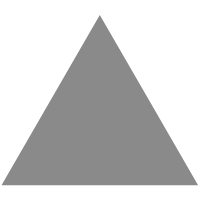
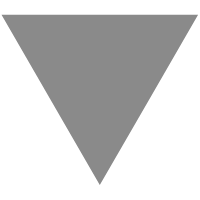
Remove Last Character from String in C++
source link: https://thispointer.com/remove-last-character-from-string-in-c/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
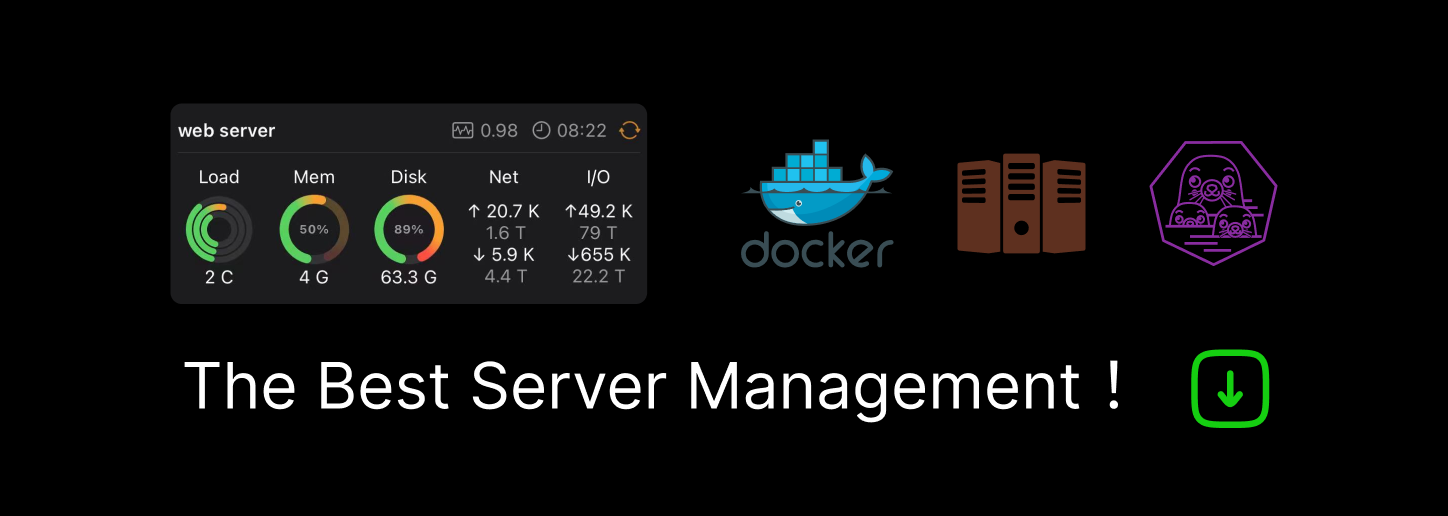
This article will discuss different ways to delete the last character from a string in C++.
Suppose we have a string like this,
"The tablet"
We need to delete the last character from this string. The final string should be like this.
"The table"
Let’s see how to do that.
Remove the last character from the string using erase()
In C++, the string class provides a function erase(), and in one of its overloaded versions, it takes two parameters i.e.
Advertisements
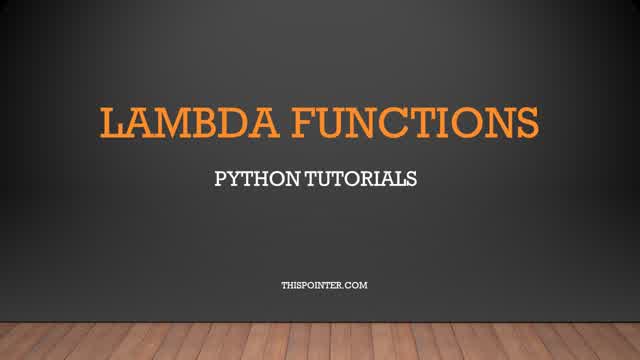
string& erase(size_t pos = 0, size_t len = npos);
Parameters:
- pos: An index position.
- len: The number of elements to delete. If not provided, then deletes the characters till end from given index position.
It deletes the specified number (len) of characters from the string, starting from the given index position (pos).
We can use this to delete the last character from the string by passing the starting index position as N-1, where N is the size of string. For example,
#include <iostream> int main() { std::string sampleStr = "The tablet"; // Remove last character from string sampleStr.erase(sampleStr.size() - 1); std::cout<<sampleStr << std::endl; return 0; }
Output:
The table
It deleted the last character from the string in C++. In the erase() function we passed the position of last character as the starting index position and used the default value of len parameter i.e. npos. Therefore, it deleted only the last character.
Remove the last character from the string using pop_back()
In C++, the string class provides a function pop_back(), and it removes the last character from string, if string is non-empty. Let’s use this to delete the last character from the string,
#include <iostream> int main() { std::string sampleStr = "The tablet"; // Remove last character from string sampleStr.pop_back(); std::cout<<sampleStr << std::endl; return 0; }
Output:
The table
It deleted the last character from the string in C++.
Remove the last character from the string using substr()
In C++, the string class provides a function substr(). It returns a copy of the sub-string selected based on index positions.
Syntax of string::substr()
string substr(size_t pos = 0, size_t len = npos) const;
It accepts two parameters,
- pos: The starting index position (pos). The Position from which it will start copying the characters.
- len: The Number of characters to be selected in the sub-string.
Returns:
- It returns a newly constructed string containing the specified characters from the calling string object.
We can use this to remove the last character from the string. We need to pass the 0 as the first argument and N-1 as the second argument in the substr() function. Here, N is the size of the string. It will return a copy of the substring containing the characters from index position 0 till the second last character. For example,
#include <iostream> int main() { std::string sampleStr = "The tablet"; // Remove last character from string sampleStr = sampleStr.substr(0, sampleStr.size() - 1); std::cout<<sampleStr << std::endl; return 0; }
Output:
The table
It deleted the last character from the string in C++.
Summary:
We learned three different ways to delete the last character from a string in C++.
Do you want to Learn Modern C++ from best?
We have curated a list of Best C++ Courses, that will teach you the cutting edge Modern C++ from the absolute beginning to advanced level. It will also introduce to you the word of Smart Pointers, Move semantics, Rvalue, Lambda function, auto, Variadic template, range based for loops, Multi-threading and many other latest features of C++ i.e. from C++11 to C++20.
Check Detailed Reviews of Best Modern C++ Courses
Remember, C++ requires a lot of patience, persistence, and practice. So, start learning today.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK