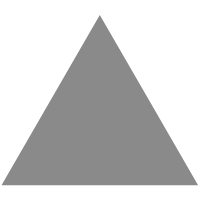
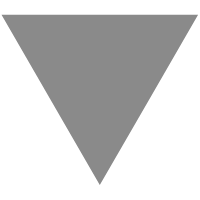
React, Vue and Svelte: Comparing the basics - Part 5
source link: https://dev.to/ccreusat/react-vue-and-svelte-comparing-the-basics-part-5-25d
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
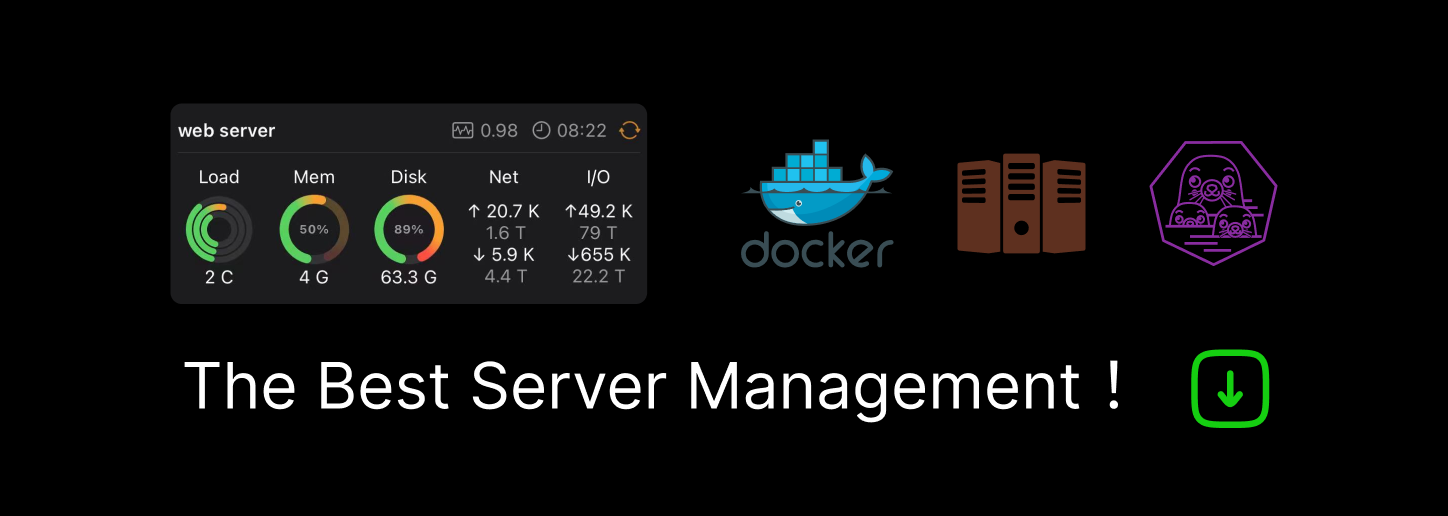
Conditional rendering in ...
React
import { useState } from 'react';
const App = () => {
const [isVisible, setIsVisible] = useState<boolean>(true);
return (
<div>{isVisible ? <p>I'm right here!</p> : <p>I'm invisible!</p>}</div>
);
};
Enter fullscreen mode
Exit fullscreen mode
<script setup lang="ts">
import { ref } from 'vue'
const isVisible:Boolean = ref(true)
</script>
<template>
<p v-if="isVisible">I'm right here!</p>
<p v-else>I'm invisible!</p>
</template>
Enter fullscreen mode
Exit fullscreen mode
Svelte
<script lang="ts">
let isVisible:boolean = true;
</script>
{#if isVisible}
<p>I'm right here!</p>
{:else}
<p>I'm invisible!</p>
{/if}
Enter fullscreen mode
Exit fullscreen mode
Data rendering in...
React
import { useState } from "react";
const [jobs, setJobs] = useState([
{id: 1, title: "Frontend Developer"},
{id: 2, title: "Backend Developer"},
{id: 3, title: "Fullstack Developer"}
]);
<ul>
{
jobs.map((job) => (
<li>{job.title}</li>
))
}
</ul>
Enter fullscreen mode
Exit fullscreen mode
import { ref } from "vue";
// `reactive` could be used instead of `ref`
const jobs = ref([
{id: 1, title: "Frontend Developer"},
{id: 2, title: "Backend Developer"},
{id: 3, title: "Fullstack Developer"}
]);
<template>
<ul>
<li v-for="job in jobs" :key="job.id">
{{ job.title }}
</li>
</ul>
</template>
Enter fullscreen mode
Exit fullscreen mode
Svelte
const jobs = [
{id: 1, title: "Frontend Developer"},
{id: 2, title: "Backend Developer"},
{id: 3, title: "Fullstack Developer"}
];
<ul>
{#each jobs as {id, title}, i}
<li id={id}>
{title}
</li>
{/each}
</ul>
Enter fullscreen mode
Exit fullscreen mode
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK