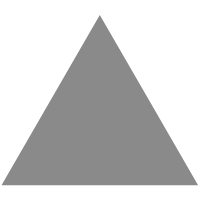
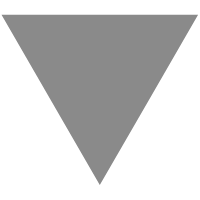
Using PHPUnit
source link: https://www.hhutzler.de/blog/using-phpunit/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Installation Directories and Software Versions
- PHP was used from our XAMPP installation at: d:\xampp\php;
- PHPUnit Installation Direcotory: D:\PHP\phpunit;
- Windows 7
- NetBeans 8.2
- PHP 5.5.15
- PHPUnit 4.8.36
- phpunit-skelgen-1.2.1.
Install PHPUnit on Windows7
Verify your PHP version from your XAMPP install on Windows
D:\PHP\phpunit> d:\xampp\php\php.exe --version PHP 5.5.15 (cli) (built: Jul 23 2014 15:05:09) Copyright (c) 1997-2014 The PHP Group Zend Engine v2.5.0, Copyright (c) 1998-2014 Zend Technologies with Xdebug v2.2.3, Copyright (c) 2002-2013, by Derick Rethans
Download PHPUnit and phpunit-skelgen package
Packages selected for download based on my PHP 5.5.15 version
- phpunit-4.8.36.phar
- phpunit-skelgen-1.2.1.phar
- Directory location for PHPUnit files: D:\PHP\phpunit
Rename/Copy files
- phpunit-4.8.36.phar -> phpunit.phar
- phpunit-skelgen-1.2.1.phar -> phpunit-skelgen.phar
Create a new PHPUnit Command File
- D:\PHP\phpunit> echo @php “%~dp0phpunit.phar” %* > phpunit.cmd
Modify your Windows Path varaible and verify your installation
Add to your windows PATH: D:\PHP\phpunit;d:\xampp\php; .... Finally verify the PHPUnit installation D:\Users\helmut> phpunit --version PHPUnit 4.8.36 by Sebastian Bergmann and contributors.
Run a PHPUnit Sample from the Command Line
Directory structure matches Netbeans Project structure
* Directory structure: * ├── PhpUnitTest * ├── Calculator.php * ├── tests * ├── bootstrap.php * ├── CalculatorTest.php
Calculator.php our PHP test class
class Calculator { /** * @assert (0, 0) == 0 * @assert (0, 1) == 1 * @assert (1, 0) == 1 * @assert (1, 1) == 2 * @assert (1, 2) == 4 */ public function add($a, $b) { return $a + $b; } }
bootstrap.php our PHP class to autoload PHP classes for testing
chdir(".."); // Note our tests directory is located just below our real PHP files echo "Current Working Directory:: " . getcwd() . "\n"; class Autoloader{ public static function register(){ //echo "Inside Register ..\n"; spl_autoload_register(function ($class) { $file = str_replace('\\', DIRECTORY_SEPARATOR, $class).'.php'; if (file_exists($file)) { require $file; echo "+++ Autoload of PHP File:: " . $file . "\n"; return true; } return false; }); } } Autoloader::register();
Create the test class using phpunit-skelgen.phar
D:\xampp\htdocs\tc\PhpUnitTest> php \php\phpunit\phpunit-skelgen.phar --test Calculator PHPUnit Skeleton Generator 1.2.1 by Sebastian Bergmann. Wrote skeleton for "CalculatorTest" to "D:\xampp\htdocs\tc\PhpUnitTest\CalculatorTest.php". Copy CalculatorTest.php to the tests directory D:\xampp\htdocs\tc\PhpUnitTest> copy CalculatorTest.php tests tests\CalculatorTest.php überschreiben? (Ja/Nein/Alle): ja
Test class generated by phpunit-skelgen.phar
/** * Generated by PHPUnit_SkeletonGenerator 1.2.1 on 2018-03-04 at 12:37:11. */ class CalculatorTest extends PHPUnit_Framework_TestCase { /** * @var Calculator */ protected $object; /** * Sets up the fixture, for example, opens a network connection. * This method is called before a test is executed. */ protected function setUp() { $this->object = new Calculator; } /** * Tears down the fixture, for example, closes a network connection. * This method is called after a test is executed. */ protected function tearDown() { } /** * Generated from @assert (0, 0) == 0. * * @covers Calculator::add */ public function testAdd() { $this->assertEquals( 0, $this->object->add(0, 0) ); } /** * Generated from @assert (0, 1) == 1. * * @covers Calculator::add */ public function testAdd2() { $this->assertEquals( 1, $this->object->add(0, 1) ); } /** * Generated from @assert (1, 0) == 1. * * @covers Calculator::add */ public function testAdd3() { $this->assertEquals( 1, $this->object->add(1, 0) ); } /** * Generated from @assert (1, 1) == 2. * * @covers Calculator::add */ public function testAdd4() { $this->assertEquals( 2, $this->object->add(1, 1) ); } /** * Generated from @assert (1, 2) == 4. * * @covers Calculator::add */ public function testAdd5() { $this->assertEquals( 4, $this->object->add(1, 2) ); } }
Run the php Unit test
D:\xampp\htdocs\tc\PhpUnitTest\tests> phpunit --bootstrap bootstrap.php CalculatorTest.php Current Working Directory:: D:\xampp\htdocs\tc\PhpUnitTest PHPUnit 4.8.36 by Sebastian Bergmann and contributors. . +++ Autoload of PHP File:: Calculator.php ...F Time: 237 ms, Memory: 9.50MB There was 1 failure: 1) CalculatorTest::testAdd5 Failed asserting that 3 matches expected 4. D:\xampp\htdocs\tc\PhpUnitTest\tests\CalculatorTest.php:91 FAILURES! Tests: 5, Assertions: 5, Failures: 1.
Return Codes from phpUnit
* PHPUnit Return Codes: * . Printed when the test succeeds. * F Printed when an assertion fails while running the test method. * E Printed when an error occurs while running the test method. * R Printed when the test has been marked as risky (see Chapter 6). * S Printed when the test has been skipped (see Chapter 7). * I Printed when the test is marked as being incomplete or not yet implemented (see Chapter 7).
PHPUnit and Netbeans Integration
Generic setup for PHPUnit within NetBeans IDE
Tools -> Option -> PHP -> PHP Framework -> PHPUnit
Create a new PHP project with the above Source for calcualater.php
Setup PHPUnit as test Provider
Right Click Project [ PhpUnitTest3] -> Properties -> Testing -> Add Folder
Create the PHP Test Classes
Right Click on Calculator.php -> Tools -> Create/Update Tests
Netbeans should create CalculatorTest.php in out tests directory by running: "D:\xampp\php\php.exe" "D:\PHP\phpunit\phpunit-skelgen.phar" "--test" "--" "Calculator" "D:\xampp\htdocs\PhpUnitTest3\Calculator.php" "CalculatorTest" "D:\xampp\htdocs\PhpUnitTest3\tests\CalculatorTest.php" PHPUnit Skeleton Generator 1.2.1 by Sebastian Bergmann. Wrote skeleton for "CalculatorTest" to "D:\xampp\htdocs\PhpUnitTest3\tests\CalculatorTest.php".
Copy above bootstrap.php to our test folder and modify PHPUnit settings
Right Click Project [ PhpUnitTest3] -> Properties -> PHPUnit
Project Files and Directories sould now look like: * Directory structure: * ├── PhpUnitTest * ├── Calculator.php * ├── tests * ├── bootstrap.php * ├── CalculatorTest.php
Finally run PHPUnit tests
Right Click Calculator.php -> Test
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK