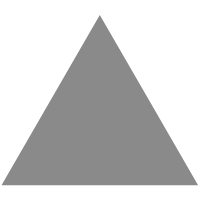
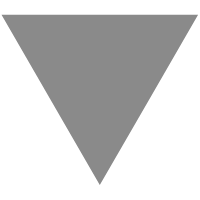
Partially Applied Functions in Scala
source link: https://blog.knoldus.com/partially-applied-functions-in-scala/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
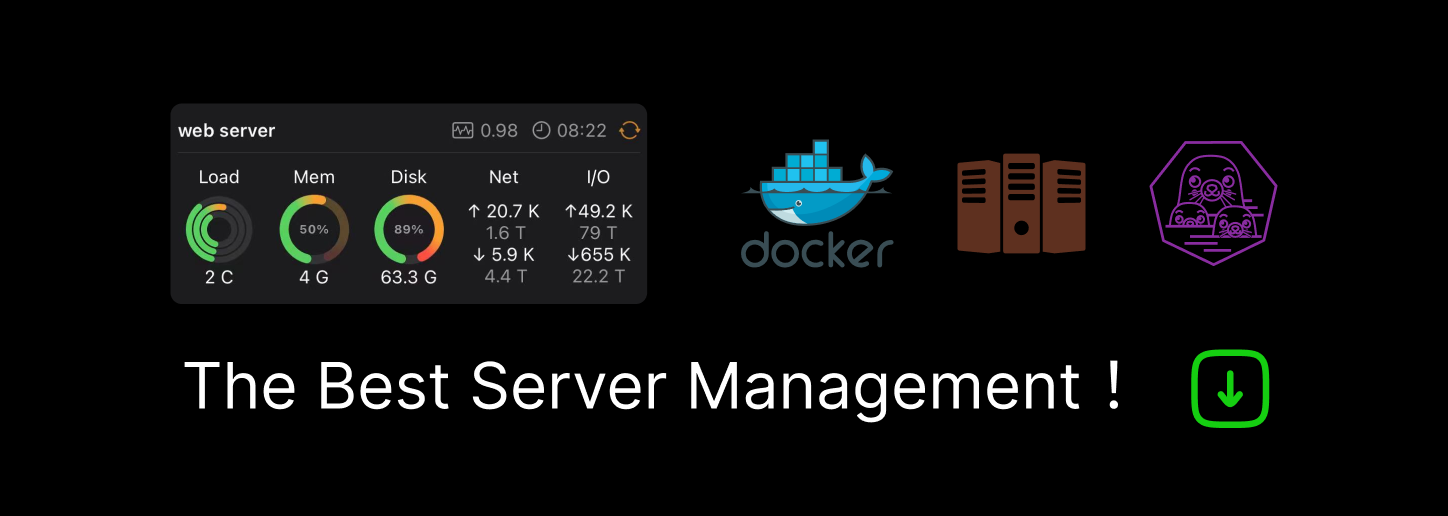
What is “partially applied”
Partially applied functions are an expression in which we do not supply all the arguments needed by the function. Instead, we supply some of the needed arguments only.
In Scala, whenever we invoke a function by passing all the needed arguments, we say that we have “applied” that function onto the arguments.
Now what we mean by the term partially applied is that we do not pass all the arguments. Rest of the arguments are passed later whenever required
Let’s understand the concept through a simple example where we transform a sum function into an increment function by partially applying it.
Creating a partially applied function
Suppose we create a simple method sum that just calculates the sum of 2 numbers like this:
def sum(num1: Int, num2: Int): Int = num1 + num2
The method simply adds the 2 numbers which we pass as arguments.
println(sum(3, 4)) // Output: 7
Problem Statement
We want to create a function that increments the value passed to it.
What we can do is we can modify the sum function such that one of its arguments is always 1 like this:
println(s"Incrementing 3: ${sum(3, 1)}") // Output: 4
println(s"Incrementing 4: ${sum(4, 1)}") // Output: 5
This solution works but is there a better way to do this….
Partially Applied Functions to the rescue ….
We create a partially applied function – increment such that we do not have to supply “1” as an argument again and again. This is how we create it:
val increment: Int => Int = sum(1, _: Int)
Here, we partially apply the function sum over argument “1”.
As seen from the type annotation we can see that increment is a function that takes an Int value and returns an Int value.
This is how we use the increment function:
println(increment(2)) // Output: 3
println(increment(3)) // Output: 4
How does this work?
Here we pass the 1st argument to the sum argument only but not the 2nd argument.
As we see that the type of increment is Int => Int. This essentially means that the Scala compiler generates a new function class whose apply method will take one argument.
When we pass an argument to increment its apply method invokes sum by first passing 1 and then the argument that it has received now. The equivalent code generated by the compiler is something like this:
val increment = new Function1[Int, Int] {
override def apply(v1: Int): Int = sum(1, v1)
}
Conclusion
In this blog we saw what are partially-applied functions, how do we create them and how it works internally.
Partially applied functions provide a nice clean way to create specialized functions from general methods. For example, in this blog, we create a specialized increment function from a general sum method.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK