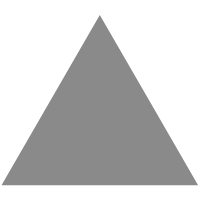
4
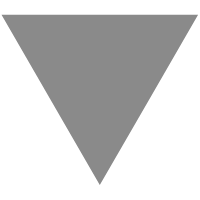
zmq超时处理设置超时时间
source link: https://viencoding.com/article/200
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
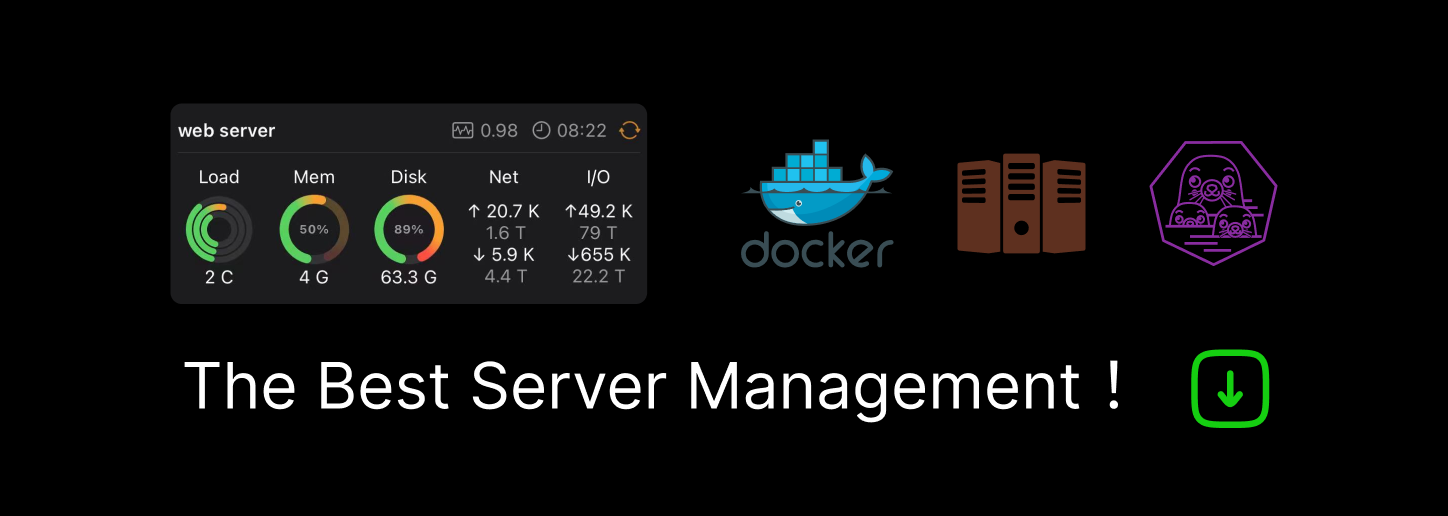
zmq客户端send服务端,如果zmq服务端不响应或长时间不返回,那么就会处于一直等待的状态,且阻塞在这个地方,使用poll设置zmq客户端等待时间,超时重试或者重新开启一个socket
zmq设置超时时间,并且超时重试
import zmq
import time
context = zmq.Context()
socket = context.socket(zmq.REQ)
socket.connect("tcp://localhost:5555")
poll = zmq.Poller()
poll.register(socket, zmq.POLLIN)
while True:
socket.send_string('hello')
while True:
socks = dict(poll.poll(3000))
if socks.get(socket) == zmq.POLLIN:
ret = socket.recv()
print(ret)
break
else:
socket.setsockopt(zmq.LINGER, 0)
socket.close()
poll.unregister(socket)
socket = context.socket(zmq.REQ)
socket.connect("tcp://localhost:5555")
poll.register(socket, zmq.POLLIN)
socket.send_string('hello')
print('confused')
socket.close()
context.term():
zmq超时机制封装成类
#!/usr/bin/python
# -*- coding: UTF-8 -*-
import zmq
__author__ = 'Vien'
class Message(object):
def __init__(self, ip='localhost', port='5555'):
self.context = zmq.Context()
self.socket = self.context.socket(zmq.REQ)
self.address = "tcp://{}:{}".format(ip, port)
self.socket.connect(self.address)
self.poller = zmq.Poller()
self.poller.register(self.socket, zmq.POLLIN)
def send(self, msg):
self.socket.send(msg)
if self.poller.poll(1000):
resp = self.socket.recv()
else:
self.socket.setsockopt(zmq.LINGER, 0)
self.socket.close()
self.poller.unregister(self.socket)
self.socket = self.context.socket(zmq.REQ)
self.socket.connect(self.address)
self.poller.register(self.socket, zmq.POLLIN)
raise Exception('Server no response, trying reconnect.')
return resp
只需要new一个对象,调用send方法即可。超时抛出异常,可以捕获异常进行重试或者其他操作。
msg = Message()
msg.send('Hello world')
import time
msg = Message()
while 1:
try:
msg.send('Hello world')
except Exception as e:
print('try again')
time.sleep(3)
viencoding.com版权所有,允许转载,但转载请注明出处和原文链接: https://viencoding.com/article/200
欢迎小伙伴们在下方评论区留言 ~ O(∩_∩)O
文章对我有帮助, 点此请博主吃包辣条 ~ O(∩_∩)O
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK