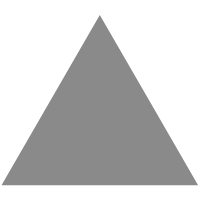
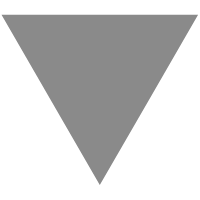
Pointer to char vs String (updated)
source link: https://www.codesd.com/item/pointer-to-char-vs-string-updated.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
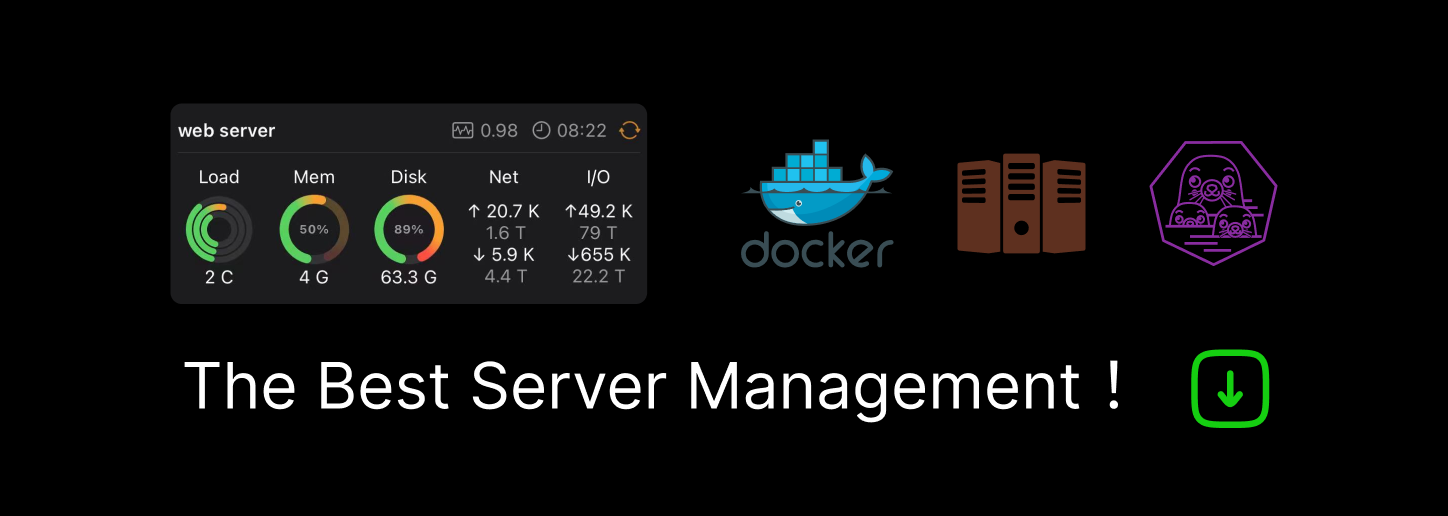
Pointer to char vs String (updated)
Consider these two pieces of code. They're converting base10 number to baseN number, where N
is the number of characters in given alphabet. Actually, they generate permutations of letters of given alphabet. It's assumed that 1 is equal to first letter of the alphabet.
#include <iostream>
#include <string>
typedef unsigned long long ull;
using namespace std;
void conv(ull num, const string alpha, string *word){
int base=alpha.size();
*word="";
while (num) {
*word+=alpha[(num-1)%base];
num=(num-1)/base;
}
}
int main(){
ull nu;
const string alpha="abcdef";
string word;
for (nu=1;nu<=10;++nu) {
conv(nu,alpha,&word);
cout << word << endl;
}
return 0;
}
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
typedef unsigned long long ull;
void conv(ull num, const char* alpha, char *word){
int base=strlen(alpha);
while (num) {
(*word++)=alpha[(num-1)%base];
num=(num-1)/base;
}
}
int main() {
char *a=calloc(10,sizeof(char));
const char *alpha="abcdef";
ull h;
for (h=1;h<=10;++h) {
conv(h,alpha,a);
printf("%s\n", a);
}
}
Output is the same:
No, I didn't forget to reverse the strings, reversal was removed for code clarification.
For some reason speed is very important for me. I've tested the speed of executables compiled from the examples above and noticed that the one written n C++ using string
is more than 10 times less fast than the one written in C using char *
.
Each executable was compiled with -O2
flag of GCC. I was running tests using much bigger numbers to convert, such as 1e8 and more.
The question is: why is string
less fast than char *
in that case?
Your code snippets are not equivalent. *a='n'
does not append to the char
array. It changes the first char
in the array to 'n'
.
In C++, std::string
s should be preferred to char
arrays, because they're a lot easier to use, for example appending is done simply with the +=
operator.
Also they automatically manage their memory for you which char
arrays don't do. That being said, std::string
s are much less error prone than the manually managed char
arrays.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK