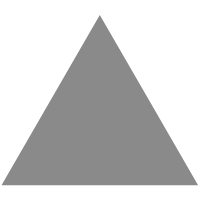
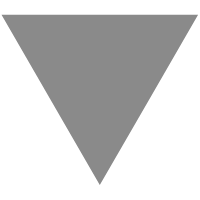
Mocha 复用测试用例
source link: https://blog.andiedie.cn/posts/cc67/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
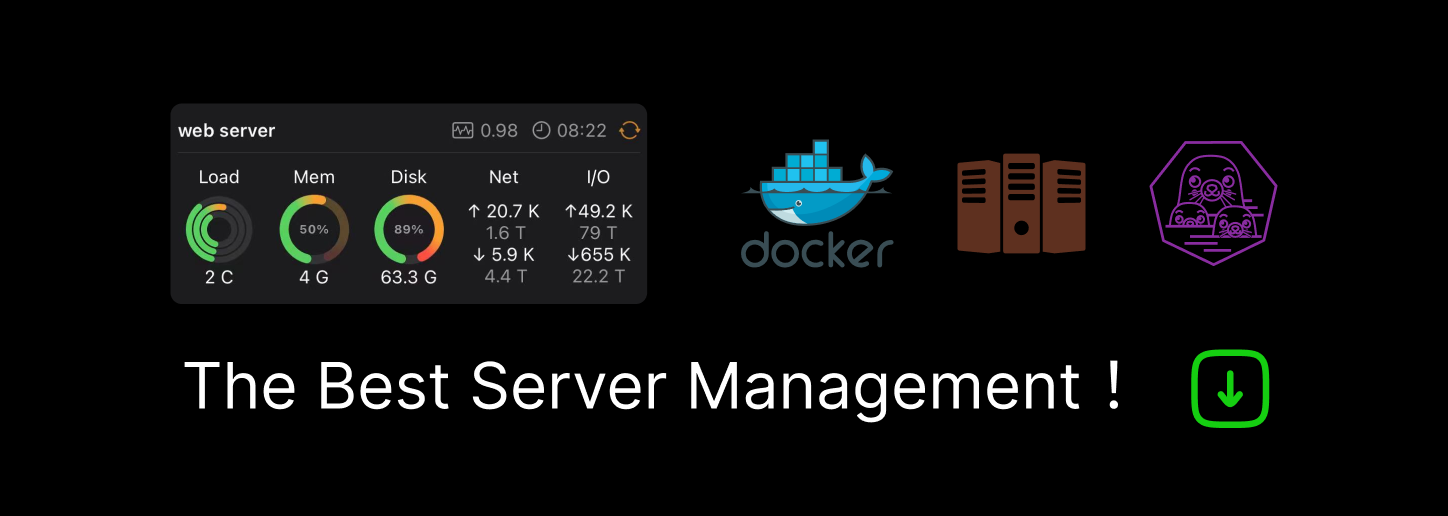
Mocha 复用测试用例
在使用 Mocha 进行单元测试时,经常会有复用测试用例的需求。例如定义了一组测试用例后,希望在多种环境下都使用这组用例进行测试。
1. 测试环境
首先简单地搭建一个测试环境。
创建项目:
mkdir mocha-reuse
cd mocha-reuse
yarn init -y
yarn add -D mocha
项目目录结构如下:
index.js
test
index.test.js
其中 index.js
内容如下:
// index.js
module.exports = class Human {
constructor(name, age) {
if (typeof name === 'string') {
this.name = name;
} else if (name instanceof Buffer) {
this.name = name.toString();
} else {
throw TypeError('Name can only be string or buffer');
}
if (typeof age === 'number') {
this.age = age;
} else if (typeof age === 'string') {
this.age = Number(age);
} else {
throw TypeError('Age can only be number or string');
}
}
toString() {
return `${this.name}: ${this.age}`;
}
}
逻辑非常简单,Human
类构造时需要两个参数,name
支持 string
和 Buffer
;age
支持 number
和 string
。
测试内容是,构造一个 Human
实例后测试 name
、age
和 toString()
是否正确。
2. 无复用版本
为了提高测试覆盖率,必须使用每一种可能的参数组合实例化 Human
。
// test/index.test.js
const assert = require('assert');
const Human = require('../index');
describe('Test with string name and number age', () => {
let human;
before(() => {
human = new Human('A', 1);
});
it('Check name', () => {
assert(human.name === 'A');
});
it('Check age', () => {
assert(human.age === 1);
});
it('Check toString', () => {
assert(human.toString() === 'A: 1');
});
});
describe('Test with buffer name and number age', () => {
let human;
before(() => {
human = new Human(Buffer.from('A'), 1);
});
it('Check name', () => {
assert(human.name === 'A');
});
it('Check age', () => {
assert(human.age === 1);
});
it('Check toString', () => {
assert(human.toString() === 'A: 1');
});
});
describe('Test with string name and string age', () => {
let human;
before(() => {
human = new Human('A', '1');
});
it('Check name', () => {
assert(human.name === 'A');
});
it('Check age', () => {
assert(human.age === 1);
});
it('Check toString', () => {
assert(human.toString() === 'A: 1');
});
});
describe('Test with buffer name and string age', () => {
let human;
before(() => {
human = new Human(Buffer.from('A'), '1');
});
it('Check name', () => {
assert(human.name === 'A');
});
it('Check age', () => {
assert(human.age === 1);
});
it('Check toString', () => {
assert(human.toString() === 'A: 1');
});
});
可以看到,除了初始化不同之外,所有的测试样例都是一样的,因此我们必须想办法复用测试用例
3. 复用测试用例
在 test
目录下创建新的文件 share.js
,将复用的用例放在里面:
const assert = require('assert');
module.exports = function () {
it('Check name', function () {
assert(this.human.name === 'A');
});
it('Check age', function () {
assert(this.human.age === 1);
});
it('Check toString', function () {
assert(this.human.toString() === 'A: 1');
});
};
此时目录结构变为:
index.js
test
index.test.js
share.js
index.test.js
变为:
const Human = require('../index');
const check = require('./share');
describe('Test with string name and number age', () => {
before(function () {
this.human = new Human('A', 1);
});
check();
});
describe('Test with buffer name and number age', () => {
before(function () {
this.human = new Human(Buffer.from('A'), 1);
});
check();
});
describe('Test with string name and string age', () => {
before(function () {
this.human = new Human('A', '1');
});
check();
});
describe('Test with buffer name and string age', () => {
before(function () {
this.human = new Human(Buffer.from('A'), '1');
});
check();
});
要注意的是,这里在所有涉及 this
的函数上,不要使用箭头函数。
- 本文作者: Andie
- 本文链接: http://blog.andiedie.cn/posts/cc67/
- 版权声明: 本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK