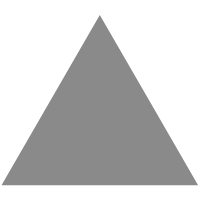
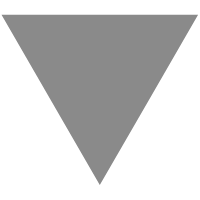
Exception Handling in Apache Camel using Spring
source link: https://blog.knoldus.com/exception-handling-in-apache-camel-using-spring/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
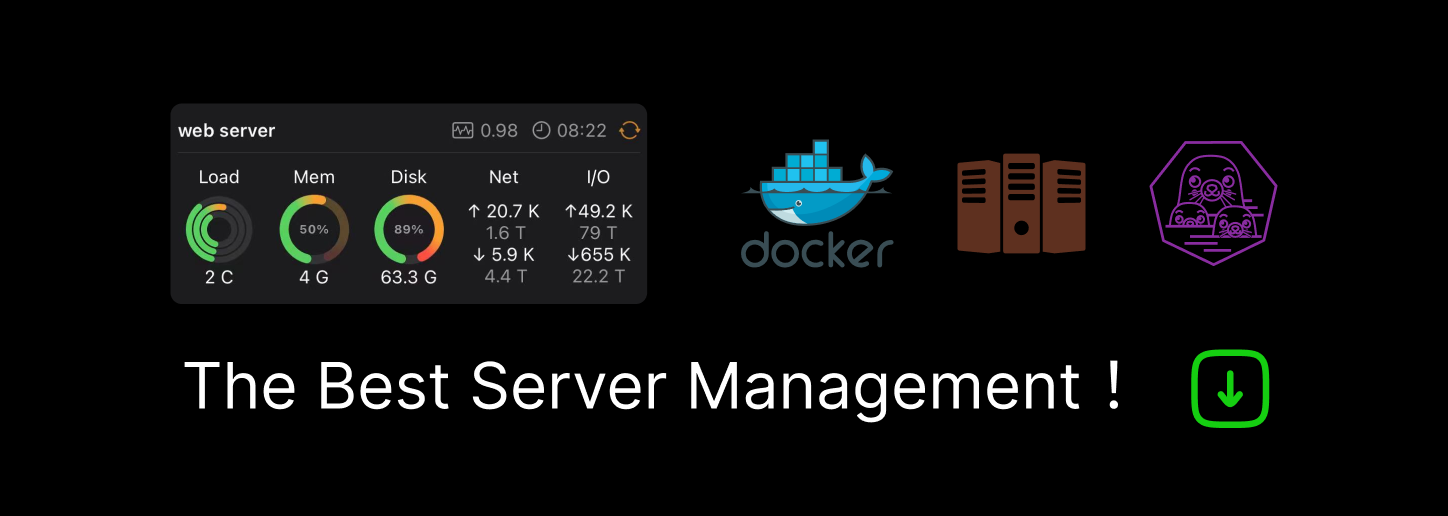
Exception Handling in Apache Camel using Spring
Reading Time: 3 minutes
Apache Camel is a rule-based routing and mediation engine that provides a Java object-based implementation of the Enterprise Integration Patterns using an API (or declarative Java Domain Specific Language) to configure routing and mediation rules. In this post we will implement Exception Handling in Apache Camel using Spring.
Features of Apache Camel
- Apache camel is a lightweight framework.
- It provides us with a number of components. These components interact and create endpoints with which a system can interact with other external systems.
- It uses Message Exchange Patterns(MEP).
- Camel provides many different type converters for marshaling and unmarshalling the message during routing.
- Routes in a variety of domain-specific languages (DSL).The most popular ones are
1. Java DSL – A Java based DSL using the fluent builder style.
2. Spring XML – A XML based DSL in Spring XML files
When using Spring XML we can make use of Spring support for
features like Transaction Management, JPA etc.
The project structure will be as follows-
Exception handling In Apache camel can be implemented in 2 ways.
- Using Do Try block
- Using OnException block
The pom.xml will be as follows-
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.spring</groupId>
<artifactId>camel-spring-integration</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-core</artifactId>
<version>2.13.0</version>
</dependency>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-spring</artifactId>
<version>2.13.0</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.5</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>1.7.5</version>
</dependency>
</dependencies>
</project>
Define a custom exception as follows-
package com.spring.exception;
public class CamelCustomException extends Exception {
private static final long serialVersionUID = 1L;
}
Create the camel processor(MyProcessor) and we throw this custom exception.
package com.spring.processor;
import org.apache.camel.Exchange;
import org.apache.camel.Processor;
import com.spring.exception.CamelCustomException;
public class MyProcessor implements Processor {
public void process(Exchange exchange) throws Exception {
System.out.println("Exception Thrown");
throw new CamelCustomException();
}
}
Define MainApplication class
package com.spring.main;
import org.springframework.context.support.AbstractApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MainApplication {
public static void main(String[] args) {
AbstractApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml");
ctx.start();
System.out.println("Application context started");
try {
System.out.println("inside try block");
Thread.sleep(5 * 60 * 1000);
}
catch (InterruptedException e) {
e.printStackTrace();
}
ctx.stop();
ctx.close();
}
}
Create applicationContext.xml file and configure routeBuilder bean
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:camel="http://camel.apache.org/schema/spring"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://camel.apache.org/schema/spring
http://camel.apache.org/schema/spring/camel-spring.xsd">
<bean id="routeBuilder" class="com.spring.route.SimpleRouteBuilder" />
<camelContext xmlns="http://camel.apache.org/schema/spring">
<routeBuilder ref="routeBuilder" />
</camelContext>
</beans>
In SimpleRouteBuilder class no exception handling code written.
package com.spring.route;
import org.apache.camel.builder.RouteBuilder;
import com.spring.processor.MyProcessor;
public class SimpleRouteBuilder extends RouteBuilder {
@Override
public void configure() throws Exception {
from("file:/home/knoldus/Downloads/Softwares/Workspace/input?noop=true").process(new MyProcessor()).to("file:/home/knoldus/Downloads/Softwares/Workspace/output");
}
}
Using Do Try block : It is similar to the Java try catch block, thrown exception will be immediately caught and the message wont keep on retrying. The limitation of this approach is that it is applicable only for the single route. Suppose we have one more seperate route.
Using OnException block : The OnException block is written as a separate block from the routes.
This applies to all the routes. Below for both route starting.
package com.spring.route;
import org.apache.camel.Exchange;
import org.apache.camel.Processor;
import org.apache.camel.builder.RouteBuilder;
import com.spring.exception.CamelCustomException;
import com.spring.processor.MyProcessor;
public class SimpleRouteBuilder extends RouteBuilder {
@Override
public void configure() throws Exception {
onException(CamelCustomException.class).process(new Processor() {
public void process(Exchange exchange) throws Exception {
System.out.println("handling ex");
}
}).log("Received body ").handled(true);
from("file:/home/knoldus/Downloads/Softwares/Workspace/input?noop=true").process(new MyProcessor()).to("file:/home/knoldus/Downloads/Softwares/Workspace/output");
from("file:/home/knoldus/Downloads/Softwares/Workspace/New/input?noop=true").process(new MyProcessor()).to("file:/home/knoldus/Downloads/Softwares/Workspace/New/output");
}
}
We get the output as follows.
Conclusion
In this blog, we have covered how to implement and configure Exception Handling in Apache Camel using Spring. Now you are ready to go to implement Exception Handling in Apache Camel using Spring. For more, you can refer to the documentation: https://www.tutorialspoint.com/apache_camel/apache_camel_using_with_spring.htm
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK