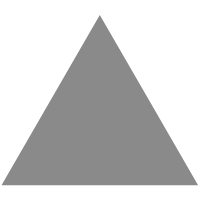
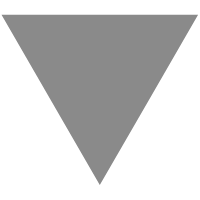
Retrieve a window handle (HWND)
source link: https://docs.microsoft.com/en-us/windows/apps/develop/ui-input/retrieve-hwnd?WT_mc_id=DOP-MVP-4025064
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
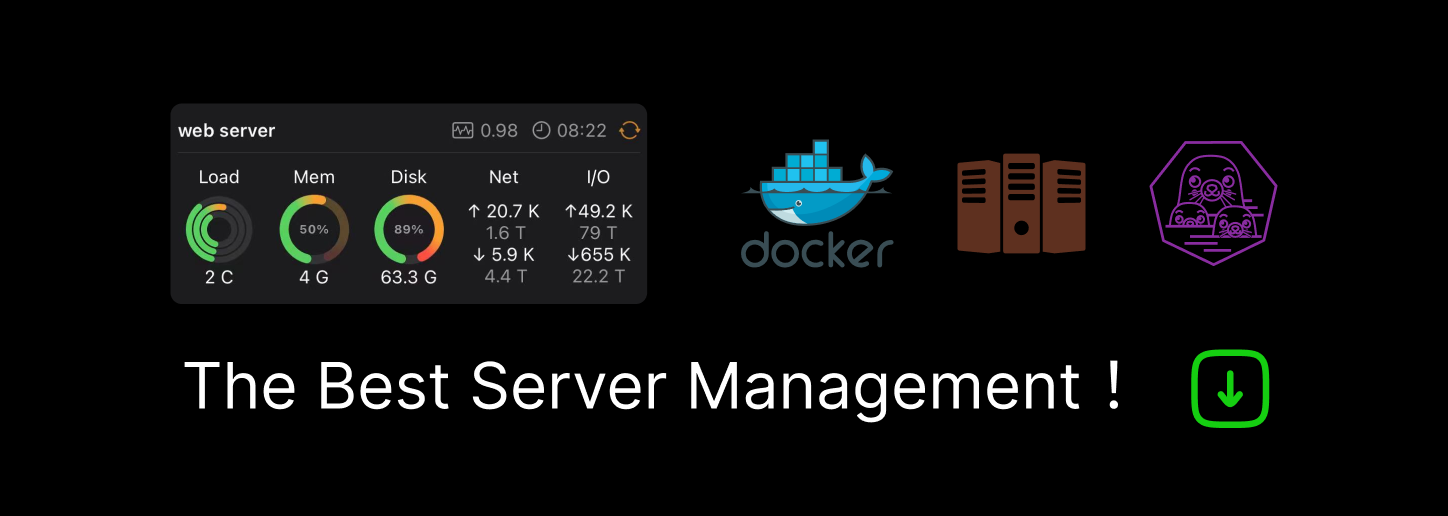
Retrieve a window handle (HWND)
- Article
- 03/08/2022
- 2 minutes to read
This topic shows you how, in a desktop app, to retrieve the window handle for a window. The scope covers Windows UI Library (WinUI) 3, Windows Presentation Foundation (WPF), and Windows Forms (WinForms) apps; code examples are presented in C# and C++/WinRT.
The development and UI frameworks listed above are (behind the scenes) built on the Win32 API. In Win32, a window object is identified by a value known as a window handle. And the type of a window handle is an HWND (although it surfaces in C# as an IntPtr). In any case, you'll hear the term HWND used as a shorthand for window handle.
There are several reasons to retrieve the HWND for a window in your WinUI 3, WPF, or WinForms desktop app. One example is to use the HWND to interoperate with certain Windows Runtime (WinRT) objects that depend on a CoreWindow to display a user-interface (UI). For more info, see Display WinRT UI objects that depend on CoreWindow.
WinUI 3 with C#
The C# code below shows how to retrieve the window handle (HWND) for a WinUI 3 Window object. This example calls the GetWindowHandle method on the WinRT.Interop.WindowNative C# interop class. For more info about the C# interop classes, see Call WinRT COM interop interfaces from a .NET 5+ app.
// MainWindow.xaml.cs
private async void myButton_Click(object sender, RoutedEventArgs e)
{
// Retrieve the window handle (HWND) of the current WinUI 3 window.
var hWnd = WinRT.Interop.WindowNative.GetWindowHandle(this);
}
WinUI 3 with C++
The C++/WinRT code below shows how to retrieve the window handle (HWND) for a WinUI 3 Window object. This example calls the IWindowNative::get_WindowHandle method.
// pch.h
...
#include <microsoft.ui.xaml.window.h>
// MainWindow.xaml.cpp
void MainWindow::myButton_Click(IInspectable const&, RoutedEventArgs const&)
{
// Retrieve the window handle (HWND) of the current WinUI 3 window.
auto windowNative{ this->try_as<::IWindowNative>() };
winrt::check_bool(windowNative);
HWND hWnd{ 0 };
windowNative->get_WindowHandle(&hWnd);
}
WPF with C#
The C# code below shows how to retrieve the window handle (HWND) for a WPF window object. This example uses the WindowInteropHelper class.
// MainWindow.xaml.cs
private void Button_Click(object sender, RoutedEventArgs e)
{
var wih = new System.Windows.Interop.WindowInteropHelper(this);
var hWnd = wih.Handle;
}
WinForms with C#
The C# code below shows how to retrieve the window handle (HWND) for a WinForms form object. This example uses the NativeWindow.Handle property.
// Form1.cs
private void button1_Click(object sender, EventArgs e)
{
var hWnd = this.Handle;
}
Related topics
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK