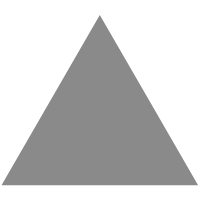
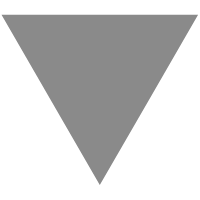
How to display or hide options for & lt; & Gt; S depending on what is cl...
source link: https://www.codesd.com/item/how-to-display-or-hide-options-for-s-depending-on-what-is-clicked-on-a-group-of-external-radio-buttons.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
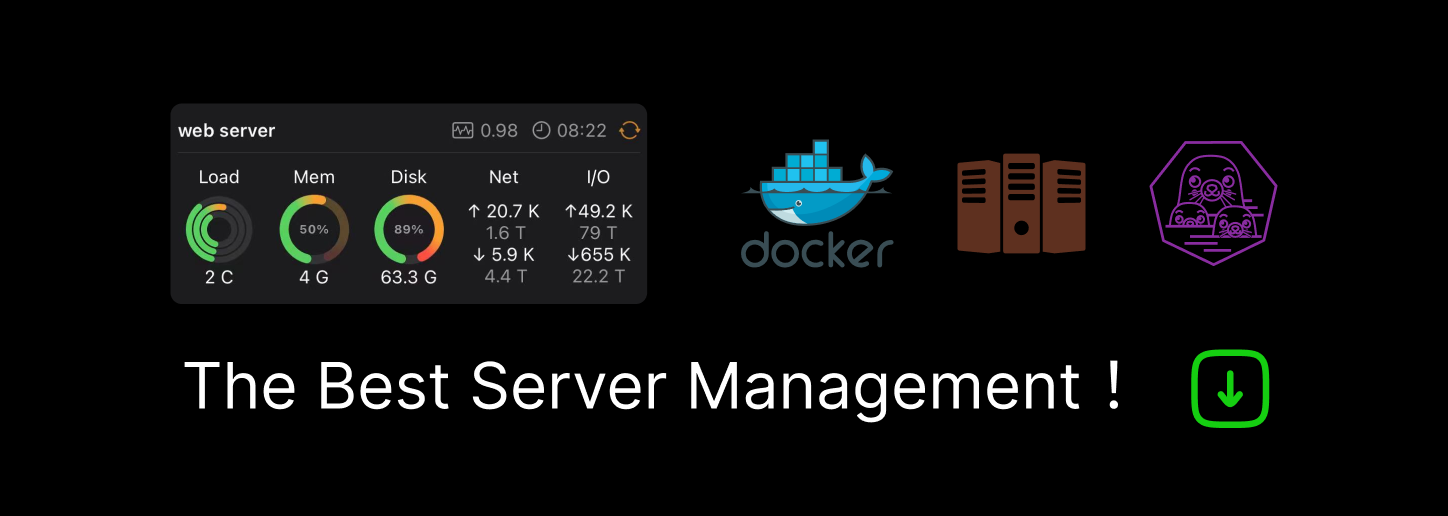
How to display or hide options for & lt; & Gt; S depending on what is clicked on a group of external radio buttons?
I am building a form using jQuery that dynamically adds select fields with incrementing ids when the user clicks "Add More". I need to be able to show or hide certain options based on what the user selected earlier in the form.
When the user clicks "A" from the radio group, then "Div A1" will be hidden. When the user clicks "B", "Div B1" will be hidden, but "Div A1" will be shown, etc. etc.
What's the best way to go about doing that?
$(function() {
$('.show_hide_div_list').hide();
$('#hide_div_A').click(function() {
$('.show_hide_div_list').show();
});
$('#hide_div_B').click(function() {
$('.show_hide_div_list').show();
});
$('#hide_div_C').click(function() {
$('.show_hide_div_list').show();
});
$('#hide_div_D').click(function() {
$('.show_hide_div_list').show();
});
var show_hide_div_max_fields = 36;
var show_hide_div_x = 0;
$('#show_hide_div_add').click(function(e) {
e.preventDefault();
if (show_hide_div_x < show_hide_div_max_fields) {
show_hide_div_x++;
var inps = $('#show_hide_div_wrapper >div:last').data('count') + 1 || 1;
$('#show_hide_div_wrapper').append('<div class="show_hide_div" data-count="' + inps + '"><select id="show_hide_div"><option value="div_a" id="div_a' + inps + '">Div A' + inps + '</option><option value="div_b' + inps + '" id="div_b' + inps + '">Div B' + inps + '</option><option value="div_c' + inps + '" id="div_c' + inps + '">Div C' + inps + '</option><option value="div_d' + inps + '" id="div_d' + inps + '">Div D' + inps + '</option></select><a class=remove>Remove</a><br><br></div>');
}
$('#show_hide_div_wrapper').on('click', 'a.remove', function() {
var inps = $('#show_hide_div_wrapper > div:last').data('count') - 1 || 1;
show_hide_div_x--;
$(this).closest('div').remove();
});
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<table>
<tr class="show_hide_div">
<td style="vertical-align:top;">
Select which options you want to hide
</td>
<td>
<label>A</label>
<input type="radio" name="hide_div" id="hide_div_A" value="A">
<br>
<label>B</label>
<input type="radio" name="hide_div" id="hide_div_B" value="B">
<br>
<label>C</label>
<input type="radio" name="hide_div" id="hide_div_C" value="C">
<br>
<label>D</label>
<input type="radio" name="hide_div" id="hide_div_D" value="D">
</td>
</tr>
<tr class="show_hide_div_list" style="line-height:1em;">
<td>
</td>
<td>
<span id="show_hide_div_wrapper">
</span>
</td>
</tr>
<tr class="show_hide_div_list">
<td>
</td>
<td>
<button id="show_hide_div_add">Add More</button>
</td>
</tr>
</table>
$(function() {
$('.show_hide_div_list').hide();
var divArray = ['A', 'B', 'C', 'D']
divArray.forEach(function (item) {
$('#hide_div_' + item).click(function () {
$('.show_hide_div_list').show()
})
});
var show_hide_div_max_fields = 36;
var show_hide_div_x = 0;
$('#show_hide_div_add').click(function(e) {
e.preventDefault();
var optionArray = ['A', 'B', 'C', 'D'];
optionArray.forEach(function (item) {
if ($('#hide_div_' + item).prop('checked')) {
if (show_hide_div_x < show_hide_div_max_fields) {
show_hide_div_x++;
var inps = $('#show_hide_div_wrapper >div:last').data('count') + 1 || 1;
var div = $('<div>').addClass('show_hide_div').prop('data-count', inps);
var select = $('<select>').prop('id', 'show_hide_div');
optionArray.forEach(function (_item) {
if (_item != item) {
var option = $('<option>').prop('id', 'div_' + _item + inps).text('Div ' + _item);
select.append(option);
}
})
div.append(select).append('<a class=remove>Remove</a><br><br>');
$('#show_hide_div_wrapper').append(div);
}
}
});
$('#show_hide_div_wrapper').on('click', 'a.remove', function() {
var inps = $('#show_hide_div_wrapper > div:last').data('count') - 1 || 1;
show_hide_div_x--;
$(this).closest('div').remove();
});
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<table>
<tr class="show_hide_div">
<td style="vertical-align:top;">
Select which options you want to hide
</td>
<td>
<label>A</label>
<input type="radio" name="hide_div" id="hide_div_A" value="A">
<br>
<label>B</label>
<input type="radio" name="hide_div" id="hide_div_B" value="B">
<br>
<label>C</label>
<input type="radio" name="hide_div" id="hide_div_C" value="C">
<br>
<label>D</label>
<input type="radio" name="hide_div" id="hide_div_D" value="D">
</td>
</tr>
<tr class="show_hide_div_list" style="line-height:1em;">
<td>
</td>
<td>
<span id="show_hide_div_wrapper">
</span>
</td>
</tr>
<tr class="show_hide_div_list">
<td>
</td>
<td>
<button id="show_hide_div_add">Add More</button>
</td>
</tr>
</table>
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK