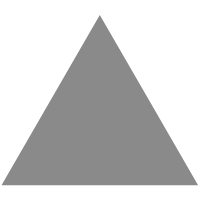
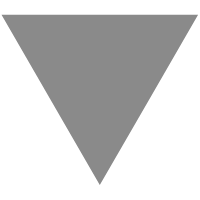
5 Python Slicing Tricks That Will Make Your Code More Elegant
source link: https://medium.com/techtofreedom/5-python-slicing-tricks-that-will-make-your-code-more-elegant-bd5e27c73f7
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
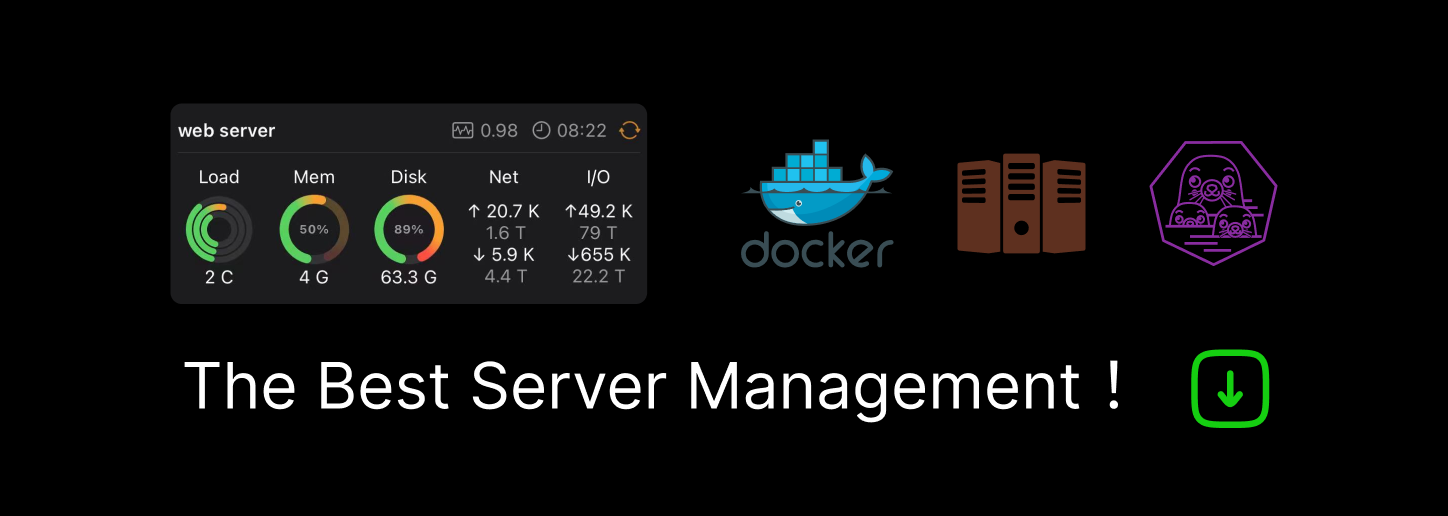
5 Python Slicing Tricks That Will Make Your Code More Elegant
Small tricks for a big difference
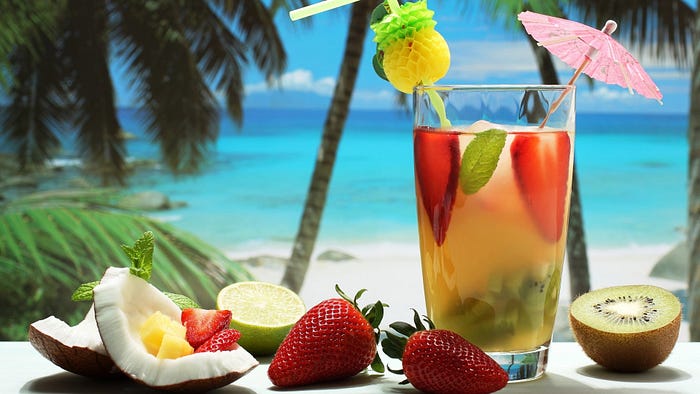
In the Python world, there are many small tricks that can make a big difference to our code. Especially for a large program, these tricks can keep everything neat and elegant.
The slice operator is one of them. It helps us get items from iterables easily and elegantly. For example, we have a list as follows:
>>> a = [1,2,3,4,5,6]
If we need to get the first, third and fifth items, how to write the code?
A Python newbie could write a for-loop including an if-else statement to filter the items. It works, but there is too much unnecessary code.
Actually, the Pythonic way to handle this is a one-line solution:
>>> a[::2]
No for-loops. No if-else statements. Just one line. This is the power of the slice technique.
This article will introduce 5 useful slicing tricks in Python, which will help us a lot if we use them properly.
The Template of a Slice Operator
Before exploring its usages, we need to know the template of writing a slice operator :
a_list[start:end:step]
As shown above, there are three components:
- start: the starting index (default value is 0)
- end: the ending index (default value is the length of the list)
- step: defines the steps when iterating the list (default value is 1)
All the three components are optional. This fact could be unbelievable but it’s true.
After knowing the template, now, let’s check out how the 5 tricks work.
1. Get the First or Last N Items of a List
Let’s use the previous list as an example again:
>>> a = [1,2,3,4,5,6]
How to get the first 3 items? Simple:
>>> a[:3]
[1, 2, 3]
As the above code shown, since the “start” component is optional and its default value is 0, we just need to define where to stop.
How to get the last 3 items? The negative index can help:
>>> a[-3:]
[4, 5, 6]
As we all known, most programming languages support positive index, and the index starts from 0.
But Python, as a gorgeous language, supports negative index. It starts from -1. In other words, -1 stands for the last item, -2 stands for the second last item, and so on.
Therefore, when we need to get the last n items of a list, just get it by the means of negative index.
2. Take Every Nth Item of a List
The third component of the template can give us more flexibility to deal with slicing tasks. For example, we can get the items at odd positions of a list:
>>> a = [1, 2, 3, 4, 5, 6]
>>> a[::2]
[1, 3, 5]
A commonly used trick is to reverse a list as follows:
>>> a = [1, 2, 3, 4, 5, 6]
>>> a[::-1]
[6, 5, 4, 3, 2, 1]
A positive “step” will start from the beginning of a list, and a negative “step” will start from the end.
3. Shallow Copy a List
The simplest way to shallow copy a list is using the following slicing trick:
>>> a = [1, 2, 3, 4, 5, 6]
>>> b = a[:]
>>> b[0]=100
>>> b
[100, 2, 3, 4, 5, 6]
>>> a
[1, 2, 3, 4, 5, 6]
With the help of the above trick, we don’t need to import the built-in copy
module if we just need a shallow copy of a list.
4. Operate Multiple Items at Once
If we need to handle multiple items in a list, do we have to use some boring for-loops?
Not really. We can still do some tasks, such as assignment or deletion, by the slicing operator. No for-loops are needed. Let’s see some examples:
Assign multiple items at once
>>> a = [1, 2, 3, 4, 5, 6]
>>> a[:3] = [7,8,9]
>>> a
[7, 8, 9, 4, 5, 6]
Delete multiple items at once
>>> a = [1, 2, 3, 4, 5, 6]
>>> del a[:2]
>>> a
[3, 4, 5, 6]
Resize a list by other items
>>> a = [1, 2, 3, 4, 5, 6]
>>> a[:4] = [7,8]
>>> a
[7, 8, 5, 6]
5. Use the Slice Object To Store Indexes
If we will slice many lists by the same indexes, there could be too many numbers and our code will become ugly even with the help of slicing tricks. Like the following code:
a = [1, 2, 3, 4, 5, 6]
b = [3, 4, 5, 6, 7, 8, 9]
c = [2, 3, 4, 5, 100, 101, 102]
a2 = a[1:5:2]
b2 = b[1:5:2]
c2 = c[1:5:2]
Not to mention that if the required indexes changed, we have to modify the corresponding indexes one by one.
Generally speaking, it’s not a good practice to put many same numbers in one program. In this scenario, the slice object can help:
a = [1, 2, 3, 4, 5, 6]
b = [3, 4, 5, 6, 7, 8, 9]
c = [2, 3, 4, 5, 100, 101, 102]indexes = slice(1, 5, 2)a2 = a[indexes]
b2 = b[indexes]
c2 = c[indexes]
As shown above, the built-in slice object is a simple assist to help us store the three numbers and use it when needed. If the indexes changed, we just need to modify the numbers in the slice object.
Conclusion
Python gives us some syntax sugar to make our code more readable and elegant. The slicing technique is a good example. Understand and apply these small tricks can improve the quality of our code very much.
Thanks for reading. If you like it, don’t forget to follow me to get more great articles about programming and technologies!
Relative articles:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK