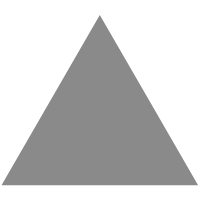
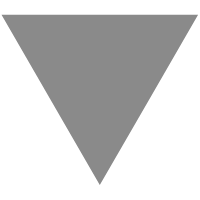
Unity实时反射相关优化
source link: https://blog.uwa4d.com/archives/USparkle_Reflection.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
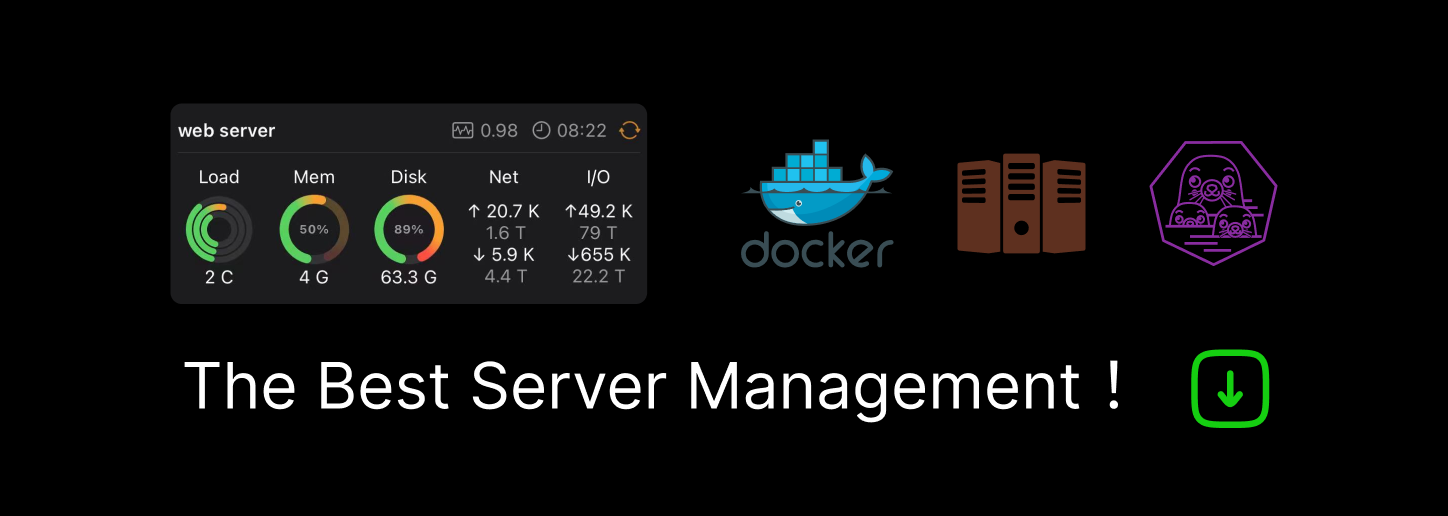
一.优化最终效果及消耗
8人同屏,最高画质,耗时1.1ms(高通 骁龙710)。因为项目未上线原因,只能用测试图进行相关说明。
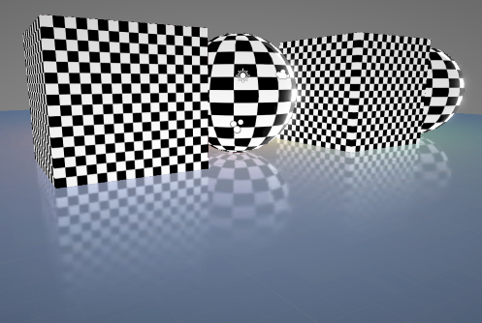
二、原反射脚本源码
1. 源码
using UnityEngine;
using System.Collections;
[ExecuteInEditMode]
public class MirrorReflection : MonoBehaviour
{
public Material m_matCopyDepth;
public bool m_DisablePixelLights = true;
public int m_TextureSize = 256;
public float m_ClipPlaneOffset = 0.07f;
public LayerMask m_ReflectLayers = -1;
private Hashtable m_ReflectionCameras = new Hashtable(); // Camera -> Camera table
public RenderTexture m_ReflectionTexture = null;
public RenderTexture m_ReflectionDepthTexture = null;
private int m_OldReflectionTextureSize = 0;
private static bool s_InsideRendering = false;
public void OnWillRenderObject()
{
if (!enabled || !GetComponent<Renderer>() || !GetComponent<Renderer>().sharedMaterial || !GetComponent<Renderer>().enabled)
return;
Camera cam = Camera.current;
if (!cam)
return;
// Safeguard from recursive reflections.
if (s_InsideRendering)
return;
s_InsideRendering = true;
Camera reflectionCamera;
CreateMirrorObjects(cam, out reflectionCamera);
// find out the reflection plane: position and normal in world space
Vector3 pos = transform.position;
Vector3 normal = transform.up;
// Optionally disable pixel lights for reflection
int oldPixelLightCount = QualitySettings.pixelLightCount;
if (m_DisablePixelLights)
QualitySettings.pixelLightCount = 0;
UpdateCameraModes(cam, reflectionCamera);
// Render reflection
// Reflect camera around reflection plane
float d = -Vector3.Dot(normal, pos) - m_ClipPlaneOffset;
Vector4 reflectionPlane = new Vector4(normal.x, normal.y, normal.z, d);
Matrix4x4 reflection = Matrix4x4.zero;
CalculateReflectionMatrix(ref reflection, reflectionPlane);
Vector3 oldpos = cam.transform.position;
Vector3 newpos = reflection.MultiplyPoint(oldpos);
reflectionCamera.worldToCameraMatrix = cam.worldToCameraMatrix * reflection;
// Setup oblique projection matrix so that near plane is our reflection
// plane. This way we clip everything below/above it for free.
Vector4 clipPlane = CameraSpacePlane(reflectionCamera, pos, normal, 1.0f);
Matrix4x4 projection = cam.projectionMatrix;
CalculateObliqueMatrix(ref projection, clipPlane);
reflectionCamera.projectionMatrix = projection;
reflectionCamera.cullingMask = ~(1 << 4) & m_ReflectLayers.value; // never render water layer
reflectionCamera.targetTexture = m_ReflectionTexture;
//GL.SetRevertBackfacing(true);
GL.invertCulling = true;
reflectionCamera.transform.position = newpos;
Vector3 euler = cam.transform.eulerAngles;
reflectionCamera.transform.eulerAngles = new Vector3(0, euler.y, euler.z);
reflectionCamera.depthTextureMode = DepthTextureMode.Depth;
reflectionCamera.Render();
// copy depth
Graphics.SetRenderTarget(m_ReflectionDepthTexture);
m_matCopyDepth.SetPass(0);
DrawFullscreenQuad();
Graphics.SetRenderTarget(null);
// Graphics.Blit(m_ReflectionTexture, m_ReflectionDepthTexture, m_matCopyDepth);
reflectionCamera.transform.position = oldpos;
//GL.SetRevertBackfacing(false);
GL.invertCulling = false;
Material[] materials = GetComponent<Renderer>().sharedMaterials;
foreach (Material mat in materials)
{
mat.SetTexture("_ReflectionTex", m_ReflectionTexture);
mat.SetTexture("_ReflectionDepthTex", m_ReflectionDepthTexture);
}
// // Set matrix on the shader that transforms UVs from object space into screen
// // space. We want to just project reflection texture on screen.
// Matrix4x4 scaleOffset = Matrix4x4.TRS(
// new Vector3(0.5f, 0.5f, 0.5f), Quaternion.identity, new Vector3(0.5f, 0.5f, 0.5f));
// Vector3 scale = transform.lossyScale;
// Matrix4x4 mtx = transform.localToWorldMatrix * Matrix4x4.Scale(new Vector3(1.0f / scale.x, 1.0f / scale.y, 1.0f / scale.z));
// mtx = scaleOffset * cam.projectionMatrix * cam.worldToCameraMatrix * mtx;
// foreach (Material mat in materials)
// {
// mat.SetMatrix("_ProjMatrix", mtx);
// }
// Restore pixel light count
if (m_DisablePixelLights)
QualitySettings.pixelLightCount = oldPixelLightCount;
s_InsideRendering = false;
}
// Cleanup all the objects we possibly have created
void OnDisable()
{
if (m_ReflectionTexture)
{
DestroyImmediate(m_ReflectionTexture);
m_ReflectionTexture = null;
}
if (m_ReflectionDepthTexture)
{
DestroyImmediate(m_ReflectionDepthTexture);
m_ReflectionDepthTexture = null;
}
foreach (DictionaryEntry kvp in m_ReflectionCameras)
DestroyImmediate(((Camera)kvp.Value).gameObject);
m_ReflectionCameras.Clear();
}
private void UpdateCameraModes(Camera src, Camera dest)
{
if (dest == null)
return;
// set camera to clear the same way as current camera
dest.clearFlags = src.clearFlags;
dest.backgroundColor = src.backgroundColor;
if (src.clearFlags == CameraClearFlags.Skybox)
{
Skybox sky = src.GetComponent(typeof(Skybox)) as Skybox;
Skybox mysky = dest.GetComponent(typeof(Skybox)) as Skybox;
if (!sky || !sky.material)
{
mysky.enabled = false;
}
else
{
mysky.enabled = true;
mysky.material = sky.material;
}
}
// update other values to match current camera.
// even if we are supplying custom camera&projection matrices,
// some of values are used elsewhere (e.g. skybox uses far plane)
dest.farClipPlane = src.farClipPlane;
dest.nearClipPlane = src.nearClipPlane;
dest.orthographic = src.orthographic;
dest.fieldOfView = src.fieldOfView;
dest.aspect = src.aspect;
dest.orthographicSize = src.orthographicSize;
}
// On-demand create any objects we need
private void CreateMirrorObjects(Camera currentCamera, out Camera reflectionCamera)
{
reflectionCamera = null;
// Reflection render texture
if (!m_ReflectionTexture || m_OldReflectionTextureSize != m_TextureSize)
{
if (m_ReflectionTexture)
DestroyImmediate(m_ReflectionTexture);
m_ReflectionTexture = new RenderTexture(m_TextureSize, m_TextureSize, 16);
m_ReflectionTexture.name = "__MirrorReflection" + GetInstanceID();
m_ReflectionTexture.isPowerOfTwo = true;
m_ReflectionTexture.hideFlags = HideFlags.DontSave;
m_ReflectionTexture.filterMode = FilterMode.Bilinear;
if (m_ReflectionDepthTexture)
DestroyImmediate(m_ReflectionDepthTexture);
m_ReflectionDepthTexture = new RenderTexture(m_TextureSize, m_TextureSize, 0, RenderTextureFormat.RHalf);
// m_ReflectionDepthTexture = new RenderTexture(m_TextureSize, m_TextureSize, 0, RenderTextureFormat.R8);
m_ReflectionDepthTexture.name = "__MirrorReflectionDepth" + GetInstanceID();
m_ReflectionDepthTexture.isPowerOfTwo = true;
m_ReflectionDepthTexture.hideFlags = HideFlags.DontSave;
m_ReflectionDepthTexture.filterMode = FilterMode.Bilinear;
m_OldReflectionTextureSize = m_TextureSize;
}
// Camera for reflection
reflectionCamera = m_ReflectionCameras[currentCamera] as Camera;
if (!reflectionCamera) // catch both not-in-dictionary and in-dictionary-but-deleted-GO
{
GameObject go = new GameObject("Mirror Refl Camera id" + GetInstanceID() + " for " + currentCamera.GetInstanceID(), typeof(Camera), typeof(Skybox));
reflectionCamera = go.GetComponent<Camera>();
reflectionCamera.enabled = false;
reflectionCamera.transform.position = transform.position;
reflectionCamera.transform.rotation = transform.rotation;
reflectionCamera.gameObject.AddComponent<FlareLayer>();
go.hideFlags = HideFlags.HideAndDontSave;
m_ReflectionCameras[currentCamera] = reflectionCamera;
}
}
// Extended sign: returns -1, 0 or 1 based on sign of a
private static float sgn(float a)
{
if (a > 0.0f) return 1.0f;
if (a < 0.0f) return -1.0f;
return 0.0f;
}
// Given position/normal of the plane, calculates plane in camera space.
private Vector4 CameraSpacePlane(Camera cam, Vector3 pos, Vector3 normal, float sideSign)
{
Vector3 offsetPos = pos + normal * m_ClipPlaneOffset;
Matrix4x4 m = cam.worldToCameraMatrix;
Vector3 cpos = m.MultiplyPoint(offsetPos);
Vector3 cnormal = m.MultiplyVector(normal).normalized * sideSign;
return new Vector4(cnormal.x, cnormal.y, cnormal.z, -Vector3.Dot(cpos, cnormal));
}
// Adjusts the given projection matrix so that near plane is the given clipPlane
// clipPlane is given in camera space. See article in Game Programming Gems 5 and
// http://aras-p.info/texts/obliqueortho.html
private static void CalculateObliqueMatrix(ref Matrix4x4 projection, Vector4 clipPlane)
{
Vector4 q = projection.inverse * new Vector4(
sgn(clipPlane.x),
sgn(clipPlane.y),
1.0f,
1.0f
);
Vector4 c = clipPlane * (2.0F / (Vector4.Dot(clipPlane, q)));
// third row = clip plane - fourth row
projection[2] = c.x - projection[3];
projection[6] = c.y - projection[7];
projection[10] = c.z - projection[11];
projection[14] = c.w - projection[15];
}
// Calculates reflection matrix around the given plane
private static void CalculateReflectionMatrix(ref Matrix4x4 reflectionMat, Vector4 plane)
{
reflectionMat.m00 = (1F - 2F * plane[0] * plane[0]);
reflectionMat.m01 = (-2F * plane[0] * plane[1]);
reflectionMat.m02 = (-2F * plane[0] * plane[2]);
reflectionMat.m03 = (-2F * plane[3] * plane[0]);
reflectionMat.m10 = (-2F * plane[1] * plane[0]);
reflectionMat.m11 = (1F - 2F * plane[1] * plane[1]);
reflectionMat.m12 = (-2F * plane[1] * plane[2]);
reflectionMat.m13 = (-2F * plane[3] * plane[1]);
reflectionMat.m20 = (-2F * plane[2] * plane[0]);
reflectionMat.m21 = (-2F * plane[2] * plane[1]);
reflectionMat.m22 = (1F - 2F * plane[2] * plane[2]);
reflectionMat.m23 = (-2F * plane[3] * plane[2]);
reflectionMat.m30 = 0F;
reflectionMat.m31 = 0F;
reflectionMat.m32 = 0F;
reflectionMat.m33 = 1F;
}
static public void DrawFullscreenQuad(float z = 1.0f)
{
GL.Begin(GL.QUADS);
GL.Vertex3(-1.0f, -1.0f, z);
GL.Vertex3(1.0f, -1.0f, z);
GL.Vertex3(1.0f, 1.0f, z);
GL.Vertex3(-1.0f, 1.0f, z);
GL.Vertex3(-1.0f, 1.0f, z);
GL.Vertex3(1.0f, 1.0f, z);
GL.Vertex3(1.0f, -1.0f, z);
GL.Vertex3(-1.0f, -1.0f, z);
GL.End();
}
}
2. 该脚本主要功能
在场景中获取当前生效的相机,根据反射平面进行镜像,并对设定的渲染层进行逐帧拍照,并将其传给反射平面进行显示。配合自带的半透明反射材质效果,叠加到底层材质上使用。
3. 主要问题
优化之前,该反射效果大概耗时10ms以上。主要问题如下:
反射效果均为实时反射,使用Layer控制。要看到反射效果,只能将该层物体纳入反射层。
反射表面为独立于真实反射表面的半透明材质,既耗性能效果也无法保证。
项目角色的面数极高(单角色万面左右),极限同屏9个角色外加宠物。除了本身的角色渲染外,同时还存在描边、压片阴影等效果。如果反射直接采用相机拍照的方式生成,额外的性能消耗极大。
项目角色本身渲染支持5盏像素光,带法线贴图,卡通光照图等各种复杂渲染效果,如果反射直接采用相机拍照的方式生成,额外的性能消耗极大。
该脚本本身也存在一些问题:
1)会根据场景中正在生效的相机,逐个生成反射相机。
2)逐帧获取反射材质,进行参数传递。
3)反射层的设置需要美术每次使用时都设置一次,容易设置过多或漏设置。
三、优化方向
针对以上问题,实时反射主要有两大优化方向,美术效果优化及性能优化。
一、美术效果优化
1.将反射效果与反射表面物理属性关联。
引入反射表面法线贴图的支持,对反射效果增加真实的法线扭曲效果。
方法:弃置默认的半透明反射表面。将反射图直接指定给反射表面的渲染材质,增加法线效果,使用法线贴图的xy通道对反射图的UV进行偏移(这里还增加了法线扭曲强度及遮罩等的调节)。
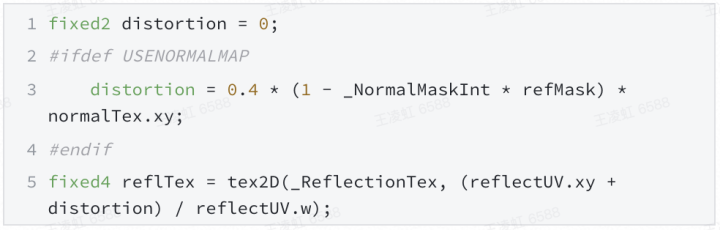
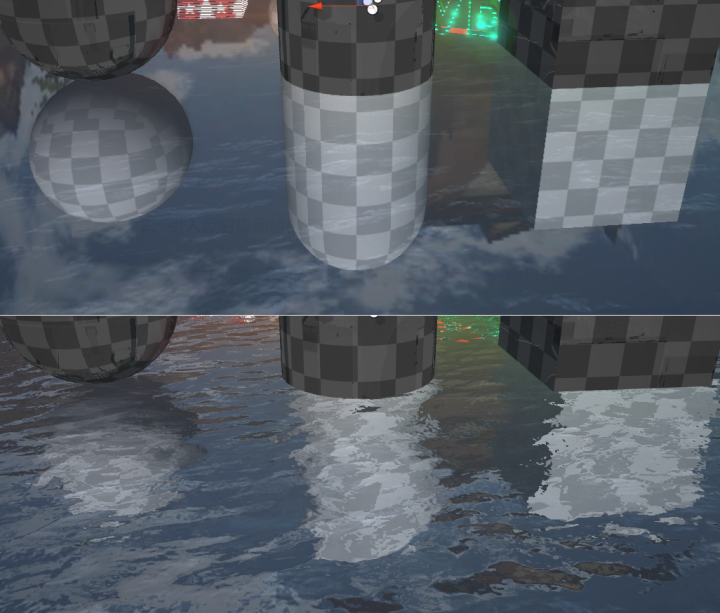
2.引入反射表面粗糙度设置。
可以调节反射效果的清晰度(模糊策略,后面性能优化详述)。
方法:不考虑性能,这里可以向脚本中传参,使用高斯模糊来进行粗糙表面的反射模糊效果。
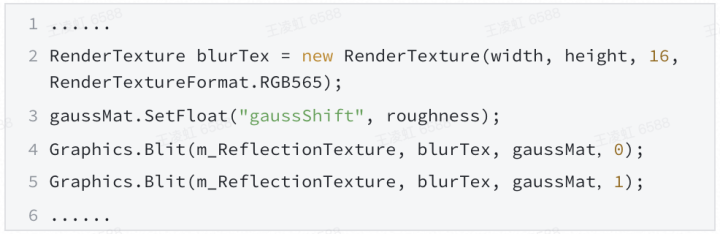
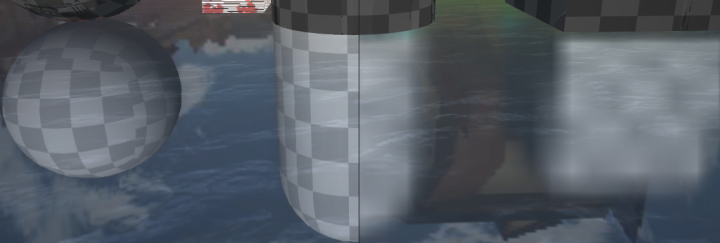
3.引入反射深度,实现反射跟随距离从有到无的柔和过渡。
方法:在渲染反射图时,传入衰减系数,根据顶点到地面高度的距离进行衰减,衰减强度写入反射图的A通道。


二、性能优化
概述:
性能优化方面主要集中在如下几个方面:
- 减少数据量,降低CPU负担。
- 降低DrawCall,增加C/GPU传输率。
- 降低渲染复杂度,加速GPU渲染。
- 优化脚本,减少GC及重复操作。
基于以上考虑,性能优化方向归为两类:美术端优化和程序端优化。
美术端优化
1.动静分离
将反射效果拆分为静态物体的与烘焙反射和动态物体的实时反射两部分组成。这样可以大大降低实时运算量。
2.预烘焙部分
根据场景需要的反射清晰度,预先烘焙尺寸尽可能小的反射图。对于非常粗糙且并不清晰的反射场景,可以共用一张场景反射图。
或者昼夜、雨晴等同场景不同状态下的反射图共用。通过调节材质参数(例如反射颜色、粗糙度及反射强度)来实现不同状态效果。
通过BoxProjection方法,修正与烘焙CubeMap的场景对齐问题。
3.实时部分
同静态与烘焙相似,可以根据场景的反射清晰度,实时生成尽可能小的反射图来使用。
(1)程序开放尺寸调节:

(2)代码写入支持尺寸配置:
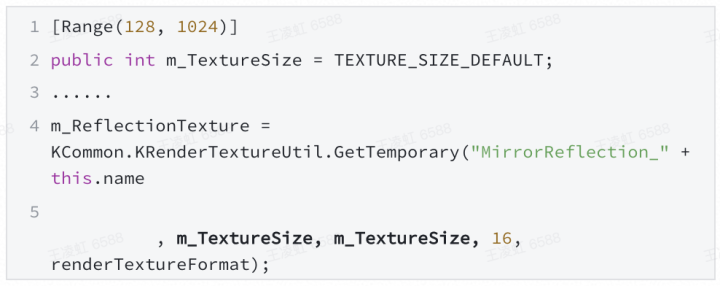
降低实时反射的DrawCall,例如:尽可能地合并角色部件,忽略较小部件不进行反射等。
降低实时反射的数据量和计算量,例如:使用低面数的Lod来渲染反射,降低反射效果要求减少灯光数量,效果数量等。
- 反射表面优化策略
同一个场景,只能存在一个反射表面。
将反射表面的材质作为高配材质由美术特别对待。
将尽可能多的参数暴露给美术,尽可能地适配高性能参数。
程序端优化
同样从概述中的几个方面进行考虑来进行优化。
1.去除不必要的渲染批次及效果
反射效果中因为清晰度有限,描边效果可以剔除,阴影效果也可以不进行渲染。
2.降低反射渲染的计算复杂度
反射效果中,只需要表现出基本的明暗关系即可,故只保留1盏平行光效果。光照计算也从像素光照更改为顶点光照。
一个角色渲染需要基本色纹理,法线纹理,粗糙金属性纹理,身体材质区分纹理,光照纹理和buff效果纹理。在反射中,精简效果到只需要基本色纹理和buff效果纹理即可。
要实现以上优化方案,就需要在进行反射渲染时,将动态物体的材质进行替换渲染。可用策略如下:
(1)更换材质
需要每帧用反射材质进行替换渲染。如果是后处理描边、风格化单色等效果,这类方法比较适合。但角色材质多样,参数各不相同,用这种方法需要自己维护动态物件的反射列表,比较繁琐。
(2)Lod Int控制
就是使用SubShader的Lod参数进行控制。这样,把各个材质的反射Shader集中到一个Lod下进行计算即可。最简单的一种方式,但后来经过测试,实时切换Lod的消耗过大,不太实际。
(3)宏开关
比较简单,集成性、通用性好,且切换消耗小。最初我们使用的优化方案。但因为存在角色的多材质及多Pass渲染,后期对优化效率仍然不足够(无法优化掉描边和阴影)。
核心代码:
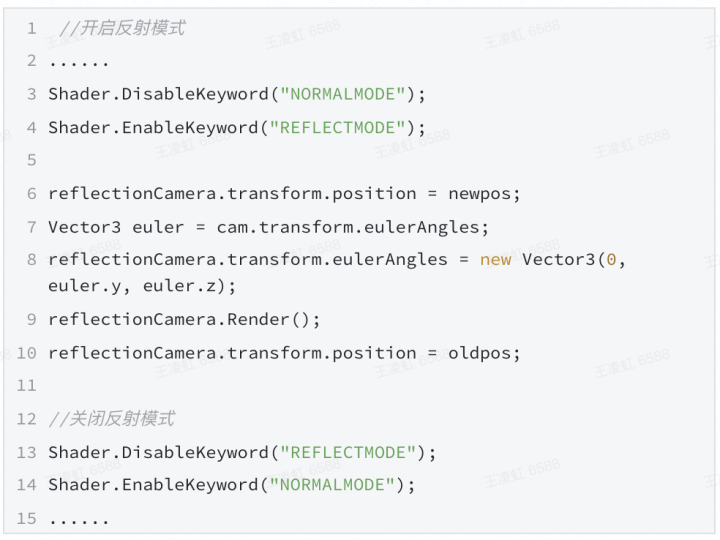
(4)使用CommandBuff
可控性和自由度最高。但问题同(1),需要自己维护角色列表,对于频繁发生角色更换的场合,维护成本会较高,但仍可实现。可以由逻辑层在发生角色更换时,更新角色列表到反射程序中进行处理即可。
部分核心代码:
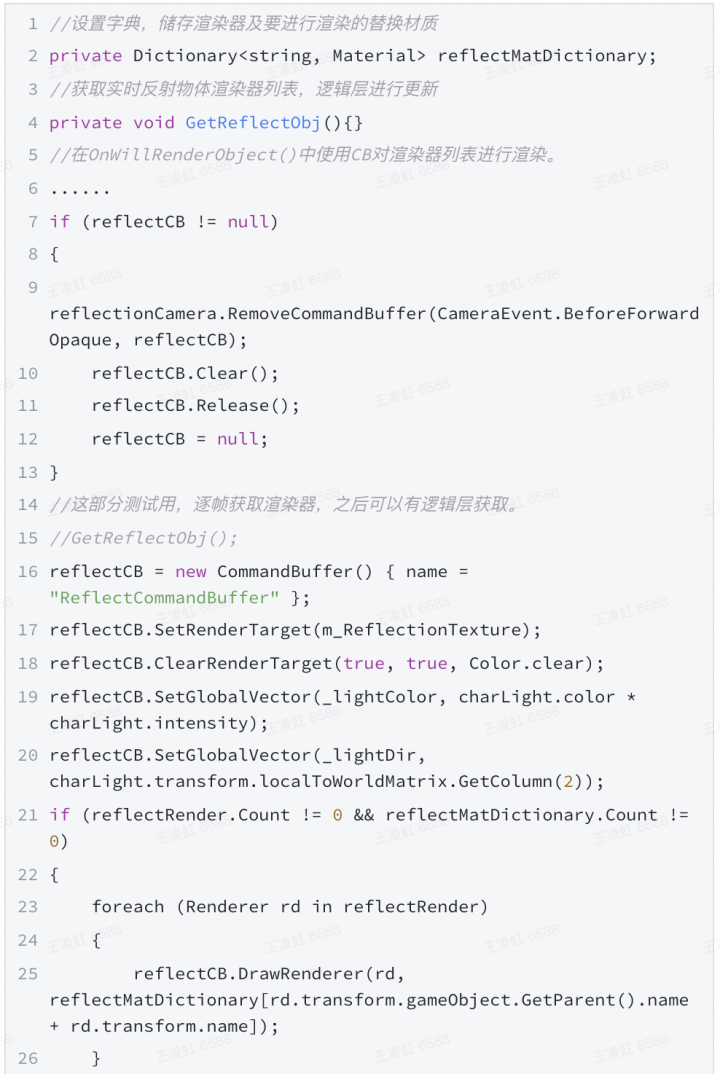
(5)使用CameraReplaceShader
我们项目最终优化采用的方法。优点是可以实现替换渲染,且不需要自己维护角色列表。缺点就是重写反射渲染的Shader。
核心脚本代码:
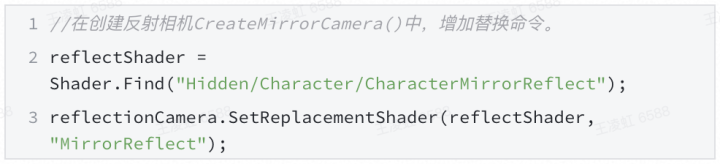
这里简单解释一下SetReplacementShader命令。

他是将该相机中进行渲染的所有Shader,根据后面设定的replacementTag进行替换。
例如上面代码中,我们使用了“MirrorReflect”的Tag,所以,在Shader中,凡是含有“MirrorReflect”Tag的SubShader都会被替换为reflectShader中,相同“MirrorReflect”Tag的SubShader。
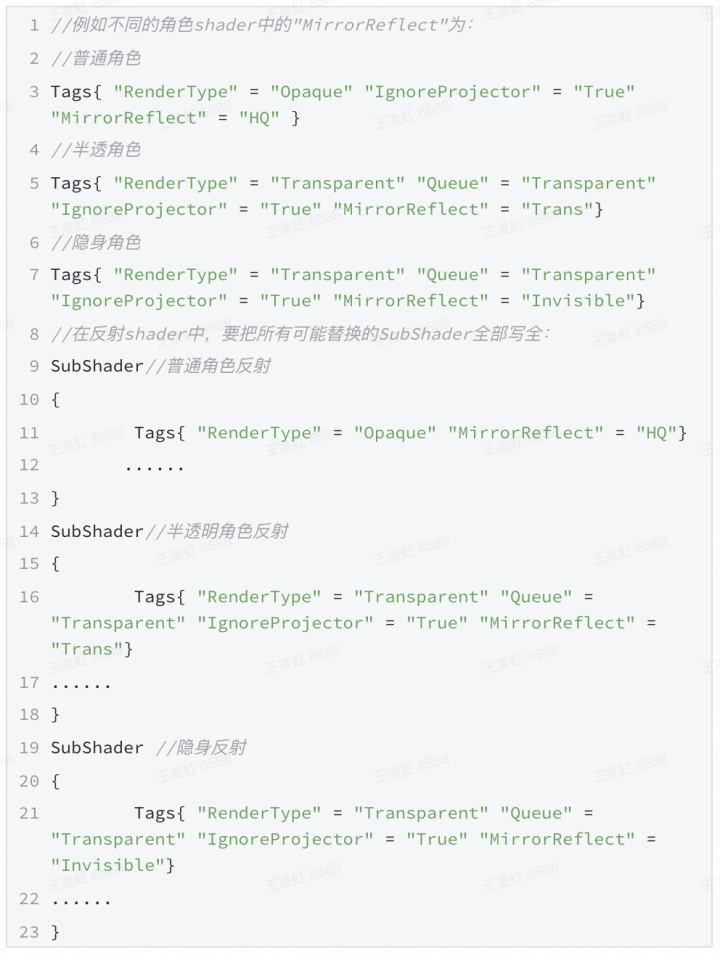
如上举例,即可在相机进行反射渲染时,将含有相同“MirrorReflect”Tag的SubShader 进行对应替换渲染了。
程序策略性优化
1.合并静态与实时反射
只需要根据反射图的A通道中,存储的反射衰减值进行动静反射混合即可。
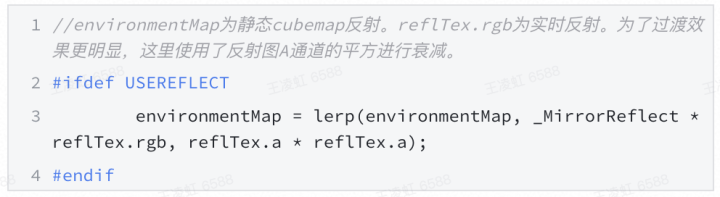
2.开放美术调节参数
如前所述的一些性能相关设置,可以开放给美术进行择优。
3.反射清晰度
引入粗糙度的概念,使用模糊来遮盖反射图分辨率不足的问题。可以进一步降低反射分辨率。
这里的模糊策略采用了MipMap的方式来避免高斯模糊带来的消耗。


程序逻辑层性能优化
1.减反射相机数量
将反射相机个数从逐相机生成的多反射相机优化为单反射相机,逐帧对位的方式。
2.减GC
将申请RT,生成相机,获取反射材质,传递材质参数等可一次性进行的操作,移出OnWillRenderObject()。
3.优化刷新率
增加刷新率设置,将逐帧渲染改为可配置刷新率渲染。目前默认为1/2刷新率。
4.反射层程序端写死
避免美术出错及加速配置。
5.开关策略
当反射平面不可见时,关闭反射功能,将所有资源进行释放(逻辑层控制)。
6.平台匹配
适配不同性能的平台。目前我们仅高配平台开启实时反射效果。中配置平台仅开启静态反射效果。
最后:优化总结
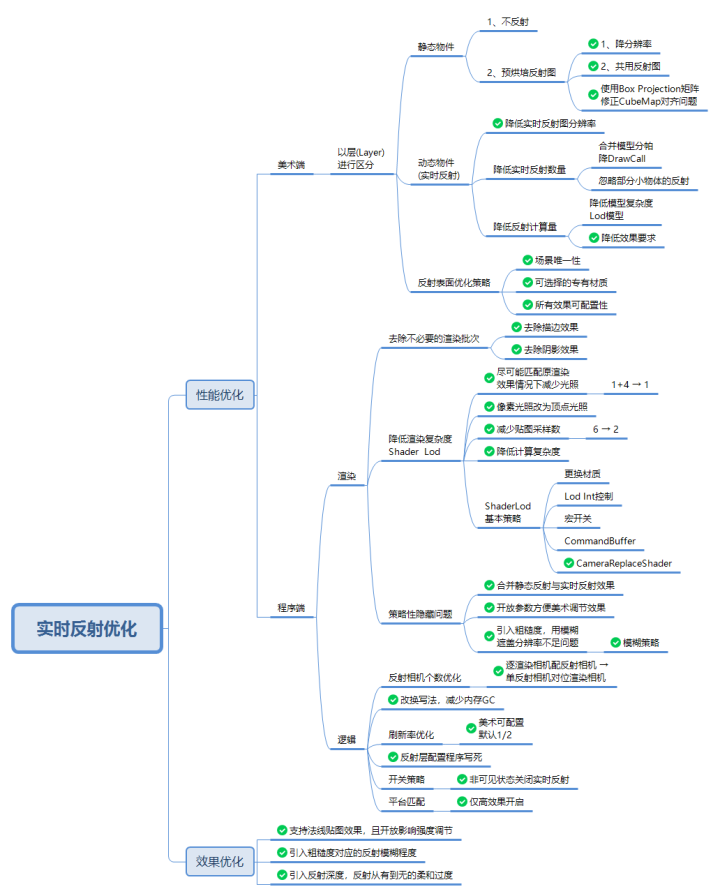
这是侑虎科技第1072篇文章,感谢作者罗汉铭供稿。罗汉铭:朝夕光年无双工作室 技术美术专家,目前就职于字节跳动101技术中心,08年加入上海昱泉国际,从事TA相关工作,参与了《流星蝴蝶剑》、《射雕三部曲》项目的制作。之后加入完美世界引擎组,从事ARK1.0-ARK2.0的相关开发工作,并支持《最终兵器》、《射雕英雄传OL》项目的开发;后转向Unity,参与了《射雕英雄传3D》手游的开发工作。
作者主页:https://www.zhihu.com/people/lucifer-3-91
作者在 UWA DAY 2021 大会中的《航母战斗群中的巡洋舰 - 为项目保驾护航的技术美术们》议题演讲已经上线UWA学堂,课程限时优惠中,扫码即可前往观看。

再次感谢罗汉铭的分享,如果您有任何独到的见解或者发现也欢迎联系我们,一起探讨。(QQ群:793972859)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK