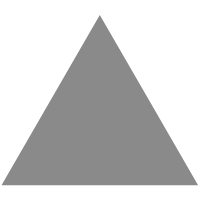
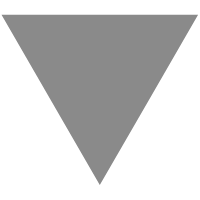
Find all factors for fake numbers
source link: https://www.codesd.com/item/find-all-factors-for-fake-numbers.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
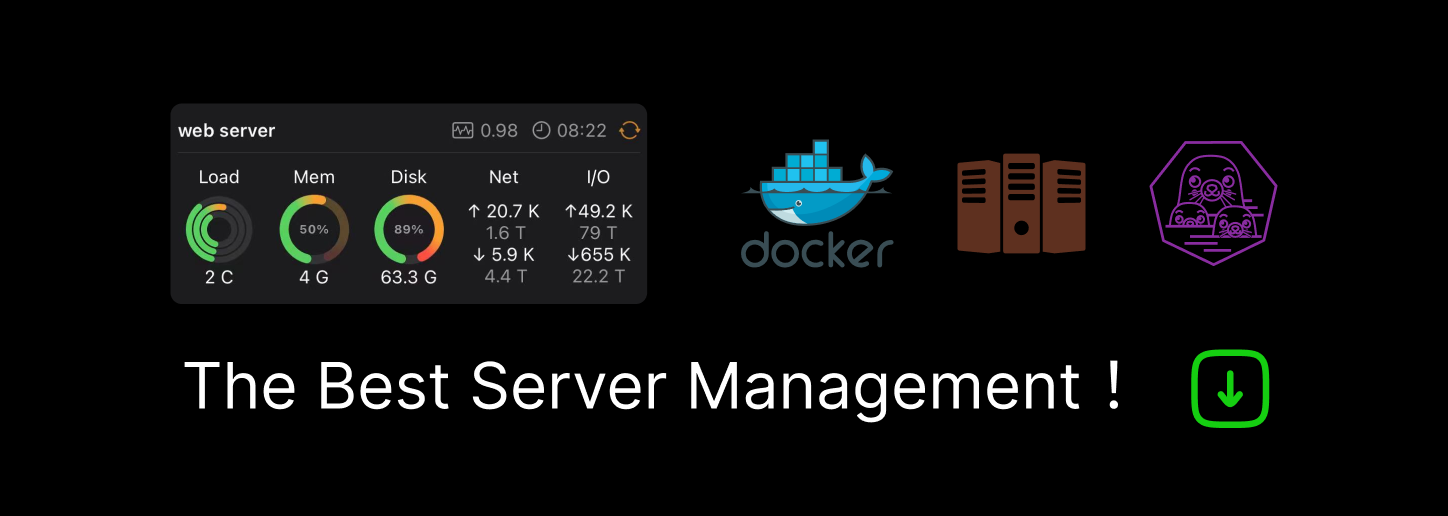
Find all factors for fake numbers
I am trying to find the quickest full factor algorithm. I use put all the factors into an arraylist for addition and compare the sum against the original number to find out if they are the same.
example. 6 has the factors of [1,2,3} if you add 1+2+3 = 6.
Is there a quicker way to factorize, add, and compare, other than the program i have now?
public class Phony_Number {
private int number;
public Phony_Number(int num) {
number = num;
print();
}
public Phony_Number() {
number = 0;
}
private ArrayList<Integer> factoring(int num) {
ArrayList<Integer> factors = new ArrayList<Integer>();
if (num % 2 == 0) {
for (int ff = 3; ff < num; ff++) {
if (num % ff == 0) {
factors.add(ff);
}
}
}
return factors;
}
private int sum(ArrayList<Integer> array) {
int sum = 0;
for (int i = 0; i < array.size(); i++) {
sum = +sum + array.get(i);
}
return sum+3;
}
private boolean compare(int num, int sum) {
if (num == sum)
return true;
return false;
}
public void print() {
for (int i = number; i > 5; i--) {
if (compare(i, sum(factoring(i)))) {
System.out.println("Number " + i + " is phony number");
}
}
}
My current result for 20,000 numbers is this
Number 8128 is phony number
Number 496 is phony number
Number 28 is phony number
Number 6 is phony number
Nano RunTime 359624716
A few little things jumped out: Your compare method is pointless, remove that and it will simplify your code a little. Your sum method can just use +=. Why loop through every number then drop half of them, just use -=2 on the loop.
Your main saving though can come from your factoring
method - you know that numbers over num/2
cannot be factors so you can stop as soon as you get to there.
(In fact in this case you may be able to stop at num/3
as you are skipping 2.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK