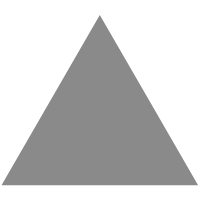
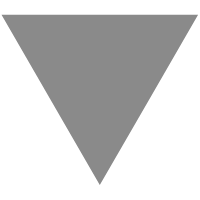
Modular Feature Driven Development - The Smart Phone Pattern (OS) with React &am...
source link: https://orizens.com/blog/modular-feature-driven-development-the-smart-phone-pattern-os-with-react-redux/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
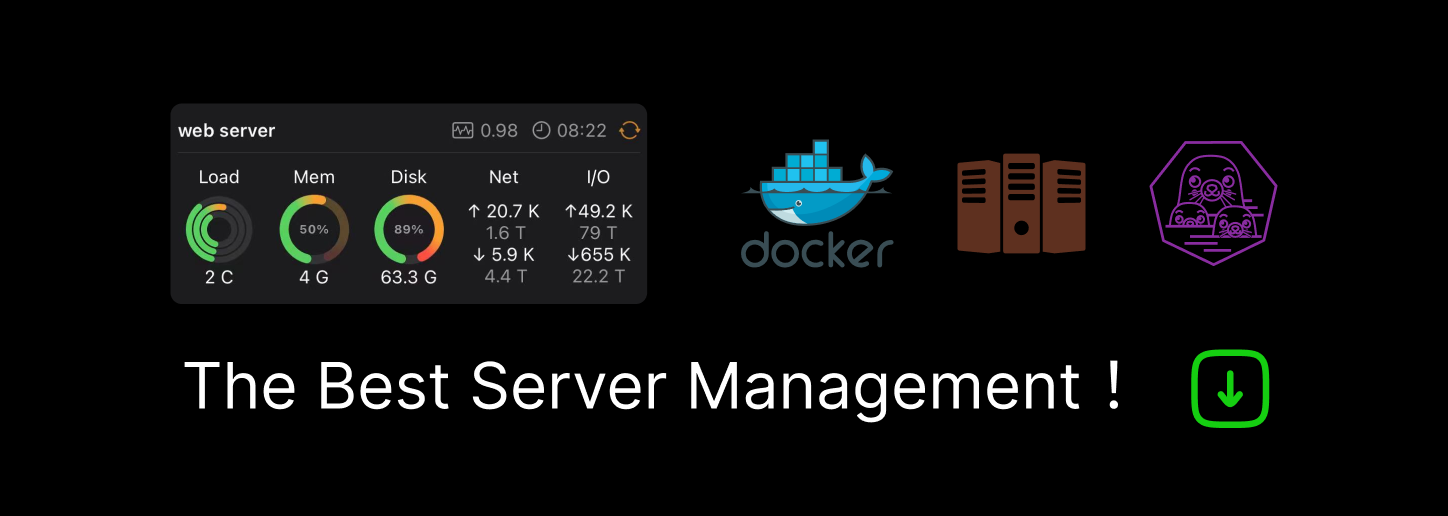
Modular Feature Driven Development - The Smart Phone Pattern (OS) with React & Redux
February 24, 2022

Modular Feature Driven Development - The Smart Phone Pattern (OS) with React & Redux
Front end architecture is a very important aspect I consider when developing apps. I’ve been developing front end since around 2007 - and so - since then - i’ve been interested in software architecture concepts, design patterns and code organization. Once, in a code review session, I was trying to explain a certain strategy i would have liked the code would be, then a colleague told me -
Not everyone thinks the same you do. that’s hard to understand.
He was right. I was trying to explain what to right and was going through all the necessary parts, but i missed one thing: Showing the big picture.
This article is focusing on an approach i’m taking when designing a feature - only then, i can refer to directory structure and follow what makes sense.
My goal is to share a perspective, show a way of thinking, a Style Guide (if I may), that I follow - while I think it may help others as well.
Feature Driven Development from wikipedia,
Feature-driven development (FDD) is an iterative and incremental software development process
The Big Picture
I was once told it’s easier if you show us rather than telling us. There are many good articles recommending about a certain directory structure, where to have what and how to divide the code files into parts.
Smart phones and mobile devices have been around for quite some time now. When i’m coding or start to design a feature, I consider a Smart Phone as the Mental Model for the app.
To simply put, when I need to add a feature to an existing app, i’m instantly thinking where it would have been belong if it was a smart phone feature that was built into the code operating system.
That’s it in a nutshell - that’s how I explain the Smart Phone Pattern (SPP) - but there’s more to it.
Rules of the Smart Phone Pattern
I a smart phone, most of these basic features are includes with the device’s api - camera, user, contacts, geolocation, microphone, sound etc. Usually, when an app is installed on the device, it asks the user to approve access for few of these api’s - usually the users just confirms. Meaning, the app relies on the device’s api and the user’s approval to access those api’s,
This is the perspective that I look at when adding features to an app. Coming into the web front end development world, most chances the basic api’s would be included within these layers:
- containers/pages layer - components that reflects a certain view that is bound to a url usually
- state management - some form of state layer (redux, react-query etc..)
- components
- hooks (React)
- utilities
- API layer
- modules/features (the goal of this article)
I consider items 2-6 as the building blocks of an app - it’s the base framework in which the entire app relies on. Once these exist, any feature that consume any of these, would probably be consumes in item #1 - containers/pages.
Features/Modules take the role of an app in a smart phone. lets see how this perspective translates in a react app.
Feature Driven Development
First, I usually, keep new features in the modules/features folder (whatever works best for the team). A feature can expose components, hooks or utilities - think an npm package that access directly to one of the layers I mentioned before. By using and importing a certain building block of one of the layers, the app ‘gives’ permission to the feature (the smart phone app) to use these.
A feature can do whatever permissions it is granted. Usually like to bind a feature around a store’s slice. That means:
- Updating the app state (phone settings, user’s data etc..)
- Share state with other features (app within an app)
- Link to other views within the app (in smart phone - open other apps, open browser etc..)
A good example would be a playlist component of a music app:
<Player>
<MediaView />
<Controls />
<Playlist />
</Player>
There are few principals to notice about the Playlist
module/feature:
- It doesn’t take any props - although it might for setting features on/off within the playlist component
- It has permissions to use these layers: state, api, components
- We understand this feature is consumed by the
Playlist
component
Similarly to an app on the smart phone, a feature may expose more ways to interact with its data, i.e:
- Exposing a
<CurrentlyPlaying />
module (widgets in android) - Exposing a hook to interact with the feature
usePlaylistController()
Folder & Files structure
A feature lives within the modules/features
folder. As I mentioned before, it asks for permission to use any of the existing building blocks that exists in the app.
If the feature requires to a state layer (In Redux), the store
folder that includes all the state definitions and clean store api hooks.
- src
- modules
- Playlist
- index.ts
- Playlist
- Playlist.tsx
- CurrentlyPlaying
- CurrentlyPlaying.tsx
- hooks
- index.ts
- usePlaylistController.ts
- useCurrentlyPlaying.ts
- store
- index.ts
- playlist.slice.ts
Notice the Playlist/index.ts
- this is the entry point of the module. This file includes all the relevant export
statements this module is exposing for the app to consume. i.e:
export * from "./Playlist/Playlist"
export * from "./CurrentlyPlaying/CurrentlyPlaying"
export * from "./hooks"
export * from "./store"
I like this approach, because eventually, I would like to consume these like that:
import { Playlist, CurrentlyPlaying } from "modules/Playlist"
Organizing features with this strategy is useful for a few reasons:
- isolated and incremental development
- feature flag ready
- easily testable
- low risk in touching any of the existing app’s code
- plug and play within the app
During phases of development, a feature may introduce new components or hooks that expose a different kind of interaction or visual elements. Usually, when there’s a requirement to use or view information from the feature’s store, I tend to create either a new component inside the feature’s folder. Doing that, I know any aspect of that features state is encapsulated and saved inside this feature’s folders. It becomes intuitive to search and skim through when searching for anything related to that feature.
What is the limit of this smart phone modular development?
Usually, a feature’s component would be consumed in a container/page component. Like any other strategy, there are always exceptions. For me, when a new feature is state agnostic and doesn’t have to rely on a state layer, it would directly go into the components
folder.
A feature may not include its own store
folder, but rather use existing store slices.
I think feature driven development should play a main role in the software development cycle - being able to design and implement modular features contributes to an app’s architecture, stability and testability.
Experience modular driven development approach in ReadM - a free virtual English reading coach app - please try it out.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK