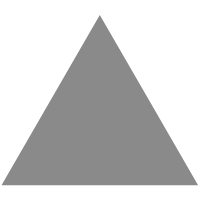
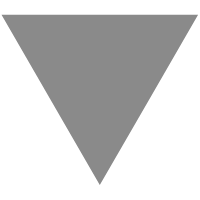
(Minimum Window Substring)
source link: https://tianrunhe.wordpress.com/2013/03/23/minimum-window-substring/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
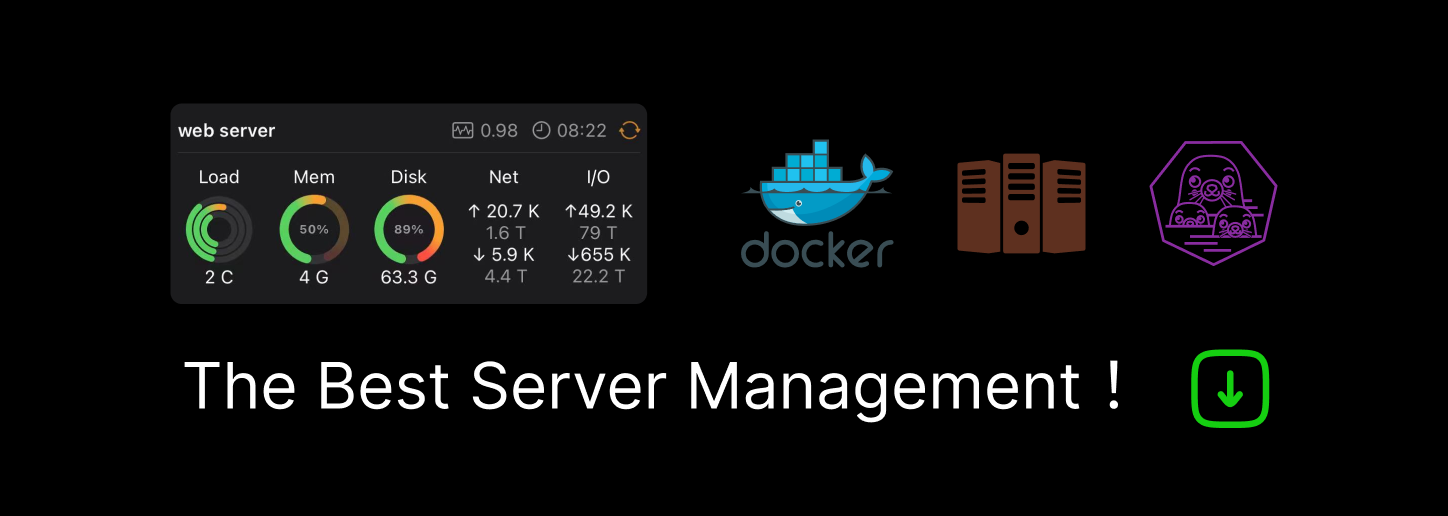
(Minimum Window Substring)
23 Mar 2013 Leave a comment
by Runhe Tian in LeetCode Tags: Array, C++, Java, String
Given a string S and a string T, find the minimum window in S which will contain all the characters in T in complexity
.
For example,
S = “ADOBECODEBANC”
T = “ABC”
Minimum window is “BANC”.
Note:
If there is no such window in S that covers all characters in T, return the emtpy string “”.
If there are multiple such windows, you are guaranteed that there will always be only one unique minimum window in S.
Thoughts:
The idea is from here. I try to rephrase it a little bit here. The general idea is that we find a window first, not necessarily the minimum, but it’s the first one we could find, traveling from the beginning of S. We could easily do this by keeping a count of the target characters we have found. After finding an candidate solution, we try to optimize it. We do this by going forward in S and trying to see if we could replace the first character of our candidate. If we find one, we then find a new candidate and we update our knowledge about the minimum. We keep doing this until we reach the end of S. For the giving example:
- We start with our very first window: “ADOBEC”, windowSize = 6. We now have “A”:1, “B”:1, “C”:1
- We skip the following character “ODE” since none of them is in our target T. We then see another “B” so we update “B”:2. Our candidate solution starts with an “A” so getting another “B” cannot make us a “trade”.
- We then see another “A” so we update “A”:2. Now we have two “A”s and we know we only need 1. If we keep the new position of this “A” and disregard the old one, we could move forward of our starting position of window. We move from A->D->O->B. Can we keep moving? Yes, since we know we have 2 “B”s so we can also disregard this one. So keep moving until we hit “C”: we only have 1 “C” so we have to stop. Therefore, we have a new candidate solution, “CODEBA”. Our new map is updated to “A”:1, “B”:1, “C”:1.
- We skip the next “N” and we arrive at “C”. Now we have two “C”s so we can move forward the starting position of last candidate: we move along this path C->O->D->E until we hit “B”. We only have one “B” so we have to stop. We have yet another new candidate, “BANC”.
- We have hit the end of S so we just output our best candidate, which is “BANC”.
Code (Java):
public
class
Solution {
public
String minWindow(String S, String T) {
int
[] needToFind =
new
int
[
256
];
int
[] hasFound =
new
int
[
256
];
for
(
int
i =
0
; i < T.length(); ++i) {
needToFind[T.charAt(i)]++;
}
int
count =
0
;
int
minWindowSize = Integer.MAX_VALUE;
int
start =
0
, end =
0
;
String window =
""
;
for
(; end < S.length(); end++) {
if
(needToFind[S.charAt(end)] ==
0
)
continue
;
char
c = S.charAt(end);
hasFound[c]++;
if
(hasFound[c] <= needToFind[c]) {
count++;
}
if
(count == T.length()) {
while
(needToFind[S.charAt(start)] ==
0
||
hasFound[S.charAt(start)] > needToFind[S.charAt(start)]) {
if
(hasFound[S.charAt(start)] > needToFind[S.charAt(start)])
hasFound[S.charAt(start)]--;
start++;
}
if
(end - start +
1
< minWindowSize) {
minWindowSize = end - start +
1
;
window = S.substring(start, end +
1
);
}
}
}
return
window;
}
}
Code (C++):
class
Solution {
public
:
string minWindow(string S, string T) {
int
needToFind[256] = {0};
int
hasFound[256] = {0};
for
(
int
i = 0; i < T.size(); ++i) {
needToFind[T[i]]++;
}
int
count = 0;
int
minWindowSize = INT_MAX;
int
start = 0, end = 0;
string window;
for
(; end < S.size(); end++) {
if
(needToFind[S[end]] == 0)
continue
;
hasFound[S[end]]++;
if
(hasFound[S[end]] <= needToFind[S[end]]) {
count++;
}
if
(count == T.size()) {
while
(needToFind[S[start]] == 0 ||
hasFound[S[start]] > needToFind[S[start]]) {
if
(hasFound[S[start]] > needToFind[S[start]])
hasFound[S[start]]--;
start++;
}
if
(end - start + 1 < minWindowSize) {
minWindowSize = end - start + 1;
window = S.substr(start, minWindowSize);
}
}
}
return
window;
}
};
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK