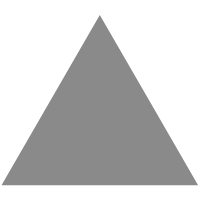
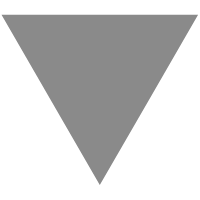
Android的XML常用标签整理
source link: https://segmentfault.com/a/1190000041473391
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
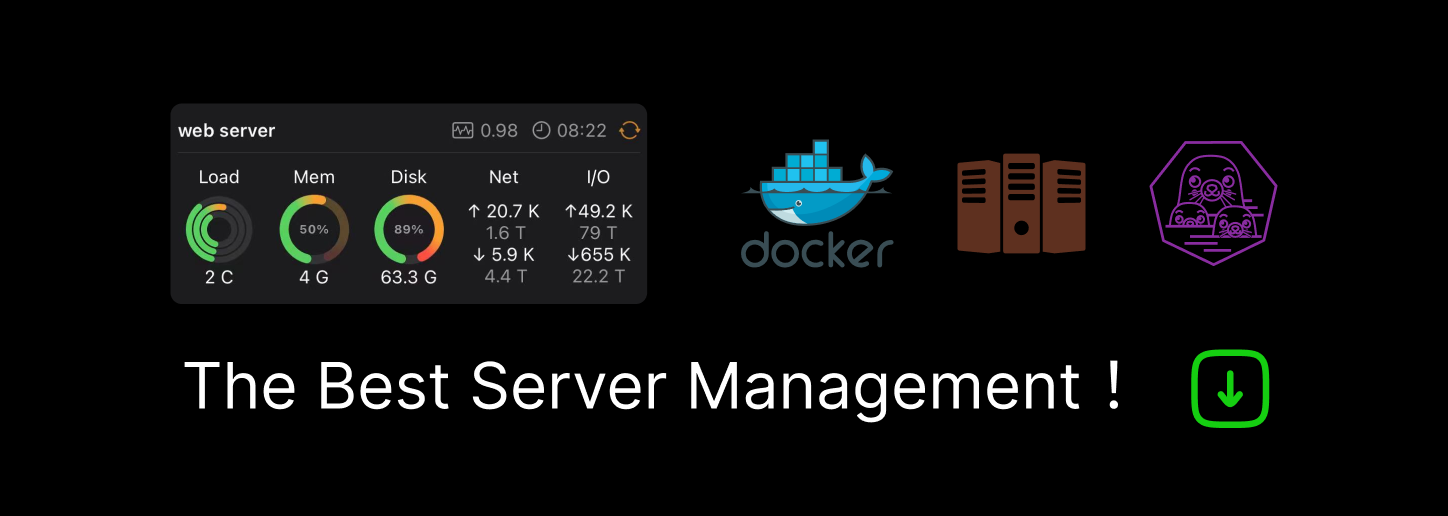
一、XML和HTML的异同
1、常规标签和自闭合标签
XML和HTML有许多相似之处,XML的标签分为两类。
一类是成对的标签,例如<manifest>和</manifest>
另一类是自闭合标签,因为这一类标签的里面没有内容,所以它只需要一个标签就能实现所有功能,例如
<activity android:name=".MainActivity2" android:exported="false" />
自闭合标签可以转换成常规的成对的标签,只需要去掉最后的/,再加上</name>即可。
2、标签内的属性
熟悉HTML的同学肯定知道HTML标签内部可以加CSS样式、定义class、定义ID以及使用标签所拥有的属性,而一对标签的中间显示的是内容。例如:
<p style="color: white" class="mt-4">努力加载中... </p>
但Android XML的用法有细微的区别,没有“标签中间的字符表示内容”这个功能。而是写在某个属性里。例如:
<Button android:text="Hello"/>
所以,在layout布局中,绝大多数标签都是自闭合标签。
二、常见Android页面元素的标签及属性
1、Android主配置文件AndroidManifest.xml
manifest有显示的意思,这个文件定义了包名、活动、启动的活动等等。例如:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.myapplication" > ① <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" ② android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.MyApplication" > ③ <activity ④ android:name=".MainActivity" ⑤ android:exported="true" > <intent-filter>⑥ <action android:name="android.intent.action.MAIN" /> ⑦ <category android:name="android.intent.category.LAUNCHER" /> </intent-filter>⑥ </activity> ④ </application> </manifest>
常见属性:
① manifest中的package定义了包名,包名就像其他语言中的命名空间,告诉计算机这个类的住址,JAVA根据包名+类名来确定唯一的类
② android:label属性声明软件的软件名,这个标题运行时会写在页面的顶部(如果页面没有定义标题的情况下)
③ android:theme属性声明的软件的主题,引入了另一个主题文件来设定样式,类似全局CSS文件
④ <activity>标签是定义活动的标签,所有的活动都要写在这里,因为标签内部有其他内容,分裂成常规的成对标签
⑤ android:name属性,指出这个活动的类名,这个类名和JAVA文件相对应。之所以有一个.是因为JAVA查找类的时候使用 “包名.类名”
⑥ <intent-filter>是意图选择器,意图用来完成活动之间的通信。意图选择器可以告诉Android系统,这个活动可以处理什么类型的意图
⑦ <action>是一个动作,android.intent.action.MAIN表名这个活动是主活动,也就是整个Android的入口
2、layout布局
一个空的,没有任何元素的活动,代码是这个样子的:
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout ① xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" ② android:layout_height="match_parent" tools:context=".MainActivity"> ③ </androidx.constraintlayout.widget.ConstraintLayout>
① <androidx.constraintlayout.widget.ConstraintLayout> 这个标签指的是活动的布局(Layout)
② android:layout_width属性用来定义此元素的宽度,layout_height用来定义高度。
如果按住Conmand键点进去就能看到这个属性允许设定的值:
遇事不决就翻译:
指定视图的基本宽度。这是包含布局管理器的任何视图所必需的属性。其值可以是恒定宽度的尺寸(如“12dip”)或特殊常数之一。
fill_parent: 视图应该和其父视图一样大(减去填充)。从API级别8开始,这个常量被弃用,并被{@code match_parent}替换
match_parent:视图应该和其父视图一样大(减去填充)。在API级别8中引入
wrap_content:视图的大小应仅足以包含其内容(加上填充)
③tools:context属性表名这个活动的类名。
3、layout的TextView文本框
这个标签的效果等于HTML的P标签。
<TextView ① android:id="@+id/textView2" ② android:layout_width="wrap_content" ③ android:layout_height="wrap_content" ③ android:layout_marginTop="172dp" ④ android:layout_marginEnd="264dp" ④ android:text="HelloWorld" ⑤ app:layout_constraintEnd_toEndOf="parent" app:layout_constraintTop_toTopOf="parent" />
① 指明这是一个TextView
② android:,ID很重要,Android根据ID定位唯一的XML元素,ID的值必须使用@+id/name
③ android:layout_width属性设定宽度,layout_height设定宽度,三个特殊值和前文提到的相同
④ android:layout_margin指定此视图顶部的额外空间。这个空间在这个视图的边界之外。
Android XML中,border、margin和CSS中的思想完全相同
⑤ android:text指定此XML元素内容的字符,相当于P标签中间的内容
4、layout的botton按钮
<Button ① android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginEnd="56dp" android:layout_marginBottom="121dp" android:text="Button" ② android:onClick="onClickFunction" ③ app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" />
① 指定这是一个按钮
② android:text指定按钮上的文字,相当于HTML中button中间的内容
③ android:onClick(重要)指定此按钮点击后,执行哪个方法,内容为本Activity中的方法名
5、layout中的spinner选择器
spinner相当于HTML中的select组件
<Spinner ① android:id="@+id/spinner" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginStart="172dp" android:layout_marginBottom="304dp" android:entries="@array/string_array" ② app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintStart_toStartOf="parent" />
① 指定此元素是一个spinner
② android:entries 指明此选择器的数据源,也就是下拉菜单的内容。内容来自string文件。
对于任何一门语言,熟悉基本写法的过程都是查文档、看源码和实践操作的结合。
对于不熟悉的标签,多看、多翻译源码里面的注释,可以获得不错的效果。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK