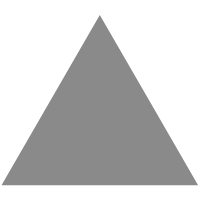
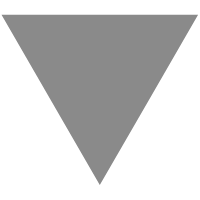
Embody System Commands in Rust Programming
source link: https://blog.knoldus.com/embody-system-commands-in-rust-programming/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
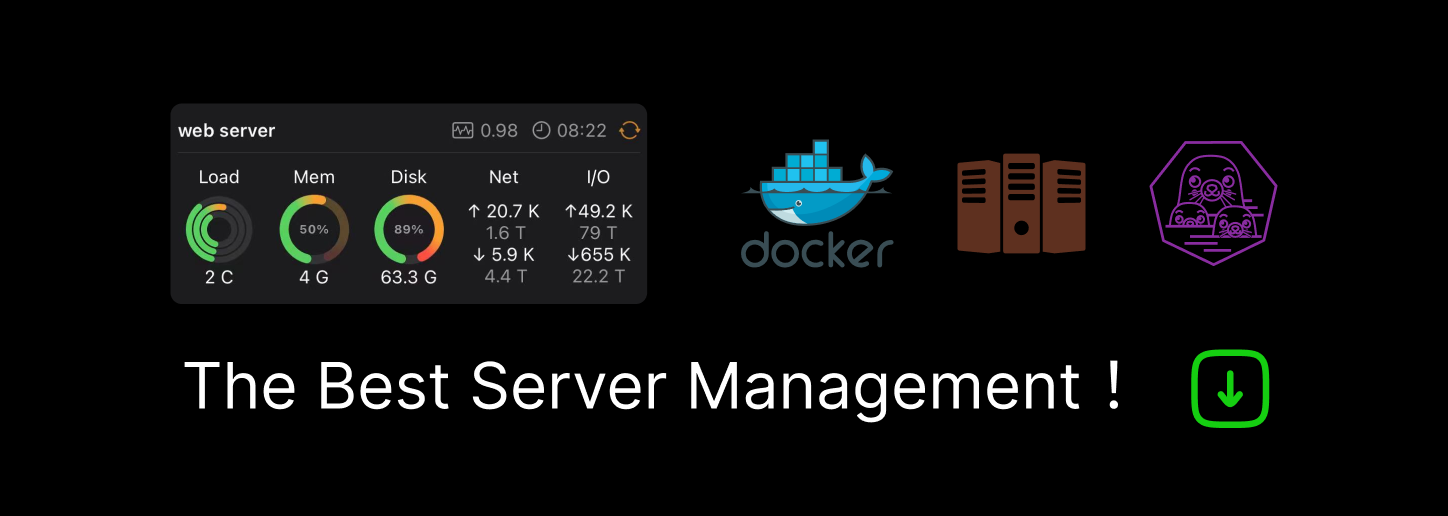
Embody System Commands in Rust Programming
Reading Time: 2 minutes
System Commands helps users to communicate with the Operating System and with the help of that commands user can operate the whole Operating System(OS). System commands are handled by the shell or command interpreter of the Operating System.
In this blog, I will show you how to execute System Commands through Rust Programming.
We can execute system commands through Rust program by leveraging the features of the Standard library. The standard library allows us to execute system commands through our program by using std::process::Command.
use std::process::Command;
The above line provides us all the features of Command structure declared in the process module of the standard library.
Now we are able to use all the methods of the Command structure.
use std::process::Command;
fn main() {
let result: Output = Command::new("cat")
.arg("file1")
.output()
.expect("failed to execute command");
println!("status: {}", result.status);
println!("stdout: {}", String::from_utf8_lossy(&result.stdout));
println!("stderr: {}", String::from_utf8_lossy(&result.stderr));
}
The above code shows the content of the file called file1 by executing cat command in the Rust program.
Here we use:
new():- this function is used to create a command.
arg():- this is used to add the arguments with the commands
output():- it is used to spawn(function that loads and executes a new child process) a process and reutns the result of output
expect():- it unwraps the result if [Ok] and panics if [Err]
The output of the above code:
$ cargo run
Finished dev [unoptimized + debuginfo] target(s) in 0.00s
Running `target/debug/system-commands-in-rust`
status: exit code: 0
stdout: Welcome to Knoldus Inc.:- World's largest pure-play Scala + Spark service company.
stderr:
There are three ways to spawn a child process:
- output():- it runs the program and returns Result of Output(Result<Output>) after completion of the child process.
- status():- it runs the program and returns the Result of ExitStatus(Result<ExitStatus>)after completion of the child process.
- spawn():- it runs the program and returns the result of Child(Result<Child>) and it doesn’t wait for completion of a child process[asynchronus] and by default stdin, stdout and stderr are inherited from the parent.
There are two ways to pass arguments in the command:
1. pass multiple arguments with arg()
2. pass multiple arguments with args()
Pass multiple arguments with arg():
use std::process::Command;
fn main() {
let result = Command::new("cp")
.arg("file1")
.arg("file2")
.spawn()
.expect("failed to execute command");
println!("Output is : {:?}", result);
}
Above code shows the use of cp command, with two arguments source and destination by executing a command as a Child process(spawn()).
The output is:
$ cargo run
Compiling system-commands-in-rust v0.1.0 (/home/knoldus/git/knoldus/system-commands-in-rust)
Finished dev [unoptimized + debuginfo] target(s) in 0.41s
Running `target/debug/system-commands-in-rust`
Output is : Child { stdin: None, stdout: None, stderr: None }
Pass multiple arguments with args():
use std::process::Command;
fn main() {
let result = Command::new("cp")
.args(&["file1", "file2"])
.status()
.expect("failed to execute command");
println!("Output is : {:?}", result);
}
Above code is same as previous one but here we are using args() to pass multiple arguments together and using status() for spawning a child process.
The output is:
$ cargo run
Compiling system-commands-in-rust v0.1.0 (/home/knoldus/git/knoldus/system-commands-in-rust)
Finished dev [unoptimized + debuginfo] target(s) in 0.44s
Running `target/debug/system-commands-in-rust`
Output is : ExitStatus(ExitStatus(0))
Hope you all will understand how to execute system commands through Rust programming.
Thanks for reading!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK