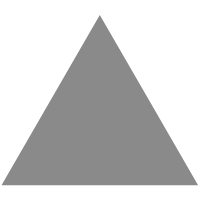
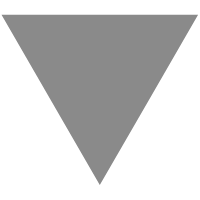
一文了解 Python 中的迭代器 #私藏项目实操分享#
source link: https://blog.51cto.com/yuzhou1su/5155521
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
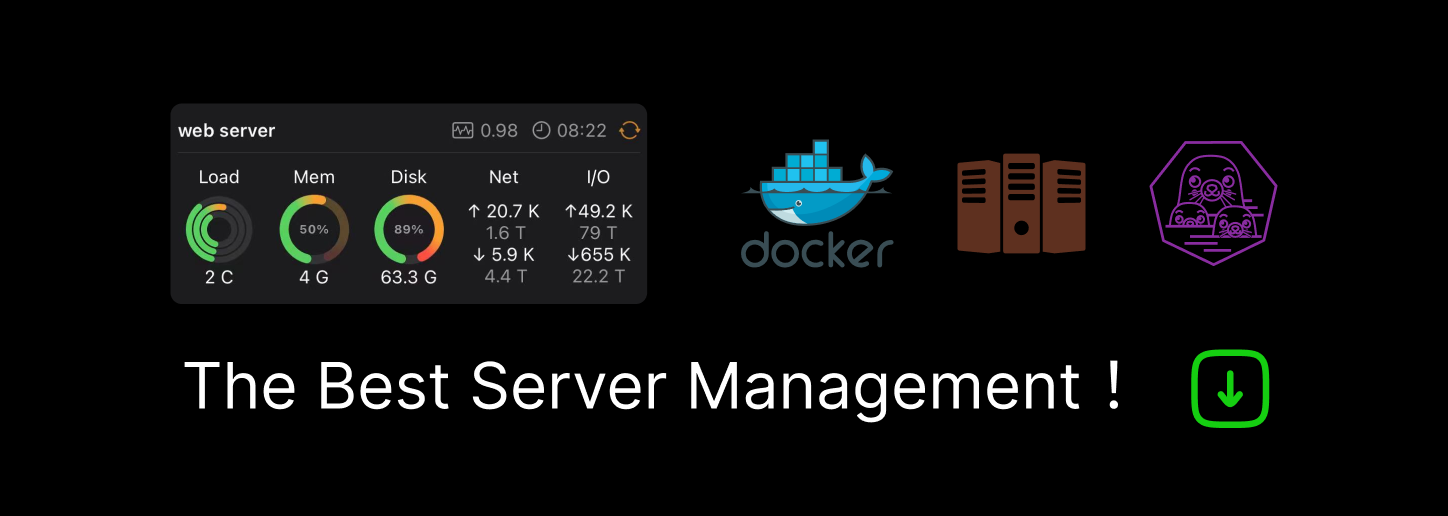
一文了解 Python 中的迭代器 #私藏项目实操分享#
推荐 原创Python 学习的人都知道,Python 中存在两种循环语句:while 和 for。for 循环可以用于 Python 中的任何序列,包括列表、元组、字符串。
...
2013 14 15926
>>>
>>> for x in (2021, 2022, 2023): print(x, end='->')
...
2021->2022->2023->
>>> for x in 'HelloWorld': print(x, end=' ')
...
H e l l o W o r l d
实际上,for 循环还能使用于任何可迭代对象。可迭代对象在 Python 中是新颖特别的概念,但实际上就是序列概念的通用化:如果对象时实际保存的序列,或者可以在迭代工具中(如 for 循环)一次产生一个结果的对象,就看做可迭代的。
迭代器:初探
Python 中迭代器无处不在。什么是迭代器?
Python 中的迭代器是一个对象,用于迭代列表、元组、字典和集合等可迭代对象。Python 迭代器对象必须实现两个特殊的方法:__iter__()
和__next__()
方法:
使用
__iter__()
方法初始化迭代器对象使用
__next__()
方法进行迭代。
通过迭代器进行迭代
iter()
函数依次调用 __iter__()
方法,返回一个迭代器。
我们使用 next()
函数手动遍历迭代器的所有项。当我们到达终点并且没有更多数据要返回时,它将引发 StopIteration
异常。下面是一个例子:
my_list = [2013, 14, 15926]
# get an iterator using iter()
my_iter = iter(my_list)
# iterate through it using next()
# Output: 2013
print(next(my_iter))
# Output: 14
print(next(my_iter))
# next(obj) is same as obj.__next__()
# Output: 15926
print(my_iter.__next__())
# This will raise error, no items left
next(my_iter)
依次执行上面的代码,输出如下:
14
15926
Traceback (most recent call last):
File "<string>", line 24, in <module>
next(my_iter)
StopIteration
一种更优雅的自动迭代方式是使用 for 循环。使用它,我们可以迭代任何可以返回迭代器的对象,例如列表、字符串、文件等。
... print(element)
...
2013
14
15926
迭代器 for 循环的工作
正如我们在上面的示例中看到的,for 循环能够自动遍历列表。
实际上 for 循环可以迭代任何可迭代对象。让我们仔细看看 for 循环是如何在 Python 中实际实现的。
# do something with element
实际实现为:
iter_obj = iter(iterable)
# infinite loop
while True:
try:
# get the next item
element = next(iter_obj)
print(element)
# do something with element
except StopIteration:
# if StopIteration is raised, break from loop
break
所以在内部,for 循环通过在可迭代对象上调用 iter()
创建一个迭代器对象 iter_obj
。具有讽刺意味的是,这个 for 循环实际上是一个无限的 while 循环。
在循环内部,它调用 next()
来获取下一个元素并使用该值执行 for 循环的主体。在所有项目耗尽后,StopIteration
被引发,内部捕获并结束循环。请注意,任何其他类型的异常都会通过。
构建自定义迭代器
在 Python 中从头开始构建迭代器很容易。我们只需要实现 __iter__()
和 __next__()
方法。
__iter__()
方法返回迭代器对象本身。如果需要,可以执行一些初始化。
__next__()
方法必须返回序列中的下一项。在到达终点时以及在随后的调用中,它必须引发 StopIteration
。
"""Class to implement an iterator
of powers of two"""
def __init__(self, max=0):
self.max = max
def __iter__(self):
self.n = 0
return self
def __next__(self):
if self.n <= self.max:
result = 2 ** self.n
self.n += 1
return result
else:
raise StopIteration
# create an object
numbers = PowTwo(3)
# create an iterable from the object
i = iter(numbers)
# Using next to get to the next iterator element
print(next(i))
print(next(i))
print(next(i))
print(next(i))
print(next(i))
输出结果:
2
4
8
Traceback (most recent call last):
File "/Users/yuzhou_1su/go/src/iterdemo.py", line 32, in <module>
print(next(i))
StopIteration
我们还可以使用 for 循环来迭代我们的迭代器类。
... print(i)
...
1
2
4
8
16
32
Python 无限迭代器
迭代器对象中的项目不必耗尽。可以有无限的迭代器(永远不会结束)。在处理此类迭代器时,我们必须小心。
这是一个演示无限迭代器的简单示例。
内置函数 iter()
可以使用两个参数调用,其中第一个参数必须是可调用对象(函数),第二个参数是哨兵。迭代器调用这个函数,直到返回的值等于哨兵。
0
>>> inf = iter(int,1)
>>> next(inf)
0
>>> next(inf)
0
我们可以看到 int()
函数总是返回 0。因此将它作为 iter(int,1)
传递将返回一个迭代器,该迭代器调用 int()
直到返回值等于 1。这永远不会发生,我们得到一个无限迭代器。
我们还可以构建自己的无限迭代器。理论上,以下迭代器将返回所有奇数:
"""Infinite iterator to return all
odd numbers"""
def __iter__(self):
self.num = 1
return self
def __next__(self):
num = self.num
self.num += 2
return num
>>> next(a)
1
>>> next(a)
3
>>> next(a)
5
>>> next(a)
7
在对这些类型的无限迭代器进行迭代时,请小心包含终止条件。如上所示,我们可以得到所有奇数,而无需将整个数字系统存储在内存中。理论上,我们可以在有限的内存中拥有无限的项目。
Python 迭代器的好处
使用迭代器的好处是可以节省资源。
代码减少。
代码冗余得到极大解决。
降低代码复杂度。
它为编码带来了更多的稳定性。
Python 的迭代器提供稳定和灵活的代码。迭代器和可迭代对象的区别:
Iterable 是一个可以迭代的对象。它在传递给
iter()
方法时生成一个迭代器。Iterator 是一个对象,用于使用
__next__()
方法对可迭代对象进行迭代。迭代器有 __next__()
方法,它返回对象的下一项。
请注意,每个迭代器也是一个可迭代的,但不是每个可迭代的都是一个迭代器。
例如,列表是可迭代的,但列表不是迭代器。可以使用函数 iter()
从可迭代对象创建迭代器。
为了实现这一点,对象的类需要一个方法 __iter__
,它返回一个迭代器,或者一个具有从 0 开始的顺序索引的 __getitem__
方法。但其本质也是实现了 __iter__
方法。
参考资料:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK