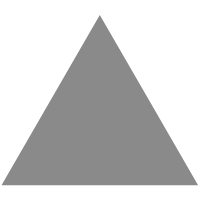
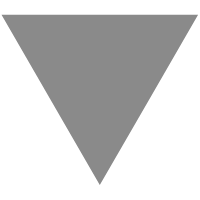
Why an array is an object in JavaScript?
source link: https://dev.to/fromaline/why-an-array-is-an-object-in-javascript-4bh6
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
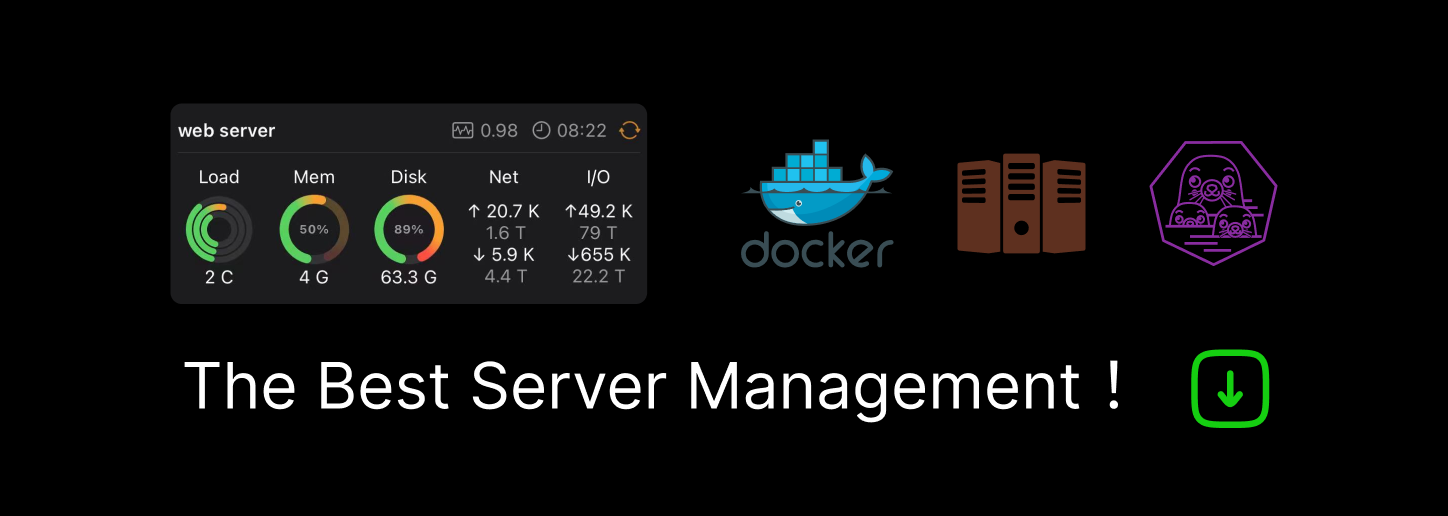
JS is a prototype-based language, so there are only primitive types and objects. It grants flexibility but makes things more confusing at the same time.
👉 Everything is an object!
Implementation of all non-primitive values in JavaScript is object-based.
Simply put, JavaScript has a single prototypical object from which all other objects get their initial properties. We can get it by accessing __proto__
.
Object.getPrototypeOf(Object).__proto__;
Object.getPrototypeOf(Array).__proto__;
Object.getPrototypeOf(Boolean).__proto__;
// The prototypical object of every object
{
constructor: ƒ Object()
hasOwnProperty: ƒ hasOwnProperty()
isPrototypeOf: ƒ isPrototypeOf()
propertyIsEnumerable: ƒ propertyIsEnumerable()
toLocaleString: ƒ toLocaleString()
toString: ƒ toString()
valueOf: ƒ valueOf()
__defineGetter__: ƒ __defineGetter__()
__defineSetter__: ƒ __defineSetter__()
__lookupGetter__: ƒ __lookupGetter__()
__lookupSetter__: ƒ __lookupSetter__()
__proto__: (...)
get __proto__: ƒ __proto__()
set __proto__: ƒ __proto__()
}
Enter fullscreen mode
Exit fullscreen mode
👉 Every array is an object too!
The array type is not an exception here. Array
global class is a global object and an array literal is just an instance of the Array
global class.
In turn, a direct prototype of the array type contains all its special methods, like fill, find, etc.
// true
Object.getPrototypeOf(Array).__proto__ === Object.getPrototypeOf(Object).__proto__
Object.getPrototypeOf([]).__proto__ === Object.getPrototypeOf(Object).__proto__
Object.getPrototypeOf([])
[
at: ƒ at()
concat: ƒ concat()
constructor: ƒ Array()
copyWithin: ƒ copyWithin()
entries: ƒ entries()
every: ƒ every()
fill: ƒ fill()
filter: ƒ filter()
find: ƒ find()
findIndex: ƒ findIndex()
findLast: ƒ findLast()
findLastIndex: ƒ findLastIndex()
flat: ƒ flat()
...
]
Enter fullscreen mode
Exit fullscreen mode
👉 How is it implemented in the JavaScript engine?
Similarly, arrays are a special case of objects inside the JavaScript engine.
But they have:
- special handling of indices
- a magical length property
To understand how objects work, check out my article.
👉 Indices handling
Array indices are represented as strings, that contain numbers.
So every element inside an array is associated with a numeric string.
👉 Length property
The length is just non-configurable and non-enumerable property. The JavaScript engine automatically updates its value once an element is added to the array or deleted from it.
P.S. Follow me on Twitter for more content like this!
How does React handle user-defined components behind the scenes?
React strives to give its users the ability to build encapsulated, reusable components, but how does it manage to implement this logic in JSX?
#webdevelopment #reactjs
Read thread to find out 👇18:41 PM - 24 Jan 2022
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK