brew and AUR.
- For Brew(OSX)
brew update
brew install conan
https://github.com/conan-io/conan/releases/latest/download/conan-win-32.exe
- AUR (Arch Linux)
yay -S conan
You can also install Conan by downloading binaries from the Conan website. Here, there are packages for Windows, Linux e.t.c
- Conan For Linux(Debian/Ubuntu)
Download the .DEB package file and install it on your Debian/Ubuntu using the below commands:
wget https://github.com/conan-io/conan/releases/latest/download/conan-ubuntu-64.deb
sudo dpkg -i conan-ubuntu-64.deb
Sample Output:
Selecting previously unselected package conan.
(Reading database ... 71512 files and directories currently installed.)
Preparing to unpack conan-ubuntu-64.deb ...
Unpacking conan (1.46.0) ...
Setting up conan (1.46.0) ...
Option 3: Install Conan by Building from source
This is yet another method one can use to install Conan on their system. With git
installed, proceed as below:
Install Python 3 on your system using the command:
##On Debian/Ubuntu
sudo apt install python3
##On RHEL/CentOS/Rocky Linux/Alma Linux
sudo yum install python3
Proceed to the Conan installation.
git clone https://github.com/conan-io/conan.git conan_src
cd conan_src
sudo python3 -m pip install -e .
Once installed, verify the Conan installation using the command:
$ conan
Consumer commands
install Installs the requirements specified in a recipe (conanfile.py or conanfile.txt).
config Manages Conan configuration.
get Gets a file or list a directory of a given reference or package.
info Gets information about the dependency graph of a recipe.
search Searches package recipes and binaries in the local cache or a remote. Unless a
remote is specified only the local cache is searched.
Creator commands
new Creates a new package recipe template with a 'conanfile.py' and optionally,
'test_package' testing files.
create Builds a binary package for a recipe (conanfile.py).
upload Uploads a recipe and binary packages to a remote.
export Copies the recipe (conanfile.py & associated files) to your local cache.
export-pkg Exports a recipe, then creates a package from local source and build folders.
test Tests a package consuming it from a conanfile.py with a test() method.
Package development commands
source Calls your local conanfile.py 'source()' method.
build Calls your local conanfile.py 'build()' method.
package Calls your local conanfile.py 'package()' method.
editable Manages editable packages (packages that reside in the user workspace, but are
consumed as if they were in the cache).
workspace Manages a workspace (a set of packages consumed from the user workspace that
belongs to the same project).
.......
Step 2 – Use Conan to Manage C and C++ Packages
Now with Conn installed, we can fetch and build packages. For example, here we will have a C++ project that depends on a third-party library(SQLite). This project will try to open a database in SQLite(testdb). In case the database does not exist, n database with the name will be created. In case it fails to open and create the database, the output printed will be “Can’t open the SQLite database“
Create the project file.
vim test_conan.cpp
In the file, add the lines below:
#include <iostream>
#include <sqlite3.h>
int main() {
sqlite3 *db;
int rc = sqlite3_open("test.db", &db);
if (SQLITE_OK == rc) {
std::cout << "Opened SQLite database successfully! \n";
sqlite3_close(db);
} else {
std::cout << "Can't open the SQLite database: " << sqlite3_errmsg(db) << "\n";
}
return 0;
}
Now we will use Conan to fetch the SQLite package. But first, we need to identify the version we want to use:
$ conan search sqlite3* --remote=conancenter
Existing package recipes:
sqlite3/3.29.0
sqlite3/3.30.1
sqlite3/3.31.0
sqlite3/3.31.1
sqlite3/3.32.1
sqlite3/3.32.2
.....
sqlite3/3.35.4
sqlite3/3.35.5
sqlite3/3.36.0
sqlite3/3.37.0
sqlite3/3.37.1
sqlite3/3.37.2
sqlite3/3.38.0
sqlite3/3.38.1
Select the newest available package, create a conanfile.txt file, and include the package name there. Also, specify the generator to be used(CMake)
$ vim conanfile.txt
[requires]
sqlite3/3.38.1
[generators]
cmake
Ensure that Cmake, GCC, and Ninja build are installed.
##On Debian/Ubuntu
sudo apt-get install build-essential
sudo apt install cmake ninja-build
##On Rhel/CentOS/Rocky Linux/Alma Linux
sudo yum install cmake
sudo yum install epel-release
sudo dnf config-manager --set-enabled powertools
sudo yum install ninja-build
sudo yum groupinstall "Development Tools" -y
Finally, create the CMakeLists.txt file, this includes settings for our project.
$ vim CMakeLists.txt
cmake_minimum_required (VERSION 3.8)
project(TestConan CXX)
set(CMAKE_CXX_STANDARD 11)
include(${CMAKE_BINARY_DIR}/conanbuildinfo.cmake)
conan_basic_setup()
add_executable(testConan test_conan.cpp)
target_link_libraries(testConan ${CONAN_LIBS})
Set the compiler to GCC. First, identify the compiler version.
$ gcc --version
gcc (GCC) 8.5.0 20210514 (Red Hat 8.5.0-4)
Copyright (C) 2018 Free Software Foundation, Inc.
This is free software; see the source for copying conditions. There is NO
warranty; not even for MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
The compiler can be exported as below:
export CONAN_COMPILER=gcc
export CONAN_COMPILER_VERSION=4.8
Alternatively, you can make the settings by editing the file:
$ vim .conan/profiles/default ***
[settings]
os=Linux
os_build=Linux
arch=x86_64
arch_build=x86_64
compiler=gcc
compiler.version=8
compiler.libcxx=libstdc++
build_type=Release
[options]
[build_requires]
[env]
Remember to set the compiler.version to the exact compiler version obtained from the command; gcc --version
That is it, now proceed and build the dependency library for SQLite. Normally done by creating a new directory and running the Conan commands there;
mkdir build
cd build
conan install ..
Build the source code. Here, we will use Ninja to build the system.
cmake .. -G Ninja
ninja
Sample Output:
.....
-- The CXX compiler identification is GNU 10.2.1
-- Detecting CXX compiler ABI info
-- Detecting CXX compiler ABI info - done
-- Check for working CXX compiler: /usr/bin/c++ - skipped
-- Detecting CXX compile features
-- Detecting CXX compile features - done
-- Conan: Adjusting output directories
-- Conan: Using cmake global configuration
-- Conan: Adjusting default RPATHs Conan policies
-- Conan: Adjusting language standard
-- Current conanbuildinfo.cmake directory: /home/thor/build
-- Conan: Compiler GCC>=5, checking major version 10
-- Conan: Checking correct version: 10
-- Configuring done
-- Generating done
-- Build files have been written to: /home/thor/build
thor@debian:~/build$ ninja
[2/2] Linking CXX executable bin/testConan
Once the process is complete, an executable file will be generated in the build folder at bin.
cd bin
Run the program.
$ ./testConan
Opened SQLite database successfully!
Step 3 – Installing Dependencies with Canon
Canon allows one to install dependencies when using the conan install command. For the example:
conan install .. --settings os="Linux" --settings compiler="gcc"
The above command:
- Checks if the package recipe (for sqlite3/3.38.1 or the specified package) exists in the local cache. If we are just starting, the cache is empty.
- Looks for the package recipe in the defined remotes. Conan comes with conancenter remote as the default, but can be changed.
- If the recipe exists, the Conan client fetches and stores it in your local Conan cache.
- With the package recipe and the input settings (Linux, GCC), Conan looks for the corresponding binary in the local cache.
- As the binary is not found in the cache, Conan looks for it in the remote and fetches it.
- Finally, it generates an appropriate file for the build system specified in the [generators] section.
You can also view available dependencies in case you want to reuse them using the command:
$ conan search "*"
Existing package recipes:
sqlite3/3.38.1
zlib/1.2.11
openssl/1.0.2t
To search for a package, use the command conan search with the package and remote repo
$ conan search poco* --remote=conancenter
Existing package recipes:
poco/1.8.1
poco/1.9.3
poco/1.9.4
poco/1.10.0
poco/1.10.1
poco/1.11.0
poco/1.11.1
You can inspect an existing package on your system using the command:
$ conan search sqlite3/3.38.1@
Existing packages for recipe sqlite3/3.38.1:
Package_ID: 51b33eeec832c7723359c5da8c7cfc0bc4f75db4
[options]
build_executable: True
disable_gethostuuid: False
enable_column_metadata: True
enable_dbpage_vtab: False
enable_dbstat_vtab: False
enable_default_secure_delete: False
enable_default_vfs: True
.........
Get information about your project:
$ conan info ..
conanfile.txt
ID: 19ab1cbb60d862f43c0b6aaefa1ce0c1cac1188a
BuildID: None
Context: host
Requires:
sqlite3/3.38.1
sqlite3/3.38.1
ID: 51b33eeec832c7723359c5da8c7cfc0bc4f75db4
BuildID: None
Context: host
Remote: conancenter=https://center.conan.io
URL: https://github.com/conan-io/conan-center-index
Homepage: https://www.sqlite.org
License: Unlicense
Description: Self-contained, serverless, in-process SQL database engine.
Topics: sqlite, database, sql, serverless
Provides: sqlite3
Recipe: Cache
Binary: Cache
Binary remote: conancenter
Creation date: 2022-03-15 03:35:24 UTC
Required by:
conanfile.txt
Get help when using Conan.
$ conan --help
Consumer commands
install Installs the requirements specified in a recipe (conanfile.py or conanfile.txt).
config Manages Conan configuration.
get Gets a file or list a directory of a given reference or package.
info Gets information about the dependency graph of a recipe.
search Searches package recipes and binaries in the local cache or a remote. Unless a
remote is specified only the local cache is searched.
Creator commands
new Creates a new package recipe template with a 'conanfile.py' and optionally,
'test_package' testing files.
create Builds a binary package for a recipe (conanfile.py).
upload Uploads a recipe and binary packages to a remote.
export Copies the recipe (conanfile.py & associated files) to your local cache.
export-pkg Exports a recipe, then creates a package from local source and build folders.
test Tests a package consuming it from a conanfile.py with a test() method.
Package development commands
source Calls your local conanfile.py 'source()' method.
build Calls your local conanfile.py 'build()' method.
package Calls your local conanfile.py 'package()' method.
editable Manages editable packages (packages that reside in the user workspace, but are
consumed as if they were in the cache).
workspace Manages a workspace (a set of packages consumed from the user workspace that
belongs to the same project).
Misc commands
profile Lists profiles in the '.conan/profiles' folder, or shows profile details.
remote Manages the remote list and the package recipes associated with a remote.
user Authenticates against a remote with user/pass, caching the auth token.
imports Calls your local conanfile.py or conanfile.txt 'imports' method.
copy Copies conan recipes and packages to another user/channel.
remove Removes packages or binaries matching pattern from local cache or remote.
alias Creates and exports an 'alias package recipe'.
download Downloads recipe and binaries to the local cache, without using settings.
inspect Displays conanfile attributes, like name, version, and options. Works locally,
in local cache and remote.
help Shows help for a specific command.
lock Generates and manipulates lock files.
frogarian Conan The Frogarian
Conan commands. Type "conan <command> -h" for help
Closing Thoughts.
That is it! We have successfully installed Conan and demonstrated how you can use it to manage C and C++ packages. I hope this was significant.
Related posts:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK