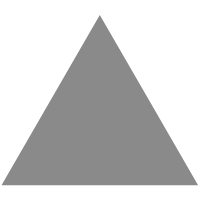
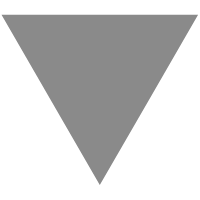
Project learning-framework learning-Swagger3
source link: https://blog.birost.com/a?ID=00000-7bf1c67b-a396-4041-9e35-55a865ddbdc0
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
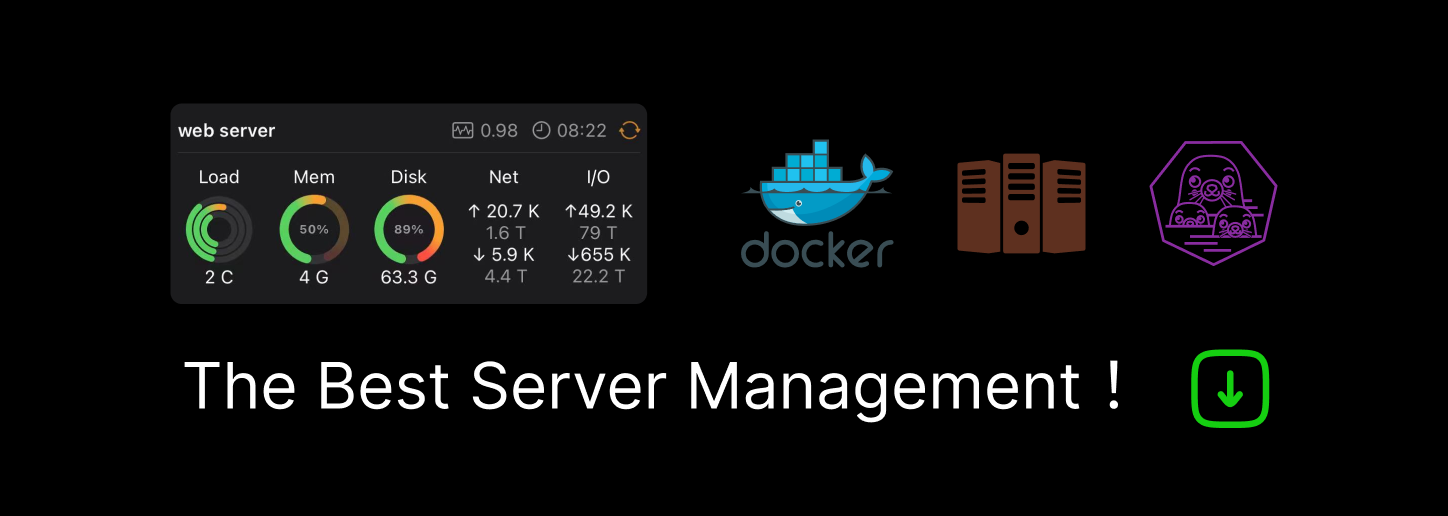
Concept and function
concept:
Swagger is a standardized and complete framework for generating, describing, invoking and visualizing RESTful Web services.
effect:
- Support API to automatically generate synchronized online documents. Another way is to write Office documents to provide interface documents.
- Provide Web page online test API. Another common way is to use postman test interface.
SpringBoot integrates Swagger
The minimum requirement for integration is that it can be used, similar to a Hello World project.
Note:
- jdk needs to use version 1.8 or above
- Access address of version 3.0 of swagger2/swagger-ui/index.html Access address before 2.x/swagger-ui.html
- maven file configuration
< dependency > < groupId > io.springfox </groupId > < artifactId > springfox-swagger2 </artifactId > < version > 3.0.0 </version > </dependency >
< dependency > < groupId > io.springfox </groupId > < artifactId > springfox-swagger-ui </artifactId > < version > 3.0.0 </version > </dependency >
< Dependency > < the groupId > io.springfox </the groupId > < the artifactId > springfox-Boot-Starter </the artifactId > < Version > 3.0.0 </Version > </dependency > copy the code
- Add @EnableOpenApi annotation above Application startup application class
The effect after the project is launched is:
SpringBoot configuration Swagger
Custom configuration class
import org.apache.commons.lang3.reflect.FieldUtils; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.util.ReflectionUtils; import org.springframework.web .servlet.config.annotation.InterceptorRegistration; import org.springframework.web.servlet.config.annotation.InterceptorRegistry; import org.springframework.web.servlet.config.annotation.WebMvcConfigurer; import springfox.documentation.builders.ApiInfoBuilder; import springfox .documentation.builders.PathSelectors; importspringfox.documentation.builders.RequestHandlerSelectors; import springfox.documentation.service.ApiInfo; import springfox.documentation.service.Contact; import springfox.documentation.spi.DocumentationType; import springfox.documentation.spring.web.plugins.Docket;
import java.lang.reflect.Field; import java.util.List;
@Configuration public class Swagger3Config implements WebMvcConfigurer { @Bean public Docket createRestApi () { return new Docket(DocumentationType.OAS_30) .apiInfo(apiInfo()) .select() //Through the .select() method, to configure the scanning interface, RequestHandlerSelectors configure how to scan the interface /* basePackage(final String basePackage)//scan the interface according to the package path any()//Scan all, all interfaces in the project will be scanned none()//do not scan the interface //Scan through annotations on methods, such as withMethodAnnotation(GetMapping.class) only scan get requests withMethodAnnotation(final Class<? extends Annotation> annotation) //Scan through annotations on the class, such as .withClassAnnotation(Controller.class) only scans interfaces in classes with controller annotations withClassAnnotation(final Class<? extends Annotation> annotation) */ .apis(RequestHandlerSelectors.basePackage( "com.study.swagger3.controller" )) //basePackage scans the interface according to the package path /* any()//scan any request none()//Do not scan any request regex(final String pathRegex)//control by regular expression ant(final String antPattern)//control by ant() */ .paths(PathSelectors.any()) //Configure how to filter by path, that is, scan all requests here any none ant regex .build(); }
/** * The top half of the API page displays information */ private ApiInfo apiInfo () { return new ApiInfoBuilder() .title( "Swagger3 Interface Document" ) .description( "document description" ) .contact( new Contact( "hughJin" , "#" , "[email protected]" )) .version( "1.0" ) .build(); }
/** * Universal interceptor excludes swagger settings, all interceptors will automatically add swagger-related resource exclusion information */ @Override public void addInterceptors (InterceptorRegistry registry) { try { Field registrationsField = FieldUtils.getField(InterceptorRegistry.class, "registrations" , true ); List<InterceptorRegistration> registrations = (List<InterceptorRegistration>) ReflectionUtils.getField(registrationsField, registry); if (registrations != null ) { for (InterceptorRegistration interceptorRegistration: registrations) { interceptorRegistration .excludePathPatterns( "/swagger**/**" ) .excludePathPatterns( "/webjars/**" ) .excludePathPatterns( "/v3/**" ) .excludePathPatterns( "/doc.html" ); } } } catch (Exception e) { e.printStackTrace(); } } } Copy code
Common Swagger annotations and instructions
Swagger annotationBrief description@Api(tags = "xxx module description")Act on the module class@ApiOperation("xxx interface description")Act on interface methods@ApiModel("xxxPOJO description")Act on the model class: such as VO, BO@ApiModelProperty(value = "xxx property description", hidden = true)Act on class methods and properties, hidden is set to true to hide the property@ApiParam("xxx parameter description")Act on parameters, methods and fields, similar to @ApiModelProperty
Swagger annotation code example
import com.study.swagger3.dto.User; import io.swagger.annotations.Api; import io.swagger.annotations.ApiImplicitParam; import io.swagger.annotations.ApiOperation; import io.swagger.annotations.ApiParam; import org. springframework.web.bind.annotation.*; import springfox.documentation.annotations.ApiIgnore;
@Api(value = "@Api: Used on the controller class to indicate the description of the class", tags = "Swagger test user information management API") @RestController @RequestMapping("/user") public class SwaggerController {
@ApiIgnore //Ignore this API @GetMapping("/hello") public String hello () { return "hello" ; }
@GetMapping(value = "/swaggerGet/{name}") @ApiOperation(value = "Interface method description", notes = "@ApiOperation: used on the requested method to explain the purpose and function of the method") @ApiImplicitParam(name = "name", value = "@ApiImplicitParams: used in the request method to indicate a set of parameter descriptions", required = true, dataType = "String", paramType = "path") public String swaggerGet ( @PathVariable String name) { return "name=" +name; }
@PostMapping(value = "/swaggerPost") @ApiOperation(value = "Add user", notes = "@ApiOperation Swagger test RESTful POST request test input a POJO (JSON format)") public User swaggerGet ( @RequestBody User user) { return user; }
@GetMapping(value = "/swaggerApiParam") @ApiOperation(value = "test parameter") public User swaggerApiParam ( @ApiParam(value = "primary key query" ,required = true) String id) { return new User(); }
}
//////////////////////////////////////
import io.swagger.annotations.ApiModel; import io.swagger.annotations.ApiModelProperty;
import java.io.Serializable;
@ApiModel("Entity class@ApiModel: used on the response class (POJO entity class), describing a return response data information (description of the entity description of the POJO class request or response)") public class User implements Serializable {
private static final long serialVersionUID = -6627165976923138391L ;
@ApiModelProperty(value = "Username@ApiModelProperty: Used on POJO properties to describe the property description of the response class", dataType = "String", name = "username", example = "hughJin") private String username; @ApiModelProperty( value = "Account password", dataType = "String", name = "password", example = "123456") private String password;
public String getUsername () { return username; }
public void setUsername (String username) { this .username = username; }
public String getPassword () { return password; }
public void setPassword (String password) { this .password = password; }
@Override public String toString () { return "User{" + "username='" + username + '\'' + ", password='" + password + '\'' + '}' ; } } Copy code
Integrated knife4j
The access path after integration is:
The integrated effect is:
Swagger3 project source code path:
Link: pan.baidu.com/s/1YC6tKQmk... Extraction code: tidu
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK